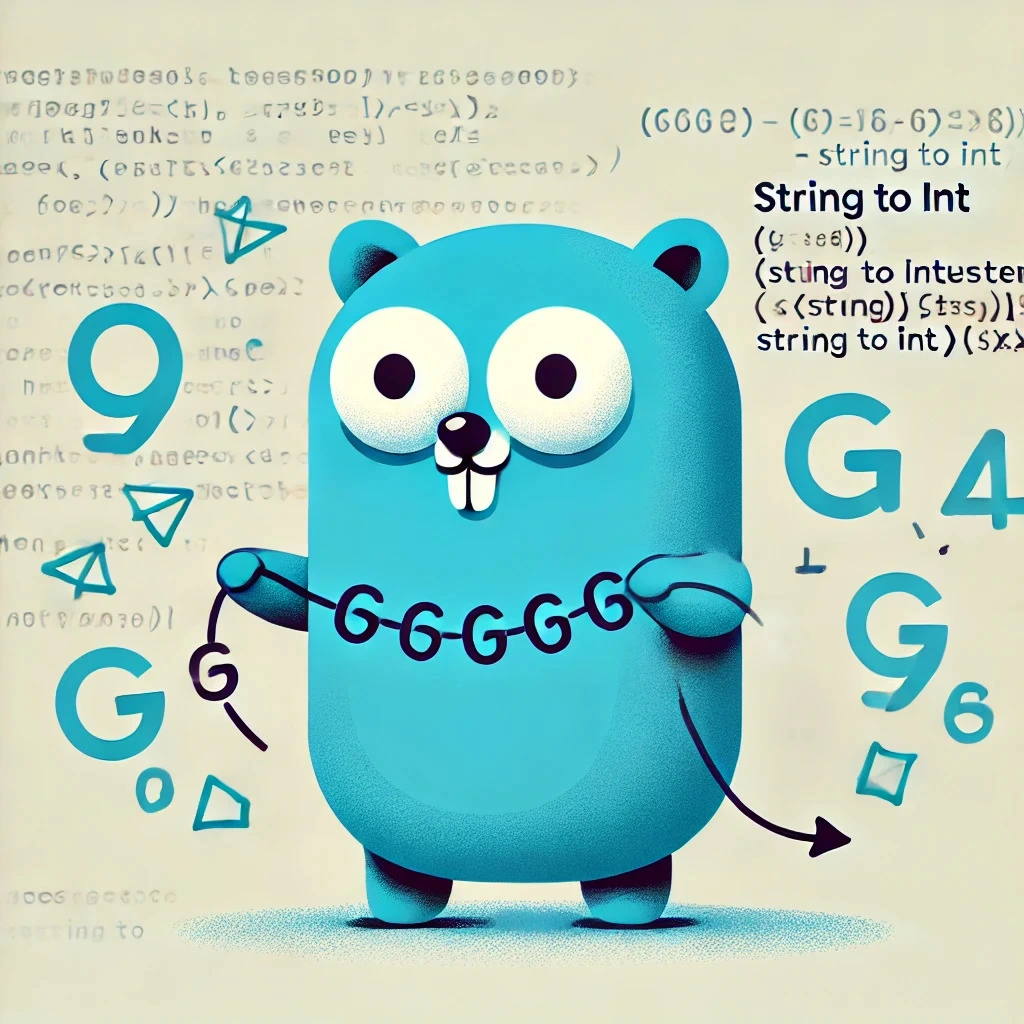
How to convert an int to string in Golang
The most straightforward and commonly used way to convert an integer to a string in Go is by using the strconv.Itoa()
function. The function name stands for “Integer to ASCII,” and it converts an integer to its string representation. Here’s an example:
package main
import (
"fmt"
"strconv"
)
func main() {
number := 123
str := strconv.Itoa(number)
fmt.Println("The string representation is:", str)
}
and here’s what it would output:
The string representation is: 123
Using Sprintf to Convert an int to a string
Another method for integer-to-string conversion is using the fmt.Sprintf
function as follows:
package main
import "fmt"
func main() {
number := 456
str := fmt.Sprintf("%d", number)
fmt.Println("Formatted string is:", str)
}
Using strconv.FormatInt()
For more control over the conversion, particularly with different integer types (such as int64), you can use strconv.FormatInt()
. This function converts an integer to a string in any base from 2 to 36. This is especially useful when you are working with larger integer types and need to convert them to strings with a specified base (for example, base 10 for decimal or base 16 for hexadecimal).
package main
import (
"fmt"
"strconv"
)
func main() {
number := int64(789)
str := strconv.FormatInt(number, 10) // Base 10 conversion
fmt.Println("Formatted string:", str)
}
Which function Should I use to Convert int to string in Go?
strconv.Itoa()
: Best for simple and efficient conversion of integers to strings without additional formatting.fmt.Sprintf()
: Ideal for when you need to format multiple values into a string, especially when you want to control how the output is structured.strconv.FormatInt()
: Useful when working with large integer types (like int64) or when you need to convert an integer to a different base.
Wrapping up
We hope you found this useful. Here are some other byteSizeGo blog posts you will enjoy: