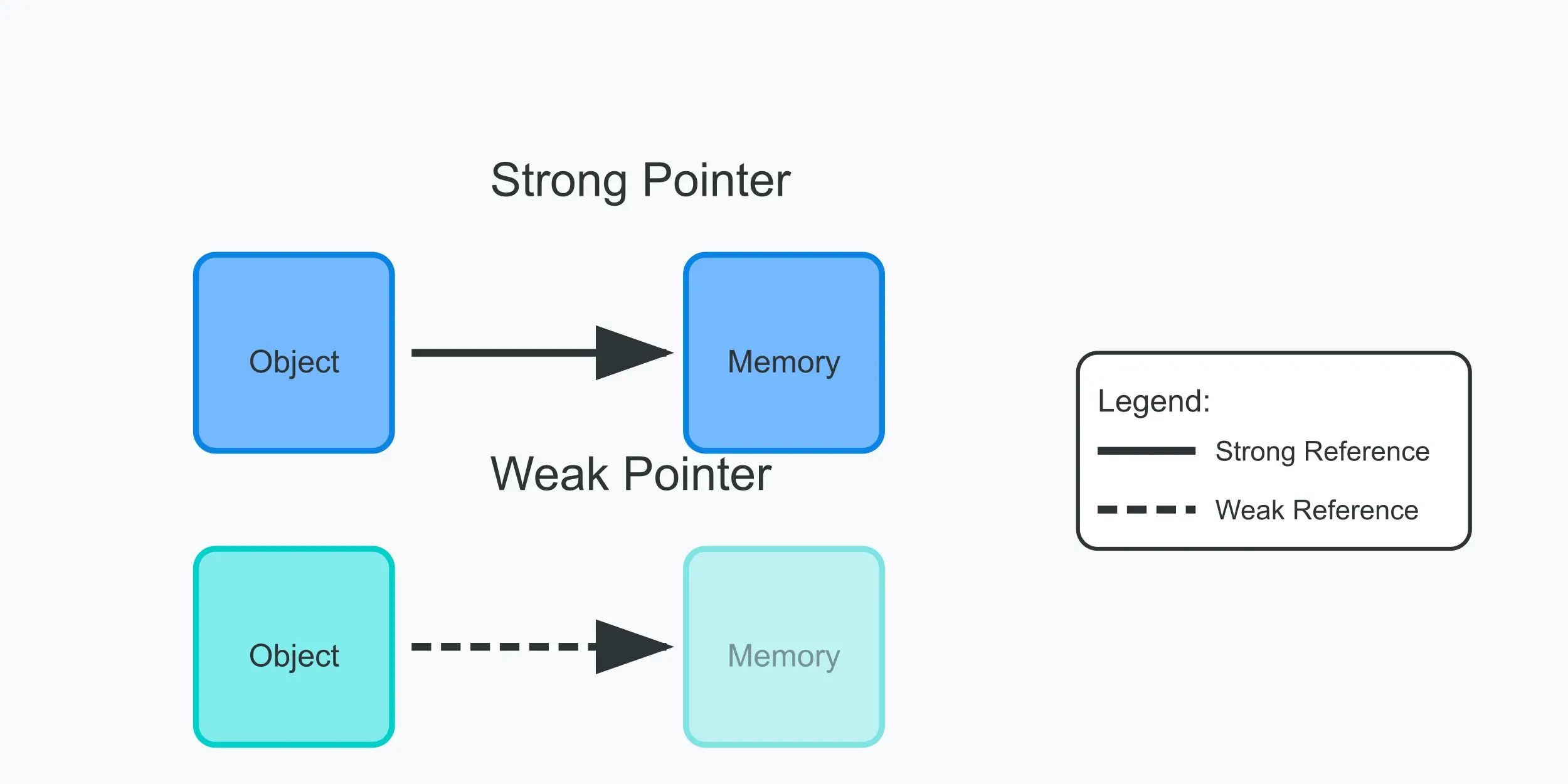
Introducing weak pointers in Go 1.24
Go 1.24 just dropped a feature called weak pointers. Don’t worry if that sounds confusing - we’ll walk through what they are and why you might use them in this blog.
What’s a Weak Pointer Anyway?
Think of a regular pointer in Go as a strong grip on an object - as long as you’re holding it, Go’s garbage collector can’t take it away. A weak pointer is more like a loose grip - you can point to something, but if nobody else is holding onto it strongly, the garbage collector can still clean it up.
Why Should You Care?
Here are some things you can do with weak pointers:
- Make Better Caches: Your browser caches images so they load faster next time. Weak pointers let you build caches that clean themselves up when you don’t need the stuff anymore.
- Save Memory: If you’re making a big program that needs to keep track of lots of things, weak pointers can help you avoid wasting memory.
- Build Smarter Data Structures: You can make maps and lists that don’t accidentally keep things in memory forever. Maps also got faster in Go 1.24,so take a read of this too.
Let’s See It in Action!
Here’s a simple example of how you might use weak pointers to build a cache that cleans itself up (and you can find more examples in the official docs):
// This is like a smart cabinet that forgets about stuff
// when you're not using it anymore
type SimpleCache struct {
stuff sync.Map
}
// Put something in the cabinet
func (c *SimpleCache) Store(key string, value interface{}) {
ptr := weak.New(value) // Create a weak grip on the value
c.stuff.Store(key, ptr)
}
// Try to get something back
func (c *SimpleCache) Get(key string) (interface{}, bool) {
ptr, found := c.stuff.Load(key)
if !found {
return nil, false // Nothing here!
}
// Try to grab what we're pointing to
value := ptr.(*weak.Pointer[interface{}]).Get()
if value == nil {
// Oops, it's gone! Clean up our empty pointer
c.stuff.Delete(key)
return nil, false
}
return value, true
}
Tips for Using Weak Pointers
Here are some important things to remember:
- Always Check for nil: When you try to get something through a weak pointer, it might be gone already. Always check! Learn more about nil handling in Go.
- Don’t Overuse Them: Weak pointers should be used rarely - they’re super helpful for specific jobs but you don’t need them everywhere.
- Be Careful with Multiple Users: If your program has lots of things happening at once, make sure to use locks or sync.Map to keep everything safe.
What’s Different About Go’s Weak Pointers?
Other programming languages have weak pointers too! But Go made them really simple to use and hard to mess up - which is pretty much Go’s whole thing. They work great with Go’s other features like garbage collection and concurrency.
Wrapping Up
Weak pointers are a new tool in Go 1.24. They’re perfect when you need to point to stuff but don’t want to force it to stick around forever. Think of them as “friendly suggestions” rather than firm grips - they let you reference things without being too clingy.
Even though they’re doing some pretty complex stuff under the hood, Go makes them really straightforward to use. Whether you’re building a cache, making a game, or just trying to make your programs use less memory, weak pointers might be just what you need!
Want to learn more? Check out these resources: