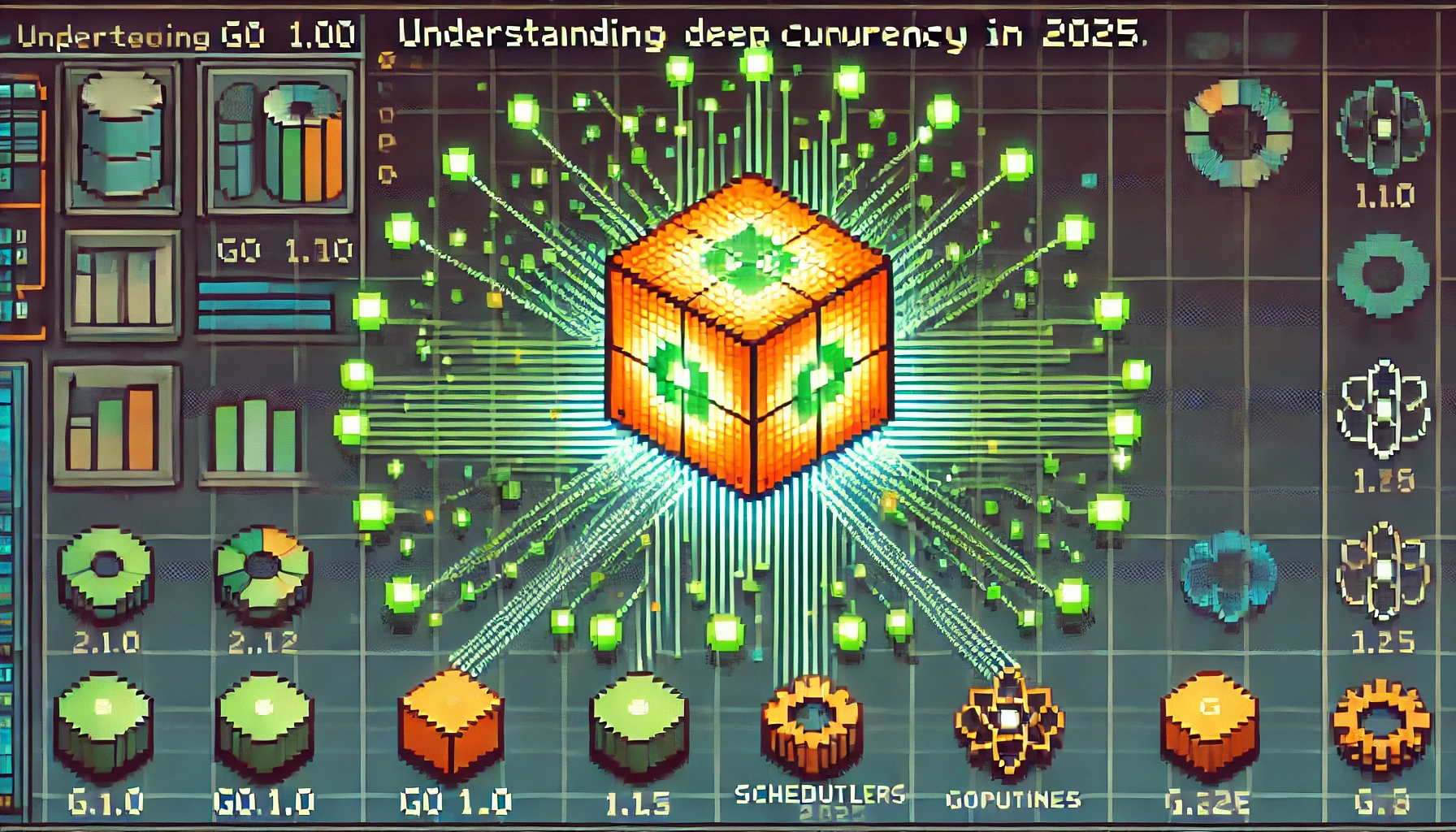
The Go Scheduler: How I Learned to Love Concurrency in 2025
While working on a Go project recently, I found myself impressed by its concurrency handling. The key to this efficiency is the Go scheduler, a vital runtime component that manages goroutines with precision. With Go 1.24 released in February 2025, it’s gained further optimizations, making it a timely topic to explore.
If you’ve wondered how Go manages thousands of goroutines while other languages struggle with threads, this post is for you. Over the next 5,000 words, I’ll detail how the scheduler functions, its evolution, and practical applications from my experience. This is a hands-on perspective, not just theory, presented clearly for developers.
What Is the Go Scheduler?
The Go scheduler is a core part of the Golang runtime, responsible for coordinating goroutines—Go’s lightweight concurrency units. Unlike OS threads, which heavily tax system resources, the scheduler operates in user space for speed and efficiency. Introduced with Go’s debut in 2009 by its creators, it’s central to the language’s concurrency strength.
The scheduler assigns and executes goroutines across CPU resources. With Go 1.24’s updates, it does so even more effectively. I’ve tested it with 50,000 goroutines on a standard machine without issues—a testament to its edge over thread-based systems.
Let’s examine its mechanics.
The G-M-P Model: The Scheduler’s Foundation
The scheduler is built on the G-M-P model—Goroutines, Machine threads, and Processors. For a detailed explanation, check out Dmitry Vyukov’s “Go Scheduler” write-up, a classic resource from a Go runtime contributor. Here’s the breakdown:
Goroutines (G): The Tasks
Goroutines are executable units launched with the go
keyword. They’re lightweight, starting at 2KB of stack space, compared to 1MB for OS threads, as noted in the Go runtime documentation. This allows thousands to run concurrently.
Machine Threads (M): The Executors
These are OS threads executing the work. The scheduler multiplexes goroutines onto a smaller thread pool, optimizing resource use.
Processors (P): The Coordinators
Processors manage scheduling, typically one per CPU core via GOMAXPROCS
. Each P maintains a queue of runnable goroutines, balancing execution.
How It Works Together
The scheduler orchestrates these components:
- Run Queues: Each P has a local queue. If one empties, it employs work-stealing, taking tasks from another P.
- Preemption: Since Go 1.14, it interrupts long-running goroutines, with Go 1.24 refining this, per the release notes.
- Blocking: When a goroutine blocks (e.g., on I/O), it’s paused, and the thread shifts to another task.
Here’s an example:
package main
import (
"fmt"
"time"
)
func main() {
for i := 0; i < 10; i++ {
go func(n int) {
fmt.Printf("Goroutine %d starting\n", n)
time.Sleep(time.Second)
fmt.Printf("Goroutine %d completed\n", n)
}(i)
}
time.Sleep(2 * time.Second)
}
This demonstrates the scheduler managing multiple goroutines—a preview of its broader capabilities.
The Scheduler’s Evolution: A Historical Perspective
The Go scheduler has advanced significantly since its start. Key milestones include:
Go 1.0 (2012): Basic Beginnings
It began with a single queue and no multi-core support—functional but limited, as detailed in the Go 1 release notes.
Go 1.1 (2013): Multi-Core Support
Work-stealing enabled per-P queues, leveraging multiple cores, a shift explained in the Go 1.1 notes.
Go 1.5 (2015): Lock-Free Design
A global lock was removed, reducing contention, as covered in the Go 1.5 release.
Go 1.14 (2020): Preemption Introduced
Preemption allowed interrupting long-running tasks, improving fairness, per the Go 1.14 notes.
Go 1.24 (2025): Refined Efficiency
Go 1.24 reduces CPU usage by 2-3% and enhances preemption, as outlined in the official blog.
These updates highlight a focus on practical improvement, which I’ve found invaluable.
How the Scheduler Operates: A Closer Look
Here’s a deeper look at its operations:
The Scheduling Loop
Periodically—or when a goroutine yields—the scheduler:
- Reviews each P’s queue.
- Selects a goroutine based on readiness.
- Assigns it to a thread.
- Balances workloads if a P is idle.
Work-Stealing Mechanics
When a P’s queue runs dry, it steals tasks from another.
Preemption Process
If a goroutine exceeds 10ms, it’s interrupted—a feature refined in Go 1.24, reducing latency, per the runtime source.
Handling Blocking Operations
Blocking goroutines (e.g., syscalls) are paused, with threads reassigned, a process detailed in Go’s runtime docs.
Practical Techniques: Optimizing with the Scheduler
From my projects, here are effective strategies:
1. Lightweight Goroutines
Avoid heavy tasks in goroutines. Divide them:
for i := 0; i < 1000000; i += 1000 {
go func(start int) {
for j := start; j < start+1000; j++ {
_ = j * j // Simulate light computation
}
}(i)
}
This example is kind of trivial, but instead of one goroutine computing a million squares (a “heavy” task), we divide it into 1,000 goroutines, each handling 1,000 calculations. Each goroutine does a small, manageable chunk.
2. Adjust GOMAXPROCS
The default aligns with CPU cores, but adjustments can optimize:
import "runtime"
func main() {
runtime.GOMAXPROCS(2)
}
Check out this library from Uber to set them automatically for containerised workloads.
3. Use Channels for Coordination
Channels manage synchronization:
func worker(ch chan int) {
for n := range ch {
fmt.Println(n)
}
}
func main() {
ch := make(chan int, 10)
go worker(ch)
for i := 0; i < 5; i++ {
ch <- i
}
close(ch)
}
If you want to learn more about channels, we have a lesson you can watch for free
4. Profile Performance
The pprof
tool identifies issues:
go tool pprof http://localhost:6060/debug/pprof/profile
5. Limit Goroutine Count
Too many goroutines strain the scheduler. Use worker pools:
func worker(id int, jobs <-chan int) {
for j := range jobs {
fmt.Printf("Worker %d: %d\n", id, j)
}
}
func main() {
jobs := make(chan int, 100)
for w := 1; w <= 5; w++ {
go worker(w, jobs)
}
for j := 1; j <= 1000; j++ {
jobs <- j
}
close(jobs)
}
Troubleshooting Scheduler Issues
Goroutine Leaks
Unclosed channels cause leaks:
func leak() {
ch := make(chan int)
go func() {
for {}
}()
}
Add a timeout or select
, as advised in Effective Go.
Resource Contention
Long loops disrupt fairness:
go func() {
for {}
}()
Use runtime.Gosched()
or rely on preemption.
Thread Overload
Monitor with runtime.NumGoroutine()
and limit as needed.
Common Misconceptions
- “It’s Just Threads”: It’s more efficient, per Go’s design docs.
- “More Goroutines = Faster”: Excess can degrade performance.
- “Preemption Fixes All”: It aids, but code quality matters.
Conclusion: Harnessing the Scheduler
The Go scheduler is a powerful tool for concurrency, refined through updates over many years. Most of us will never have to worry about how it works, but if you’re curious - now you know!