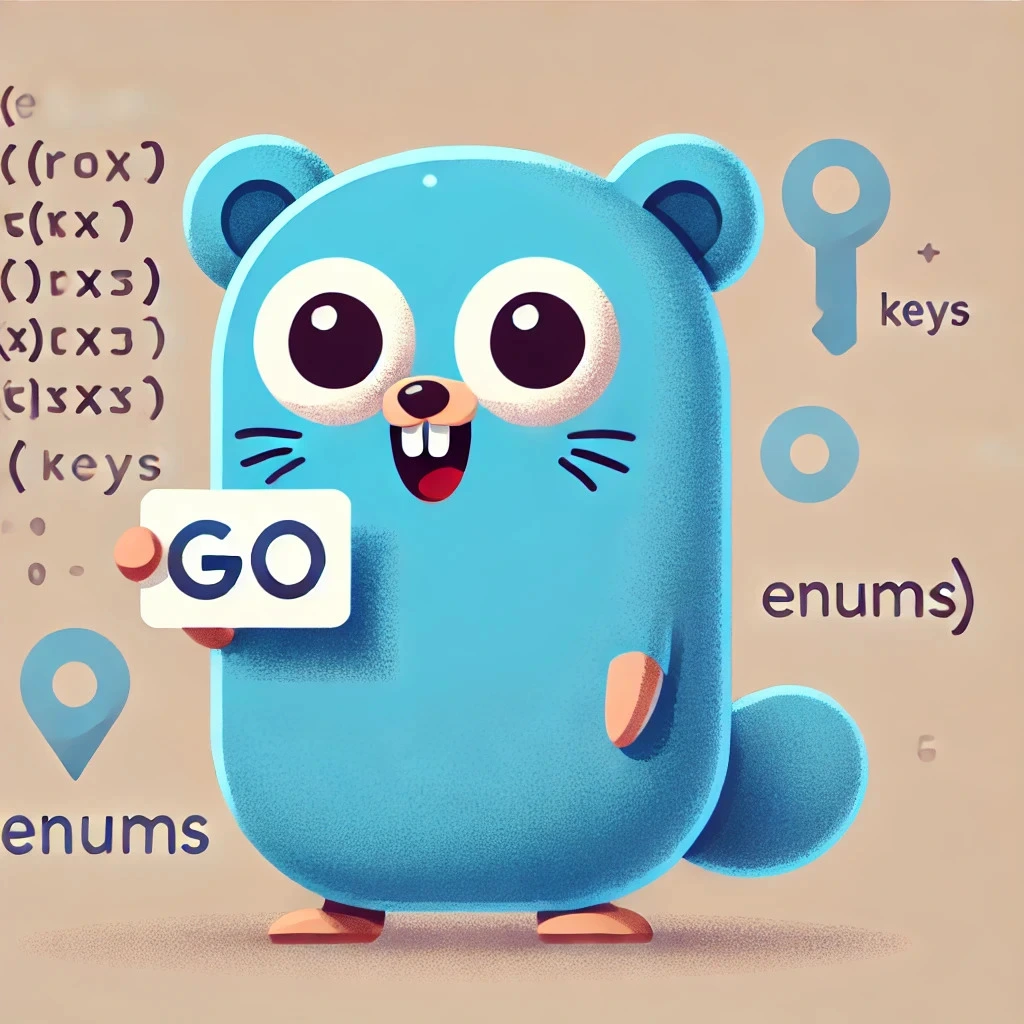
Understanding Enums in Golang
Enums (short for enumerations) are a useful feature in many programming languages, allowing developers to define a set of named constants that represent unique values. However, Golang (Go) doesn’t provide native support for enums like other languages such as C, Java, or Python. But don’t worry — you can still achieve similar functionality using Go’s types and constants. In this post, we’ll dive into Golang enums, how to define and use them.
What Is an Enum in Go?
Although Golang does not have an explicit enum keyword, we can create something like an enums using constants in combination with iota, a special Go identifier that simplifies the creation of incrementing constants. Enums in Go are typically used when you need a fixed set of values, such as status codes, types of entities, or categories of data. Here’s a quick example of what that looks like:
package main
import (
"fmt"
)
type Day int
const (
Sunday Day = iota
Monday
Tuesday
Wednesday
Thursday
Friday
Saturday
)
func main() {
fmt.Println(Sunday) // Output: 0
fmt.Println(Saturday) // Output: 6
}
Why Use Enums in Go?
Enums provide several benefits in your Go code:
-
Improved readability: Descriptive names for values help explain meaning.
-
Type safety: Ensures values stay within a known range.
-
Maintainability: Reduces the chance of invalid or undefined values being used.
What is iota?
ota is a built-in Go identifier used in const declarations. It starts at 0 and increments by 1 for each new constant, which is nice for creating enum-type things. Here’s a breakdown:
const (
A = iota // A == 0
B // B == 1
C // C == 2
)
If you need more control over the values, you can explicitly set the values at any point:
const (
Red = 1
Blue = iota + 1 // Blue == 2
Green // Green == 3
)
How do I Create String Representations of Enums?
You can do something like this:
package main
import "fmt"
type Day int
const (
Sunday Day = iota
Monday
Tuesday
Wednesday
Thursday
Friday
Saturday
)
func (d Day) String() string {
return [...]string{"Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"}[d]
}
func main() {
fmt.Println(Sunday) // Output: Sunday
fmt.Println(Saturday) // Output: Saturday
}
Here’s how this works.
-
We define a String() method for the Day type.
-
Inside String(), we return a string from an array of names based on the index of the enum (Sunday = 0, Monday = 1, etc.).
Now, whenever you print a Day value, it will print the corresponding string instead of the integer.
What are the Best Practises for Enums?
- Use Custom Types: Always define a custom type for your enums instead of using plain int or string. This provides type safety:
type Status int
- Provide String Representation: Implement the String() method for your enum type to make debugging and logging easier.
- Use iota: Use iota when you need sequential constants.
- Use 0 as a default value: Always handle invalid or unspecified enum values. To do this, we recommend using the 0 value as unspecified as follows:
const (
Unknown PhoneType = iota
Mobile
Home
Work
)