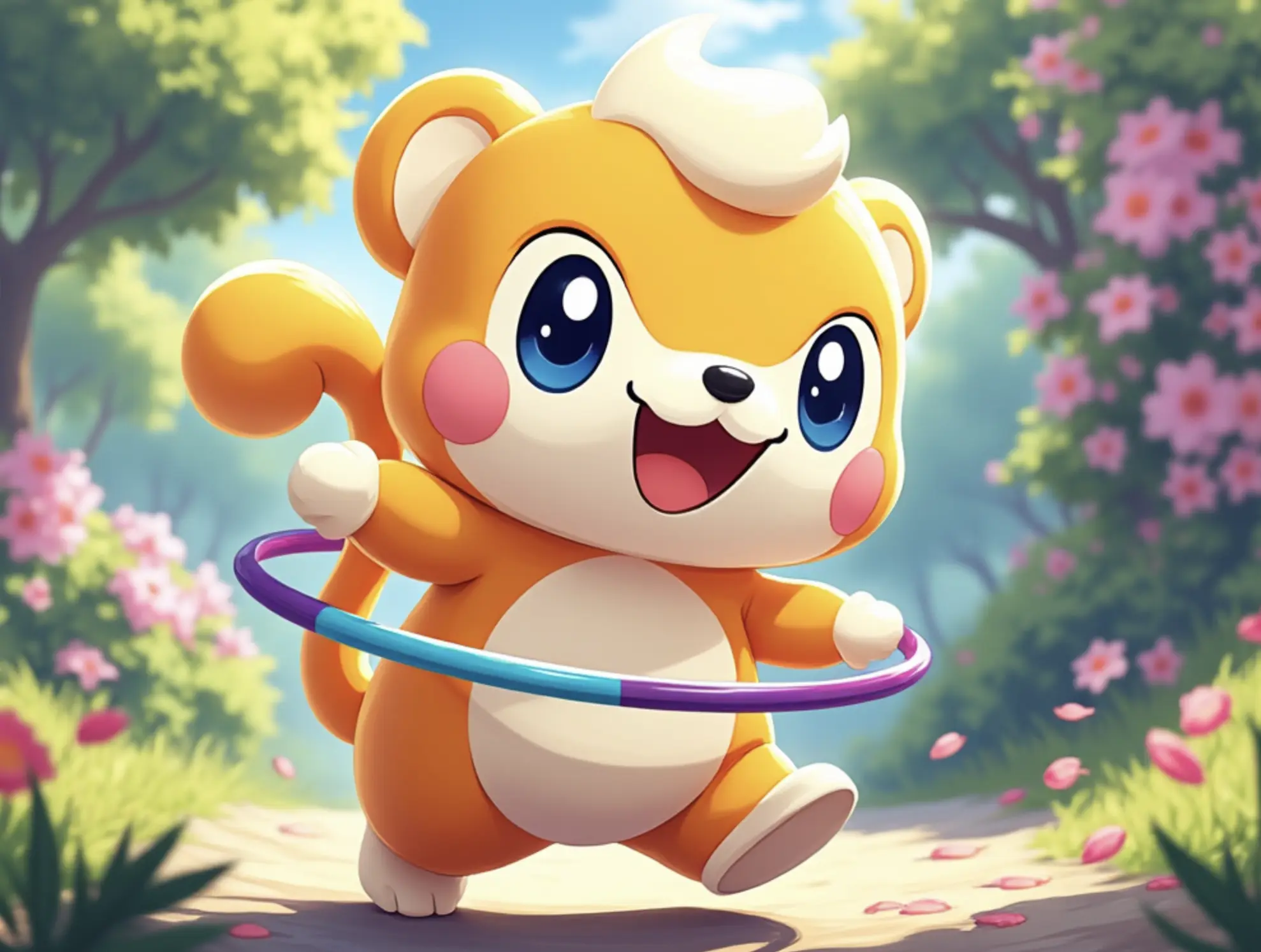
Everything You Need to Know About for Loops in Go
In Golang, one of the most useful programming constructs you’ll use is the for loop. Unlike many other languages that offer a variety of loop structures, Go simplifies things by providing only one looping construct: the for loop. Despite this simplicity, Go’s for loop is versatile and can do everything you need; even if it not as succinct as some other languages.
This guide will walk you through everything you need to know about Golang for loops—from basic syntax to (slightly more) advanced patterns. Whether you’re a beginner or looking to refine your knowledge, this comprehensive breakdown will tell you everything you need to know about the for loop in Go.
The Basic Syntax of Golang For Loops
A standard for loop in Go looks like this:
for initialization; condition; post {
// Your logic here
}
Let’s break that down.
-
Initialization: This part runs once before the loop starts, typically setting a loop counter.
-
Condition: The loop runs as long as this condition evaluates to true.
-
Post: This executes after each iteration, usually updating the loop counter.
Example: Simple Counting Loop
for i := 0; i < 5; i++ {
fmt.Println(i)
}
would output:
0
1
2
3
4
Different Patterns of For Loops in Golang
Go’s for loop can be used in a variety of ways. Below are some of the most common patterns we see.
Infinite Loops
By leaving out the condition in a for loop, you can create an infinite loop that runs until it’s stopped.
for {
fmt.Println("This will loop forever and ever")
}
To break out, you can use a break:
for {
fmt.Println("Looping...")
if someCondition {
break
}
}
A “while” Loop
Go doesn’t officially have a while loop, but we can make one:
x := 0
for x < 5 {
fmt.Println(x)
x++
}
Ranges in for Loops
Golang’s range keyword is used to iterate over arrays, slices, maps, strings, and channels. When combined with for, it can be really useful:
Ranging Over a Slice
numbers := []int{1, 2, 3, 4, 5}
for index, value := range numbers {
fmt.Printf("Index: %d, Value: %dn", index, value)
}
outputs:
Index: 0, Value: 1
Index: 1, Value: 2
Index: 2, Value: 3
Index: 3, Value: 4
Index: 4, Value: 5
Ranging Over a Map
ages := map[string]int{"Matt": 25, "John": 30, "Chris": 35}
for name, age := range ages {
fmt.Printf("%s is %d years oldn", name, age)
}
outputs:
Matt is 25 years old
John is 30 years old
Chris is 35 years old
(although not necessarily in that order, Go does not guarantee order in a map when iterating like this).
Ranging Over a String
str := "Golang"
for index, char := range str {
fmt.Printf("Index: %d, Character: %cn", index, char)
}
outputs:
Index: 0, Character: G
Index: 1, Character: o
Index: 2, Character: l
Index: 3, Character: a
Index: 4, Character: n
Index: 5, Character: g
Nested for Loops
Sometimes, you need to perform more complex operations that require nested loops—loops inside other loops. Nested for loops are slow to execute as the amount of elements you are looping over increases so it’s always worth trying to avoid them where possible.
for i := 1; i <= 3; i++ {
for j := 1; j <= 2; j++ {
fmt.Printf("i = %d, j = %dn", i, j)
}
}
outputs:
i = 1, j = 1
i = 1, j = 2
i = 2, j = 1
i = 2, j = 2
i = 3, j = 1
i = 3, j = 2
Control Flow - Using Break and Continue
Go offers control flow statements like break and continue to manage the flow of loops.
-
break: Exits the loop entirely.
-
continue: Skips the rest of the loop’s current iteration and moves to the next.
Here’s an example breaking a loop:
for i := 0; i < 10; i++ {
if i == 5 {
break
}
fmt.Println(i)
}
outputs:
0
1
2
3
4
and for continue:
for i := 0; i < 10; i++ {
if i%2 == 0 {
continue
}
fmt.Println(i)
}
outputs:
1
3
5
7
9
Best Practices for Using For Loops in Golang
-
Use range when possible: Using range makes your code more idiomatic and easier to read.
-
Avoid infinite loops unless necessary: Infinite loops can cause unintended behavior if not carefully controlled. Always ensure there’s a clear way to stop them!
-
Minimize nested loops: While nested loops are sometimes necessary, try to keep them to a minimum as they can lead to performance issues.
-
Break early when possible: If a loop condition is met early, break to exit the loop as soon as possible to ensure your program is as fast as possible and doesn’t have unintended side effects.
Wrapping Up
You really do know everything you need to know about the for loop now! You can experiment with the examples above in the Go Playground, and consider taking our free course that will teach you everything you need to know about the latest Go release too.