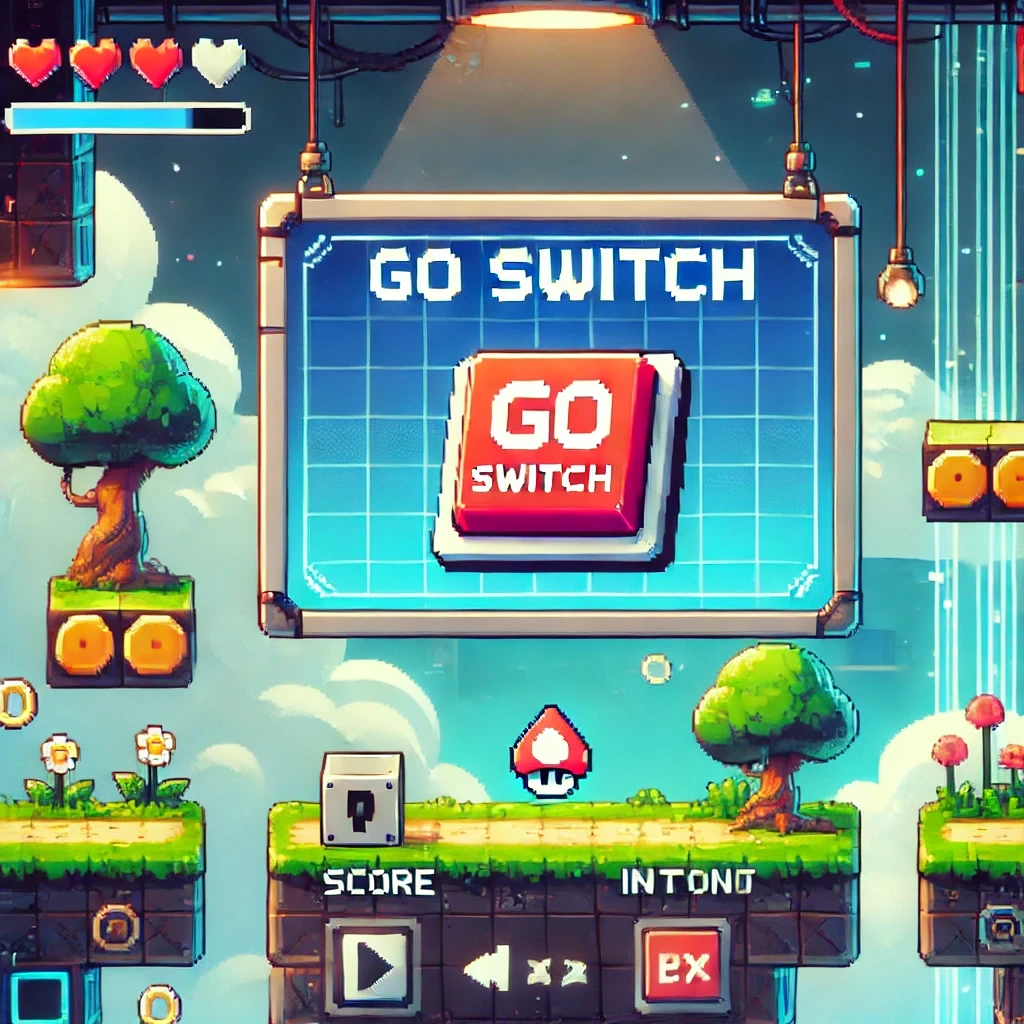
A Simple Guide to Switch Statements in Go
Do you ever get tired of writing multiple if-else statements in your Go code? Golang case statements (also called switch statements) can make your logic clearer, faster, and easier to maintain. In this post, you’ll learn how to use a switch statement in Go to simplify your code.
Why Use a Switch Statement in Go?
In Go, switch statements help you handle different possibilities without creating a chain of endless if-else blocks. A golang case is a direct way to check a condition and then execute just the code you need. This improves readability because:
- It makes your intention obvious.
- It groups related cases together.
- It lowers the chance of errors compared to a long list of if-else statements.
Basic Structure of a Golang Case
Here’s a simple example of a switch statement in Go:
Use a variable called value
and check it like this:
switch value {
case 1:
fmt.Println("Value is 1")
case 2:
fmt.Println("Value is 2")
default:
fmt.Println("Value is something else")
}
value
is the variable you want to check.case
lines show potential matches.default
runs if none of the other cases match.
Multiple Conditions in One Case
You can also put several conditions under one case. If you have related values that need the same treatment, group them:
switch day := "Tuesday"; day {
case "Monday", "Tuesday", "Wednesday":
fmt.Println("It's a weekday!")
default:
fmt.Println("Not a weekday.")
}
This makes your code shorter and more clear, instead of creating a separate case for each day.
The Blank Switch for Flexible Conditions
Go lets you create a “blank” switch, meaning you don’t have to specify a particular variable. Instead, you can use boolean expressions:
value := 95
switch {
case value > 90:
fmt.Println("Great job! You scored above 90.")
case value >= 70:
fmt.Println("Good work! You’re above 70.")
default:
fmt.Println("Keep trying!")
}
This is handy when you want to check a range of conditions, rather than comparing just one variable to different values.
Fallthrough: When You Need Multiple Matches
In Go, execution doesn’t move automatically from one case to the next. If you want to handle multiple cases in sequence, use fallthrough
:
switch number := 1; number {
case 1:
fmt.Println("One")
fallthrough
case 2:
fmt.Println("Two")
}
Without fallthrough
, Go would stop after matching the first case. Use it sparingly, though—it can make your code less obvious to other readers.
Final Thoughts
Using golang case statements is a great way to make your logic easier to read, keep related conditions together, and cut down on bugs. Whether you’re new to Go or just refreshing your skills, learning to use switch effectively is an important tool for writing clear, maintainable code.
Key Takeaways:
- Switch statements replace long if-else chains for easier reading.
- You can group multiple conditions in one case.
- A blank switch lets you use boolean expressions for more flexible checks.
- Fallthrough can process more than one case, but use it with caution.
Now that you know how to use golang case statements, try them out in your next Go project!
If you want to learn more even more Go, we have a free video course for you here. Complete it and get a free JetBrains GoLand License!