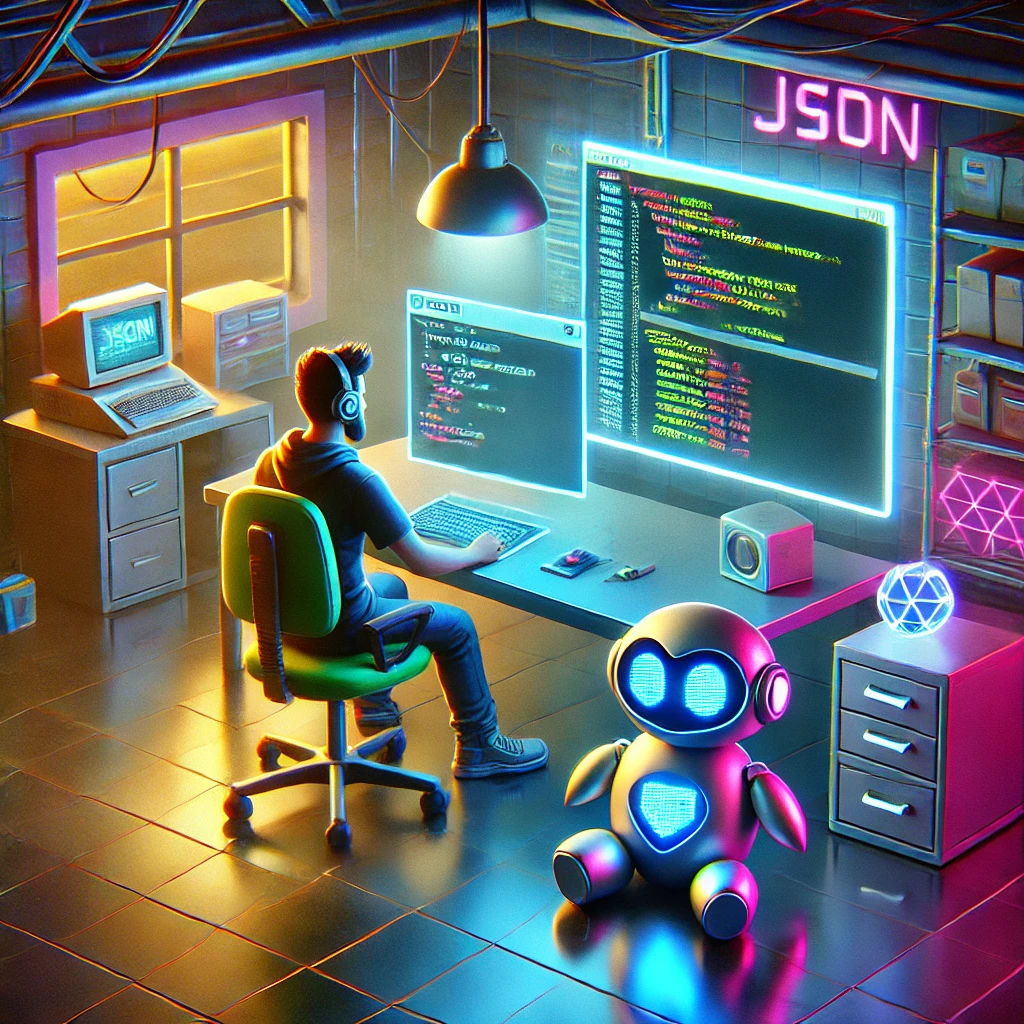
Understanding the New JSON Output in ‘go build’ and ‘go test’ Commands in Go 1.24
Go 1.24 brings JSON output support for the go build
and go test
commands. This feature allows developers to programmatically analyze build and test results, making it easier to integrate these commands into CI/CD pipelines and custom tooling.
In this blog post, we’ll explore what this new feature offers, how to use it, and scenarios where it can improve your workflow.
What’s New in Go 1.24?
With Go 1.24, you can now pass flags to the go build
and go test
commands to produce JSON-formatted output. This structured, machine-parsable output provides detailed information about the process and results, including errors, warnings, and test results, in a format that is easy for computers.
Previously, parsing the plain-text output of these commands required custom scripts or regex patterns, which could be error-prone and difficult to maintain. The JSON output solves this problem by offering a well-defined structure.
Why JSON Output Matters
The JSON output is designed to:
- Simplify Automation: Tools and scripts can now reliably interpret the output without complex parsing logic.
- Enable Advanced Analytics: Developers can analyze build and test data programmatically to uncover trends and patterns.
- Enhance Debugging: Detailed and structured error information makes it easier to identify and fix issues. We love debugging here at byteSizeGo and think its well worth optimizing for!
Using JSON Output with go build
To enable JSON output for go build
, use the -json
flag. For example:
go build -json ./...
This command will output detailed information about the build process in JSON format. Each line of output is a JSON object, making it easy to process the data line by line.
Example Output
{
"Mode": "build",
"Package": "example.com/myproject",
"Action": "compile",
"Error": null
}
Key Fields
- Mode: Specifies the command mode (e.g.,
build
ortest
). - Package: The package being built.
- Action: The current action (e.g.,
compile
,link
). - Error: Contains error details if an issue occurs.
Using JSON Output with go test
Similarly, you can enable JSON output for go test
by combining the -json
flag with -v
(verbose output):
go test -json ./...
This command produces structured data for each test case, including pass/fail status, execution time, and error messages.
Example Output
{
"Action": "run",
"Package": "example.com/myproject",
"Test": "TestExampleFunction",
"Elapsed": 0.012,
"Output": "PASS\n"
}
Key Fields
- Action: Indicates the current test action (
run
,pass
,fail
). - Package: The package containing the test.
- Test: The name of the test being executed.
- Elapsed: The time taken to run the test.
- Output: The raw output of the test case.
Best Practices for JSON Output
- Use Parsers: Use JSON parsers in your preferred language (e.g.,
encoding/json
in Go) to process the output. - Log Output: Save the JSON output to files for debugging or historical analysis.
- CI/CD Integration: Integrate the JSON output with your CI/CD pipelines to automatically parse and report build and test results.
Example: Parsing JSON Output in Go
Here’s a simple example of how to parse the JSON output in Go:
package main
import (
"encoding/json"
"fmt"
"os"
)
type BuildOutput struct {
Mode string `json:"Mode"`
Package string `json:"Package"`
Action string `json:"Action"`
Error string `json:"Error"`
}
func main() {
decoder := json.NewDecoder(os.Stdin)
for {
var output BuildOutput
if err := decoder.Decode(&output); err != nil {
break
}
fmt.Printf("Processed: %+v\n", output)
}
}
Limitations and Considerations
- Line-by-Line Parsing: Each line of the output is a separate JSON object, requiring line-by-line parsing.
- Compatibility: Ensure that all team members use Go 1.24 or later to avoid compatibility issues.
- Performance: Generating JSON output may slightly impact performance for very large projects.
Wrapping Up
Happy Go1.24-ing! We have more discussion about the features being added on the rest of our blog so take a look!