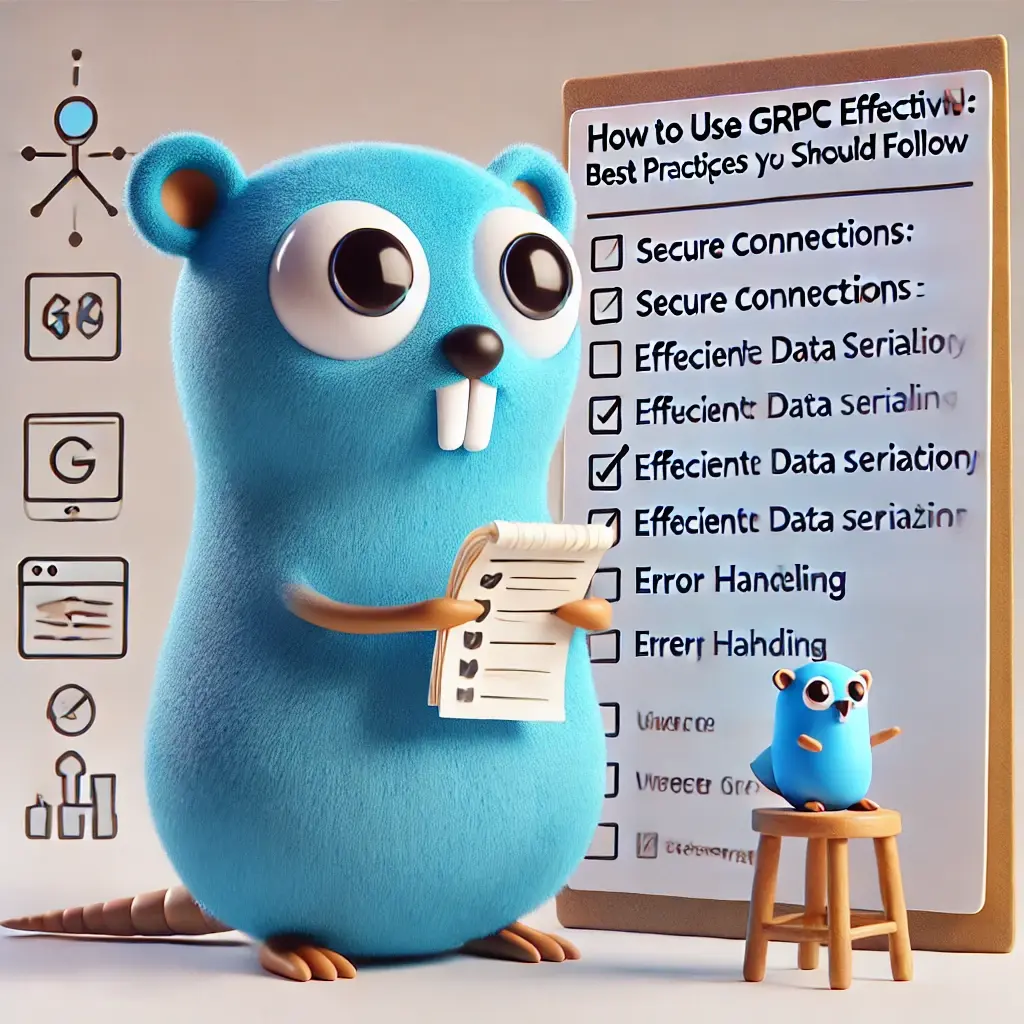
How to Use gRPC Effectively: Best Practices You Should Follow
Efficient communication between services is crucial, especially when building distributed systems. Enter gRPC, a powerful open-source remote procedure call (RPC) framework developed by Google. With its emphasis on performance and scalability, gRPC is changing the game for how applications communicate. In this guide, we’ll dive into the best practices for using gRPC effectively, helping you harness its full potential.
Understanding gRPC Basics
What is gRPC and How Does It Work?
At its core, gRPC (gRPC Remote Procedure Call) is designed to facilitate seamless communication between client and server applications. It allows clients to invoke methods on a server as if they were local calls, which streamlines the process significantly. This framework utilizes HTTP/2 and protocol buffers (protobufs) as its interface definition language (IDL), enabling both high performance and ease of use.
One of the standout features of gRPC is its use of protocol buffers, which are language-neutral and platform-neutral. This means you can define your services and message types once, and they can be used across different languages and platforms. By serializing data into a binary format, gRPC reduces message sizes and speeds up communication compared to traditional JSON-based APIs.
If you’re keen on keeping up with the latest advancements in Go, check out our free courses: What’s New in Go 1.22 and Go 1.23 in 23 Minutes. These resources can help you understand new features that may enhance your gRPC implementation. If you want to learn more about gRPC, you can check out our gRPC with Go course which will take you from zero to expert.
Why Choose gRPC?
Choosing gRPC can bring numerous advantages to your development process:
- Speed: Thanks to its binary serialization and the efficiency of HTTP/2, gRPC typically outperforms traditional REST APIs.
- Bi-directional Streaming: This feature allows both clients and servers to send and receive multiple messages simultaneously, making it ideal for real-time applications like chat services or live updates.
- Strongly Typed APIs: With protocol buffers, you get type safety, reducing the chances of errors that can occur with dynamic typing in other languages.
- Load Balancing and Failover: gRPC has built-in support for load balancing and failover, which helps ensure high availability and resilience.
gRPC vs. REST: What’s the Difference?
While REST has long been the go-to for API design, gRPC offers some compelling benefits:
- Performance: gRPC’s use of binary serialization makes it significantly faster than REST, which typically relies on slower text formats like JSON.
- Streaming Capabilities: Unlike REST, which is inherently request-response, gRPC supports streaming, making it more efficient for scenarios that involve continuous data exchange.
- Contract-First Development: gRPC encourages a contract-first approach, meaning you define your API upfront. This can simplify both client and server implementation.
Getting Started with gRPC
How to Set Up gRPC
Setting up gRPC in your project is straightforward. Here’s a quick guide for a few popular programming languages:
- Go: You can easily add gRPC by running
go get google.golang.org/grpc
. - Java: Just include the gRPC dependency in your pom.xml (for Maven) or build.gradle (for Gradle).
- Python: Install the required packages with
pip install grpcio grpcio-tools
.
Once you have your libraries set up, the next step is to create your .proto files, where you define your services and message types. If you’re looking to enhance your Go skills further, consider checking out Mastering Go with GoLand for comprehensive resources.
Best Practices for Defining .proto Files
When defining your services in .proto files, consider these best practices to make your APIs clear and efficient:
- Use Clear and Descriptive Names: Meaningful names for your services and messages can make a world of difference. They should convey the purpose of the service effectively.
- Keep Messages Lean: Aim for smaller messages that only include necessary fields. This helps improve performance and reduces serialization time.
- Version Your Protobufs: As your application evolves, it’s crucial to maintain backward compatibility. Implement versioning to handle updates while ensuring existing clients remain unaffected.
- Document Everything: Adding comments in your .proto files can provide context and explanations that are invaluable for future developers or even your future self.
Useful Tools for gRPC Development
To make your development process smoother, consider using these handy tools:
- gRPC Gateway: This tool helps create a RESTful JSON API on top of your gRPC services, making them more accessible to clients that don’t support gRPC natively.
- Postman: With gRPC support, Postman makes it easier to test your APIs during development, providing a familiar interface for many developers.
- grpcui: This user-friendly GUI client is designed specifically for testing gRPC services, allowing for quick interactions and easier debugging.
Implementing gRPC Services
Best Practices for Building gRPC Services
When it comes to designing your gRPC services, consider these best practices:
- Focus on Specific Capabilities: Each service should target a distinct business capability. This not only makes your services easier to understand but also improves maintainability.
- Leverage Streaming: If your application requires real-time data processing or deals with large datasets, take advantage of gRPC’s streaming capabilities for better efficiency.
- Implement Comprehensive Error Handling: Clearly communicate errors to clients using gRPC’s status codes, which helps in troubleshooting and enhances the user experience.
- Consider Caching: For data that’s frequently accessed, implementing caching strategies can significantly reduce the load on your services and speed up response times.
Ensuring Security in gRPC
Security is always a priority when it comes to service communication. gRPC provides several options to enhance security:
- SSL/TLS Encryption: Ensure your data is secure in transit by implementing SSL/TLS to encrypt communications between clients and servers.
- Token-Based Authentication: Use token-based methods like JSON Web Tokens (JWT) to manage user authentication without exposing credentials.
- OAuth2 for Access Control: Implement OAuth2 to give users secure access to your services, ensuring that permissions are managed effectively.
Managing Service Versioning
As your services evolve, effective versioning becomes crucial to avoid disrupting existing clients. Here are some strategies:
- Follow Semantic Versioning: Use a versioning scheme like major.minor.patch to communicate the impact of changes clearly.
- Separate Service Names for Major Changes: When making significant changes, consider creating new service definitions rather than altering existing ones to prevent breaking existing clients.
- Provide Deprecation Notices: If you need to phase out a feature, give clients ample notice and guidance on how to transition smoothly.
Optimizing gRPC Performance
Tips for Boosting gRPC Performance
To keep your gRPC services running at peak performance, here are some strategies to consider:
- Conduct Regular Load Testing: Perform load testing to identify and address performance bottlenecks before they become issues.
- Optimize Message Sizes: Regularly review your message definitions to ensure they’re as efficient as possible, which can lead to reduced latency.
- Connection Management: Reuse existing connections whenever possible instead of creating new ones for each request. This can dramatically cut down on overhead.
Effective Error Handling
Good error handling is essential in any distributed system. With gRPC, you can implement:
- Custom Error Codes: Use gRPC’s status codes to provide meaningful feedback on what went wrong.
- Automatic Retries for Transient Errors: Implement retry logic with exponential backoff to gracefully handle temporary issues without disrupting the user experience.
Monitoring and Logging
Monitoring your gRPC services is critical for maintaining their health and performance. Here’s how to do it effectively:
- Distributed Tracing: Use tools like OpenTelemetry to trace requests as they flow through your services, which can help you identify performance issues and bottlenecks.
- Centralized Logging: Implement solutions like Fluentd or Logstash to gather logs from all your services in one place, making it easier to analyze and troubleshoot issues.
- Track Key Metrics: Monitor essential metrics such as latency, error rates, and throughput with tools like Prometheus or Grafana. This data can provide valuable insights into the health of your services.
FAQs
Why should I choose gRPC over REST?
gRPC offers significant advantages such as improved performance through binary serialization, built-in bi-directional streaming, and a contract-first approach with protobufs, making it an excellent choice for various applications.
How do I define a gRPC service?
You define a gRPC service in .proto files, specifying the service methods along with their request and response message types. Clear naming conventions help enhance readability and understanding. In our course we’ll teach you how to do this and much more.
What’s the best way to handle errors in gRPC?
Effective error handling includes using gRPC’s status codes to indicate success or failure and providing clear error messages. Implementing retry logic for transient errors can also improve user experience.
How can I optimize the performance of my gRPC services?
To optimize performance, focus on minimizing message sizes, reusing connections, conducting load testing, and implementing caching strategies for frequently accessed data. Monitoring your services is essential for identifying potential issues.
What tools are best for testing gRPC services?
For testing gRPC services, tools like Postman (with gRPC support), gRPCUI for a GUI approach, and distributed tracing tools are excellent options for simplifying testing and debugging.