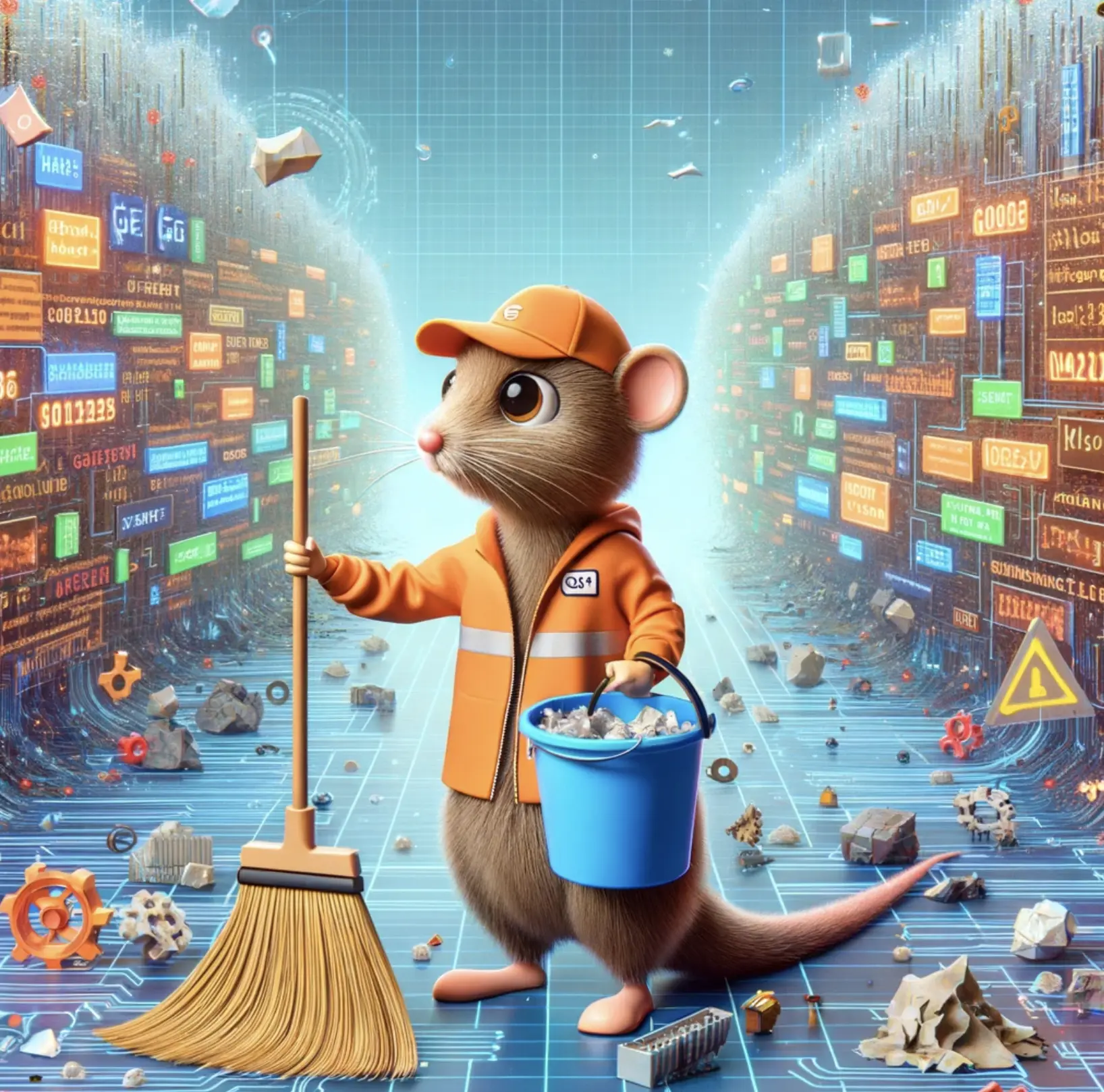
Garbage Collection In Go
For most of us, garbage collection is something we have heard of but never need to pay attention to. However, one of the reasons #golang is so efficient and easy to learn is due to its excellent GC.
What is Garbage Collection?
Garbage collection is the process of automatically reclaiming memory that is no longer in use or referenced by your application. Manual memory management, as seen in languages like C and C++, can be error-prone. GC aims to automate this process, allowing developers to focus on logic rather than memory management.
How Does GC Work in Go?
Go’s garbage collector is a concurrent, tri-color, mark-sweep collector. This means:
-
Concurrent: Most of the GC work is done while the Go program is still running, leading to minimal pause.
-
Tri-color: This involves categorizing object sets into three colors—white, grey, and black, representing objects that are unprocessed, currently being processed, and complete.
-
Mark-Sweep: The process consists of a mark phase, where live objects are identified, followed by a sweep phase, where the memory of unreachable objects is reclaimed.
Note: Most of the concepts listed above are not unique to Go. For a deeper understanding, you can refer to articles such as Mark and Sweep Garbage Collection Algorithm and Garbage Collection.
Can you manually manage GC in Go? When should you do that?
Yes, you can! Check out Go’s GC Guide for a detailed guide on tuning and running the GC.
For most of us, you’ll never need to do this. However, if you are optimizing for incredibly low latency, have a segment of code that allocates a lot of memory, or you know there are idle periods in your code (e.g., between requests), you may want to trigger the GC manually.