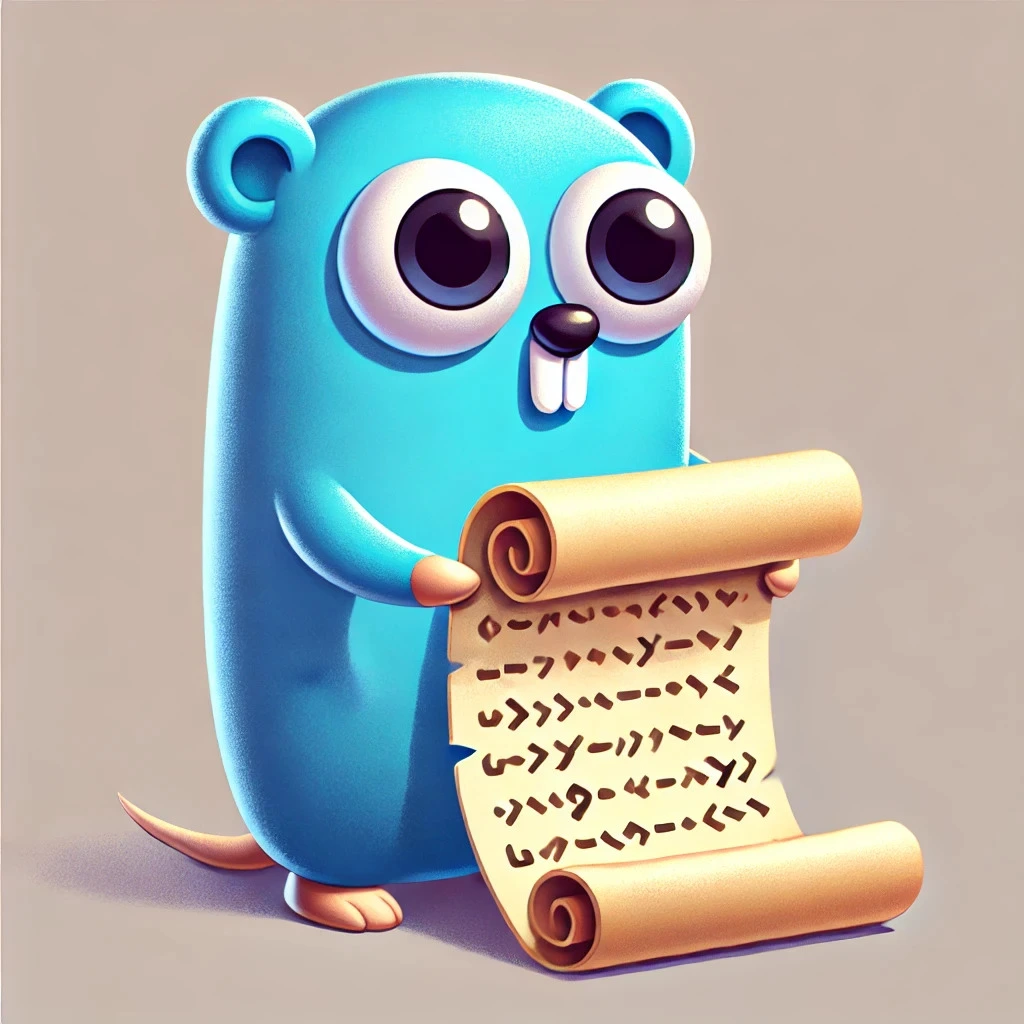
Mastering CSV Handling in Go: A Comprehensive Guide to the Golang CSV Reader
When working with data in Go, CSV (Comma-Separated Values) files are among the most popular formats for storing and exchanging information. Go’s rapid development and the new features introduced in recent versions enhance its capabilities for handling various data formats.
To stay updated, check out our free courses on what’s new in Go 1.22 and Go 1.23 in 23 Minutes. For those looking to deepen their Go expertise, especially using Goland, our free course on Mastering Go with GoLand is a must-watch.
In this guide, we will explore everything you need to know about the Golang CSV reader, from reading CSV files to writing and handling large datasets with ease. By the end of this post, you’ll be equipped with the knowledge to efficiently process CSV data using Go.
What is the Golang CSV Reader?
Go’s encoding/csv package provides a straightforward way to read and write CSV files. It comes with built-in methods for parsing rows and columns, customizing delimiters, and handling edge cases like quotes or empty fields.
Key features of the Golang CSV reader include:
- Simple and efficient API to read and write CSV files.
- Ability to handle large datasets efficiently, thanks to Go’s performance.
- Flexibility in handling different delimiters, custom headers, and more.
Why Use the Golang CSV Reader?
- Performance: Go is known for its speed and efficiency, making it ideal for processing large CSV files.
- Simplicity: The encoding/csv package provides an easy-to-use interface for reading and writing CSV data.
- Customization: You can customize CSV parsing behavior to suit different file formats and structures.
Setting Up Your Go Environment for CSV Parsing
To get started with reading and writing CSV files in Go, you need to set up your Go environment and ensure that the encoding/csv package is available.
go mod init csvreader
go get encoding/csv
With your environment set up, you’re ready to dive into CSV parsing.
How to Use the Golang CSV Reader: A Step-by-Step Tutorial
Reading a CSV File with Golang
Let’s start with the basics—reading a CSV file. Below is a simple example of how to use the Golang CSV reader to read a file:
package main
import (
"encoding/csv"
"fmt"
"os"
)
func main() {
file, err := os.Open("data.csv")
if err != nil {
fmt.Println("Error:", err)
return
}
defer file.Close()
reader := csv.NewReader(file)
records, err := reader.ReadAll()
if err != nil {
fmt.Println("Error:", err)
return
}
for _, record := range records {
fmt.Println(record)
}
}
This code reads all the records from a CSV file into a slice of slices and prints them. The ReadAll
method reads the entire file at once.
Handling Errors in Golang CSV Reader
Error handling is crucial when reading CSV files, especially when dealing with large files or inconsistent formats. For example, if the file doesn’t exist or if there are malformed records, the Golang CSV reader will return an error. You should at a minimum log it, and at a maximum terminate your program.
if err != nil {
fmt.Println("Error:", err)
return
}
Customizing the CSV Reader
The encoding/csv package allows you to customize the reader to handle different delimiters (not just commas) and quoting rules. For example, if your CSV file uses semicolons instead of commas:
reader.Comma = ';'
Processing Large CSV Files with Golang
For large CSV files, reading the entire file at once may not be ideal. Instead, you can process the file line by line using the Read()
method. This approach is memory-efficient and ideal for handling large datasets:
for {
record, err := reader.Read()
if err == io.EOF {
break
}
if err != nil {
fmt.Println("Error:", err)
continue
}
fmt.Println(record)
}
Writing to CSV Files in Go
Writing data to a CSV file is just as easy. Here’s how to write records to a CSV file using the Golang CSV writer:
file, err := os.Create("output.csv")
if err != nil {
log.Fatalln("Error creating file:", err)
}
defer file.Close()
writer := csv.NewWriter(file)
defer writer.Flush()
records := [][]string{
{"Name", "Age", "Location"},
{"Alice", "30", "New York"},
{"Bob", "25", "Los Angeles"},
}
for _, record := range records {
if err := writer.Write(record); err != nil {
log.Fatalln("Error writing record to file:", err)
}
}
This example writes a few rows of data into a CSV file using the Write()
method.
Best Practices for Working with the Golang CSV Reader
To get the most out of the Golang CSV reader, here are some best practices:
- Memory Management: Use
Read()
instead ofReadAll()
for large files to avoid loading the entire file into memory. - Error Handling: Always handle errors gracefully, especially when working with external data sources.
- Delimiter Customization: If your CSV files use different delimiters, ensure you customize the
Comma
attribute in the reader.
Common Mistakes and How to Avoid Them
- Mismatched Delimiters: Ensure the delimiter matches the one used in your CSV file.
- Handling Quotes: If your CSV contains quoted fields, ensure proper handling of quotes using the
LazyQuotes
option.
Wrapping Up
The Golang CSV reader is a powerful and easy-to-use tool for handling CSV files. Whether you’re working with large datasets or small configurations, Go’s encoding/csv package offers flexibility, performance, and simplicity. By following the best practices in this guide, you’ll be able to handle CSV data efficiently in your Go applications.
Ready to put the Golang CSV reader to work? Try reading and writing your own CSV files today!