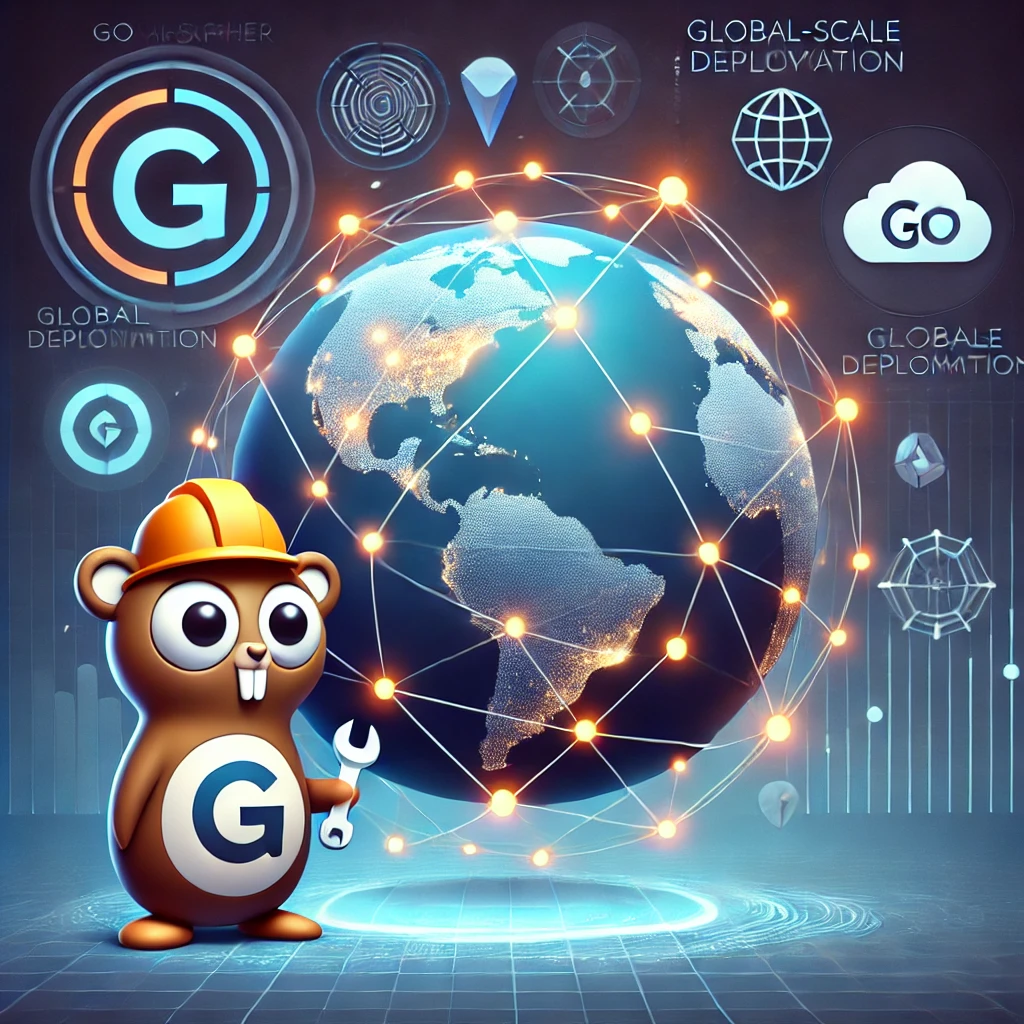
Deploying gRPC Applications Using Sevalla
If you have spent any time in the Javascript ecosystem, you will have found you can often deploy entire apps just by pushing to GitHub. Go is fairly easy to deploy generally, but it is still not quite as simple as that…until now.
Sevalla is a new platform that allows this sort of workflow for your applications. With its focus on reducing complexity, Sevalla handles networking, global deployment, and security considerations such as DDoS protection with ease. This article explores deploying a gRPC service written in Go using Sevalla, both directly from a Git repository and via Docker.
Why Sevalla?
Sevalla is a deployment platform that abstracts the intricacies of cloud infrastructure management. It helps developers by automatically building and deploying applications, handling networking configurations, and it also integrates with services like Google Cloud Platform and Cloudflare, so you get performance benefits from being able to deploy all around the globe, whilst giving you things like DDoS protection out of the box. New users receive $50 free credit to explore the platform, and that’s probably enough to run a hobby app for a while. Sevalla supports deploying applications directly from a Git repository or using Docker images, making it flexible for various workflows.
gRPC Example: Hello World
For this demonstration, we’ll use a basic “Hello World” gRPC application. The server features a SayHello
RPC that takes a name as a request and returns a personalized greeting.
service HelloService {
rpc SayHello(SayHelloRequest) returns (SayHelloResponse);
}
message SayHelloRequest {
string name = 1;
}
message SayHelloResponse {
string message = 1;
}
Below is the complete code for both the server and client implementations.
gRPC Server Code
Let’s start with the server implementation:
package main
import (
"context"
"log"
"net"
"os"
"google.golang.org/grpc"
pb "path/to/your/protobuf/package"
)
type server struct {
pb.UnimplementedHelloServiceServer
}
func (s *server) SayHello(ctx context.Context, in *pb.HelloRequest) (*pb.HelloReply, error) {
return &pb.HelloReply{Message: "Hello " + in.Name}, nil
}
func main() {
port := os.Getenv("PORT")
if port == "" {
port = "8080"
}
lis, err := net.Listen("tcp", ":"+port)
if err != nil {
log.Fatalf("failed to listen: %v", err)
}
s := grpc.NewServer()
pb.RegisterHelloServiceServer(s, &server{})
log.Printf("Server listening on port %s", port)
if err := s.Serve(lis); err != nil {
log.Fatalf("failed to serve: %v", err)
}
}
Sevalla use’s the PORT
environment variable and configures networking to expose our service with a public domain name (custom domains also supported). By default, this environment variable is set to 8080, although this can be configured.
gRPC Client Code
Now, let’s look at the client implementation:
package main
import (
"context"
"log"
"os"
"time"
"google.golang.org/grpc"
"google.golang.org/grpc/credentials/insecure"
pb "path/to/your/protobuf/package"
)
func main() {
address := os.Getenv("GRPC_HOST")
if address == "" {
log.Fatalf("GRPC_HOST environment variable not set")
}
conn, err := grpc.Dial(address, grpc.WithTransportCredentials(insecure.NewCredentials()), grpc.WithBlock())
if err != nil {
log.Fatalf("did not connect: %v", err)
}
defer conn.Close()
c := pb.NewHelloServiceClient(conn)
name := "Chris"
ctx, cancel := context.WithTimeout(context.Background(), time.Second)
defer cancel()
r, err := c.SayHello(ctx, &pb.HelloRequest{Name: name})
if err != nil {
log.Fatalf("could not greet: %v", err)
}
log.Printf("Greeting: %s", r.Message)
}
Deploying the Server via Git Repository
As mentioned, Sevalla supports direct integration with GitHub, allowing seamless deployment upon each commit. Here’s how to set up your deployment:
- Ensure the
main.go
is in the root directory: Sevalla expects yourmain.go
to reside in the root of your project. - Connect Sevalla to GitHub: Log in to Sevalla and authorize access to your GitHub repo.
- Create a Sevalla Application: In the Sevalla dashboard, click “Add Service > Application” then on the Create new application page choose Git repository.
- Select Deployment Options: Choose the corresponding repo and branch, and configure automatic deployments for every push.
- Choose the Deployment Region: Deploy in a region closest to your users for optimal performance.
- Allocate Resources: Configure CPU and RAM according to your application’s requirements.
That’s it! Now your application should start building and get deployed automatically!
Deploying the Client Using Docker
For the client, we’ll create a Docker image and deploy it through Sevalla:
- Create a Dockerfile:
# Start with a base Go image
FROM golang:1.22.1-alpine as build
# Set the working directory
WORKDIR /build
# Copy the source code
COPY . .
# Build the application
RUN GOOS=linux GOARCH=amd64 go build -ldflags "-s -w" -o /hello-client ./cmd/client
# Use a minimal base image
FROM gcr.io/distroless/base-debian10
# Copy the built binary
COPY --from=build /hello-client /hello-client
# Command to run the application
ENTRYPOINT ["/hello-client"]
- Build and Push the Docker Image
To build and push to Github container registry, use the following commands (if using a private repo, make sure you’re authenticated using a personal access token):
docker build -t gcr.io/<your-project-id>/<image-name>:latest .
docker push gcr.io/<your-project-id>/<image-name>:latest
- Deploy via Sevalla:
- Create another new application in Sevalla and select the Docker image.
- Enter your image name and tag.
- If using a private registry, you will need to set up and specify a registry credential to pull your Docker image. To add a new credential, go to Company settings > Registry credentials.
- Select region, allocate resources and deploy.
- Set the necessary environment variables, such as
GRPC_HOST
to point to your server and redeploy your server.
Monitoring and Scaling
Sevalla provides detailed analytics, including CPU, memory usage, and request metrics, offering insights into your application’s performance. You can scale resources based on real-time demand, ensuring efficient resource utilization.
Conclusion
Sevalla makes deploying gRPC applications in Go straightforward and efficient. By abstracting away the complexities of deployment, networking, and security, Sevalla allows developers to focus on building applications. With support for both Git and Docker workflows, Sevalla is flexible enough to adapt to various development pipelines. Whether you’re deploying a simple service or a complex microservices architecture, Sevalla provides the tools necessary to get your applications live quickly and securely.
You can check out Sevalla here, and if you want to learn more about building and deploying gRPC applications, you can check out our course here.