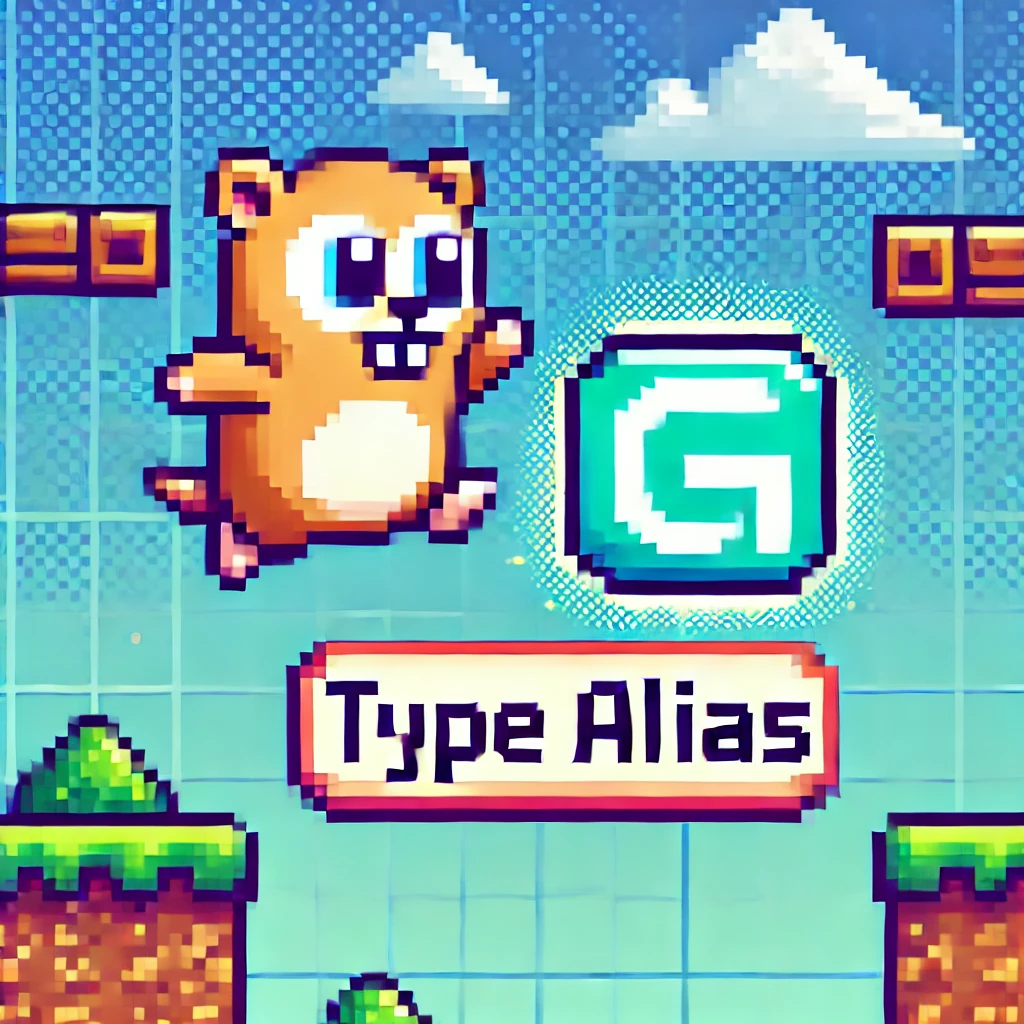
Exploring Generic Type Aliases in Go 1.24
Go 1.24 introduces generic type aliases, enabling you to define type aliases with their own type parameters. This change allows more flexible and maintainable code, especially during refactoring.
Understanding Type Definitions and Type Aliases
Go has always supported custom type definitons and type aliases. Let’s review them quickly.
-
Type Definition: Creates a new, distinct type based on an existing one. For example:
type Celsius float64 type Fahrenheit float64
Here,
Celsius
andFahrenheit
are distinct types, even though they share the underlying typefloat64
. -
Type Alias: Provides an alternative name for an existing type without creating a new type. For example:
type Manager = Employee
In this case, Manager
is simply another name for Employee
, and the two are interchangeable.
Introducing Generic Type Aliases in Go 1.24
Prior to Go 1.24, type aliases couldn’t have their own type parameters. With the new release, you can define a type alias with its own type parameters( Source).
Practical Example: Refactoring with Generic Type Aliases
Consider a scenario where you have a generic type Container
in a package named oldpkg
:
// oldpkg/container.go
package oldpkg
type Container[T any] struct {
Value T
}
You aim to move Container
to a new package newpkg
while maintaining backward compatibility. With Go 1.24’s generic type aliases, this process becomes straightforward.
First, define the Container
type in the new package:
// newpkg/container.go
package newpkg
type Container[T any] struct {
Value T
}
Next, in the old package, create a type alias that references the new package’s Container
:
// oldpkg/container.go
package oldpkg
import "path/to/newpkg"
type Container[T any] = newpkg.Container[T]
This setup ensures that any code importing oldpkg
continues to function seamlessly, as oldpkg.Container
is now an alias for newpkg.Container
.
Using the Refactored Generic Type Alias
Here’s how you can use the Container
type after refactoring:
package main
import (
"fmt"
"path/to/oldpkg"
)
func main() {
// Create a Container for an integer
intContainer := oldpkg.Container[int]{Value: 42}
fmt.Println(intContainer.Value) // Output: 42
// Create a Container for a string
stringContainer := oldpkg.Container[string]{Value: "Hello, Go 1.24!"}
fmt.Println(stringContainer.Value) // Output: Hello, Go 1.24!
}
In this example, oldpkg.Container
is used to create containers for different types (int
and string
). The type alias ensures that the underlying implementation from newpkg
is used, promoting code consistency and maintainability.
Benefits of Generic Type Aliases
-
Simplified Refactoring: Moving generic types between packages becomes more straightforward without breaking existing code.
-
Enhanced Readability: Provides clearer and more concise code, especially when dealing with complex generic types.
-
Flexibility: Allows for the creation of more adaptable and reusable code components.
For more detailed information, refer to the Go 1.24 Release Notes.