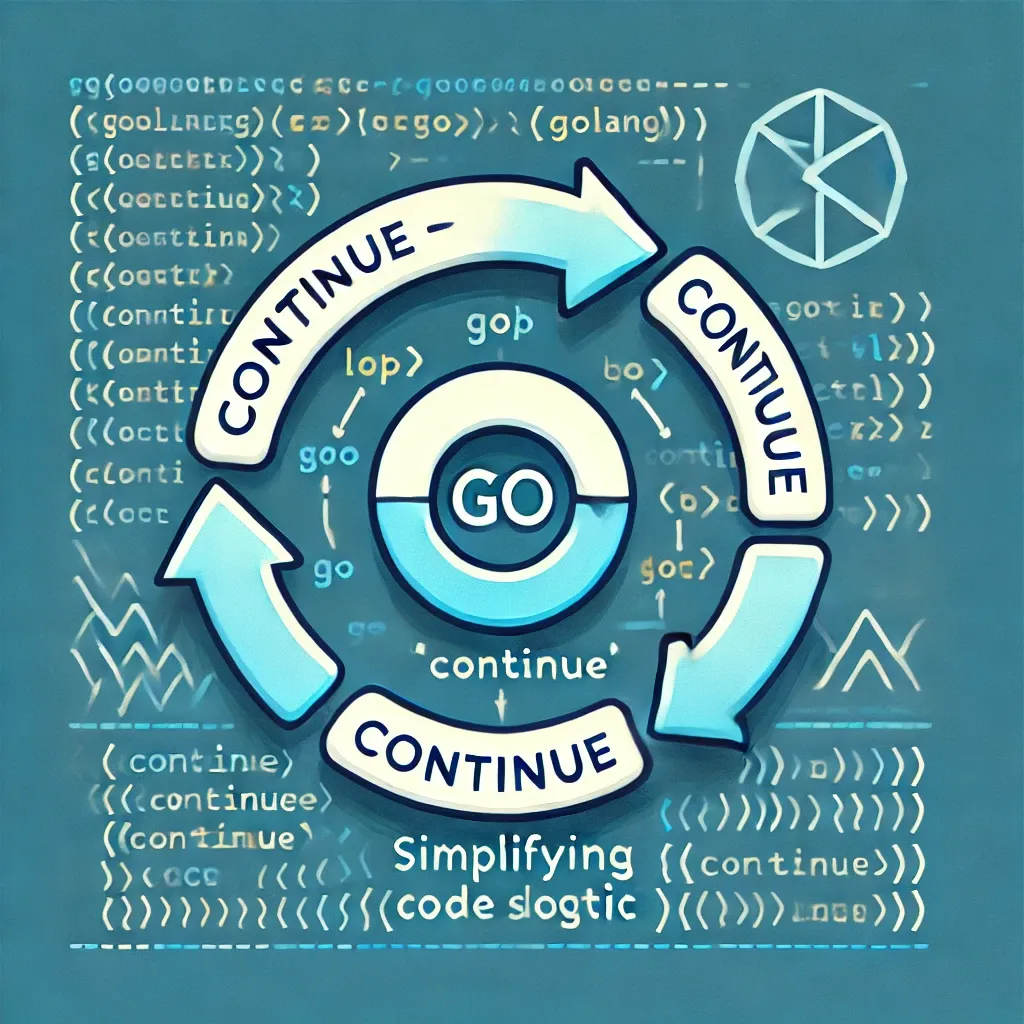
Golang continue: Simplifying Your Loop Logic for Cleaner Code
When it comes to programming, loops are the backbone of repetition, data processing, and efficient workflows. In Go, loops are straightforward, yet powerful. But there’s a trick that many Go developers might overlook: the continue
statement. This little command can actually make your loops simpler, cleaner, and a lot easier to read. In this guide, we’re going to dig into what continue
does, why it’s useful, and how to make the most of it in your code.
And hey, if you’re serious about leveling up in Go, I recommend some great resources. For instance, check out The Ultimate Guide to Debugging with Go for solid debugging advice, or if you’re diving into RPC, gRPC with Go is a must-watch. And if you’re interested in command-line interfaces, you can’t miss The Art of CLI in Golang.
Whether you’re a Go beginner or a more seasoned developer, mastering continue
can make your code cleaner and more efficient, especially when you’re dealing with large datasets or complex logic.
What is the continue Statement in Go?
So, what exactly does continue
do? In simple terms, the continue
statement skips the rest of the current loop cycle and jumps straight to the next one. If a certain condition is met, continue
will ignore any remaining code in that loop and go right to the next iteration. It’s like telling Go, “Skip this one; let’s move on.”
Here’s a quick example:
for i := 1; i <= 10; i++ {
if i%2 == 0 {
continue // Skip even numbers
}
fmt.Println(i)
}
In this snippet, continue
is used to skip even numbers, so only the odd numbers get printed. This trick might seem minor, but when used right, it can save time, keep your code tidy, and make your intentions crystal clear.
1. Using continue in Golang Loops
Getting comfortable with continue
is the first step to streamlining your loops. Here are some practical examples of using it to keep your code streamlined and efficient.
Skipping Specific Values
Picture a collection of numbers where your focus is solely on the positive ones that you want to work with. Instead of wrapping all your code in an if
statement to filter out negatives, you can just use continue
and skip over the negative values:
numbers := []int{3, -1, 4, -2, 5}
for _, num := range numbers {
if num < 0 {
continue // Skip negative numbers
}
fmt.Println(num) // Only prints positive numbers
}
Here, we’re skipping negative numbers in each iteration, which makes the loop logic simpler and more direct.
Filtering Data with continue
Another classic use of continue
is when you’re working with data but want to skip entries that don’t match certain criteria. Suppose you have a list of email addresses, and you only want the ones from a specific domain. By using continue
, you can avoid the ones that don’t match without cluttering your loop:
emails := []string{"user1@example.com", "user2@test.com", "user3@example.com"}
for _, email := range emails {
if !strings.Contains(email, "@example.com") {
continue // Skip non-matching domains
}
fmt.Println(email) // Only prints emails from example.com
}
Using continue
here makes it easy to zero in on only the emails you care about, and it keeps the code simple and easy to follow.
By the way, if you’re interested in advanced debugging tricks to help make your life easier when working with loops like these, The Ultimate Guide to Debugging with Go is packed with insights.
Improving Performance in Condition-Heavy Loops
Working with large datasets or multiple conditions? Extra checks can slow things down. By placing continue
statements carefully, you can skip irrelevant cases early in the loop, keeping things quick and efficient.
2. Common Use Cases for continue in Go
a. Skipping Unwanted Elements
One of the most practical ways to use continue
is to filter out unwanted elements, like null values, empty strings, or specific characters. For instance, let’s say you’re processing user data and want to skip over any entries that are empty:
users := []string{"Alice", "", "Bob", "Charlie", ""}
for _, user := range users {
if user == "" {
continue // Skip empty entries
}
fmt.Println(user)
}
Using continue
here makes it easy to avoid empty strings without cluttering the loop with extra if
statements. Even a small change can have a huge impact.
b. Handling Errors Without Breaking the Loop
Error handling can sometimes throw off an entire loop. But with continue
, you can skip over any errors you encounter, allowing the rest of the data to process as expected. Let’s say you’re working with a list of URLs, and some of them might be invalid:
urls := []string{"https://golang.org", "invalid-url", "https://example.com"}
for _, url := range urls {
if _, err := http.Get(url); err != nil {
fmt.Println("Skipping invalid URL:", url)
continue
}
fmt.Println("Processing URL:", url)
}
In this code, continue
lets us move past invalid URLs without crashing the entire loop, making it more resilient.
c. Keeping Code Readable in Complex Logic
When a loop’s logic gets complex, nested if
statements can make code hard to follow. Using continue
for early exits keeps the main logic in focus, reducing nesting and making everything easier to read.
3. Benefits of Simplifying Loop Logic with continue
Using continue
effectively brings several benefits to your Go code, especially in terms of readability and performance. Here’s why you’ll want to consider it:
-
Improving Code Readability: Code readability matters—especially if you’re working in a team, or you’ll be revisiting code later. Nested
if
statements can get messy, but withcontinue
, you can remove extra nesting, making your intentions clear. -
Boosting Efficiency: In loops involving large datasets, avoiding unnecessary operations makes a difference. Using
continue
to skip irrelevant cases helps your loop run faster. This can be especially important for real-time applications or data-heavy tasks. -
Smoother Error Handling: The
continue
statement is also handy for error handling, letting you bypass certain entries without halting everything. This is particularly useful when working with data streams or file operations where some issues are expected but shouldn’t disrupt the whole loop.
If you’re interested in learning more about handling complex command-line interfaces in Go, check out The Art of CLI in Golang for some excellent tips and tricks.
4. Best Practices and Tips for Using continue
If you’re ready to get the most out of continue
, here are some best practices:
-
Don’t Overdo It: While
continue
is great, using it too much can make your logic confusing. Use it sparingly, and only when it makes your code cleaner. For more complex cases, you might want to consider breaking out helper functions. -
Use Helper Functions: If you find yourself using
continue
often, consider making a helper function that returns atrue
orfalse
for conditions where you want to skip iterations. This modular approach keeps your code organized and reusable.
func shouldSkip(num int) bool {
return num < 0 || num > 100
}
numbers := []int{50, -1, 75, 200, 30}
for _, num := range numbers {
if shouldSkip(num) {
continue
}
fmt.Println(num)
}
- Comment Your Code: A quick comment explaining why you’re skipping an iteration goes a long way, especially for others reading your code.
Conclusion
The continue
statement is a simple yet powerful way to simplify loop logic in Golang. Using continue
effectively can make your code cleaner, easier to read, and faster. Whether you’re filtering data, handling errors, or just cutting down on nested conditions, continue
helps you focus on what’s important without unnecessary clutter.
If you’re interested in diving deeper, especially with debugging complex loops, check out The Ultimate Guide to Debugging with Go to hone your skills even further.
FAQs
-
Can I use
continue
in nested loops in Go?
Yes,continue
works in nested loops, but it only affects the nearest enclosing loop. For multi-level skipping, consider breaking things out into functions. -
How does
continue
differ frombreak
in Go?
continue
skips the current iteration, whilebreak
exits the loop completely. Usecontinue
when you want to skip specific cases without stopping everything. -
Can
continue
improve performance?
Yes, especially with large datasets. By bypassing unnecessary entries,continue
helps loops run faster. -
When should I avoid
continue
?
Ifcontinue
makes your code harder to read or understand, it’s best avoided. Use it only when it genuinely simplifies things. -
Can I debug
continue
statements?
Absolutely. Using print statements or a debugger can help you see what’s being skipped, so you can be sure your logic is working as intended.