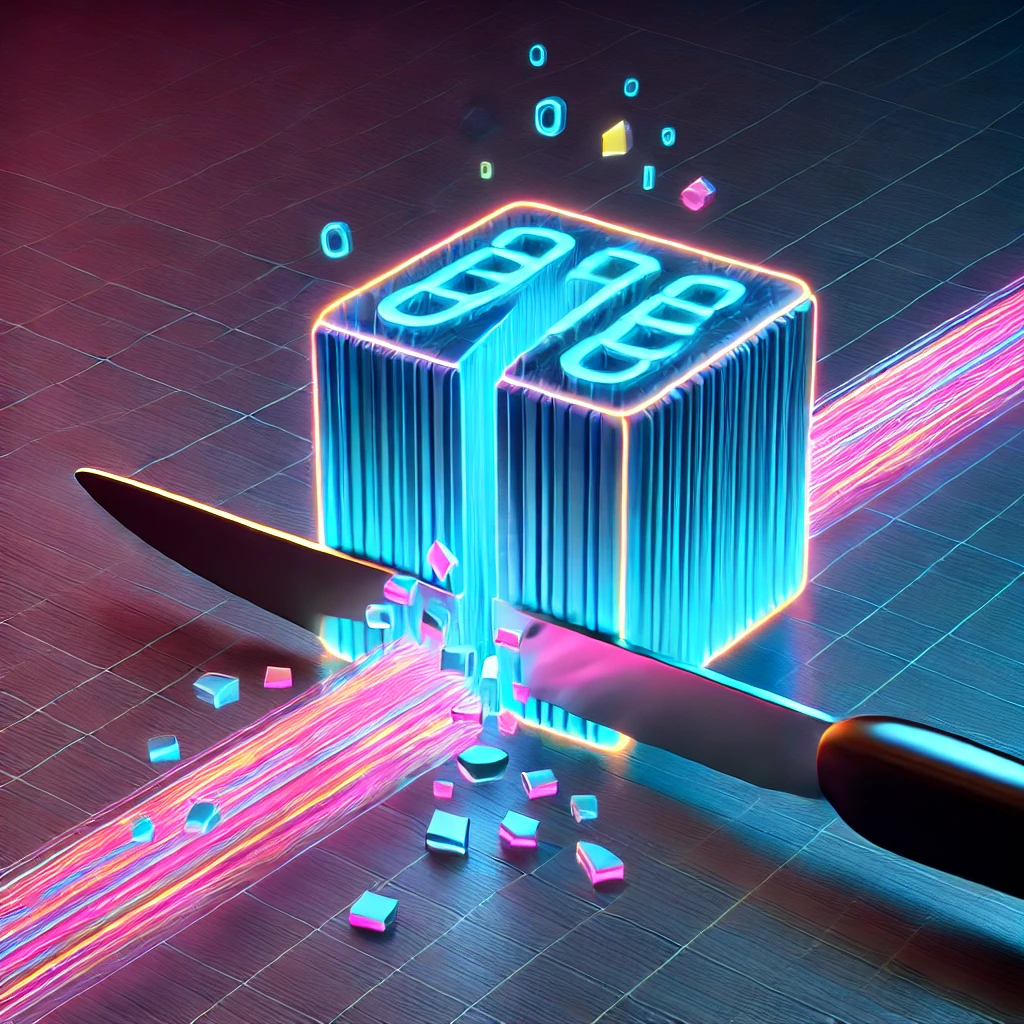
Converting byte to string in Go
Working with data in Golang often involves converting between different data types. One common task is converting bytes to strings, especially when dealing with file I/O, network communication, or data encoding. In this blog post, we’ll dive deep into various methods to convert bytes to strings in Golang, understand the nuances, and explore best practices.
Table of Contents
- Understanding Bytes and Strings in Golang
- Simple Conversion Using
string()
- Using
strconv
Package - Converting with
bytes
Buffer - Handling Encoding and Decoding
- Potential Pitfalls and How to Avoid Them
- Best Practices
- Conclusion
Understanding Bytes and Strings in Golang
In Golang, a byte
is an alias for uint8
, representing an 8-bit unsigned integer. A string, on the other hand, is a read-only slice of bytes. Understanding this relationship is crucial when converting between the two.
var b byte = 'A' // ASCII value 65
var s string = "Hello, World!"
Simple Conversion Using string()
The simplest way to convert a byte slice ([]byte
) to a string is by using the string()
function.
Example:
package main
import (
"fmt"
)
func main() {
byteSlice := []byte{72, 101, 108, 108, 111}
str := string(byteSlice)
fmt.Println(str) // Output: Hello
}
Explanation:
- Input: A byte slice containing ASCII values.
- Process: Convert the byte slice to a string using
string(byteSlice)
. - Output: The string “Hello”.
Using strconv
Package
For more control over the conversion, especially when dealing with individual bytes, the strconv
package can be useful.
Example:
package main
import (
"fmt"
"strconv"
)
func main() {
b := byte(65)
str := strconv.Itoa(int(b))
fmt.Println(str) // Output: 65
}
Explanation:
- Input: A single byte with the ASCII value for ‘A’.
- Process: Convert the byte to an integer and then to a string.
- Output: The string “65”.
However, note that this converts the byte’s numeric value to its string representation, not the character it represents.
Converting with bytes
Buffer
When working with larger data or streaming data, using a bytes.Buffer
can be efficient.
Example:
package main
import (
"bytes"
"fmt"
)
func main() {
var buffer bytes.Buffer
byteSlice := []byte("Hello, World!")
buffer.Write(byteSlice)
str := buffer.String()
fmt.Println(str) // Output: Hello, World!
}
Explanation:
- Input: A byte slice containing the string “Hello, World!”.
- Process: Write the byte slice to a
bytes.Buffer
and then convert it to a string. - Output: The string “Hello, World!”.
Handling Encoding and Decoding
When dealing with text that may contain non-ASCII characters, it’s essential to handle encoding properly.
Example with UTF-8:
package main
import (
"fmt"
)
func main() {
byteSlice := []byte{0xe6, 0x97, 0xa5, 0xe6, 0x9c, 0xac}
str := string(byteSlice)
fmt.Println(str) // Output: 日本
}
Explanation:
- Input: A byte slice representing the UTF-8 encoding of “日本” (Japan).
- Process: Convert the byte slice to a string using
string(byteSlice)
. - Output: The string “日本”.
Using encoding
Packages:
For other encodings, use the golang.org/x/text/encoding
packages.
package main
import (
"fmt"
"golang.org/x/text/encoding/charmap"
"log"
)
func main() {
byteSlice := []byte{0xE4, 0xF6, 0xFC} // ISO 8859-1 encoded bytes
decoder := charmap.ISO8859_1.NewDecoder()
str, err := decoder.String(string(byteSlice))
if err != nil {
log.Fatal(err)
}
fmt.Println(str) // Output: äöü
}
Explanation:
- Input: A byte slice with ISO 8859-1 encoded bytes.
- Process: Use a decoder to properly interpret the bytes.
- Output: The string “äöü”.
Potential Pitfalls and How to Avoid Them
1. Misinterpreting Byte Values
Issue: Converting bytes directly to strings without considering encoding can lead to misinterpretation.
Solution: Always ensure that the byte slice is encoded correctly for direct conversion.
2. Modifying Strings
Strings in Golang are immutable. Trying to modify a string will result in a compilation error.
Example:
str := "Hello"
str[0] = 'h' // Error: Cannot assign to str[0]
Solution: Convert the string to a byte slice, modify it, and then convert it back.
str := "Hello"
byteSlice := []byte(str)
byteSlice[0] = 'h'
str = string(byteSlice)
fmt.Println(str) // Output: hello
3. Performance Considerations
Repeated conversions can be costly in performance-critical applications.
Solution: Minimize conversions and consider using buffers or byte slices directly when appropriate.
Best Practices
- Understand Encoding: Always be aware of the text encoding of your byte slices.
- Use Appropriate Packages: Leverage standard library packages like
bytes
,strconv
, and external packages for encoding. - Minimize Conversions: Avoid unnecessary conversions to optimize performance.
- Handle Errors: Always check for errors during conversions, especially when dealing with external data.
Conclusion
Converting bytes to strings in Golang is straightforward, but it requires an understanding of how bytes and strings are represented in memory and how encoding affects the conversion. By using the methods and best practices outlined in this guide, you can handle byte-to-string conversions efficiently and correctly in your Golang applications.
If you’re just getting started with Go, check out our free course on Mastering Go with GoLand which will help you get started, and you’ll get a free one-year license to GoLand for completing it!
Happy Golang!