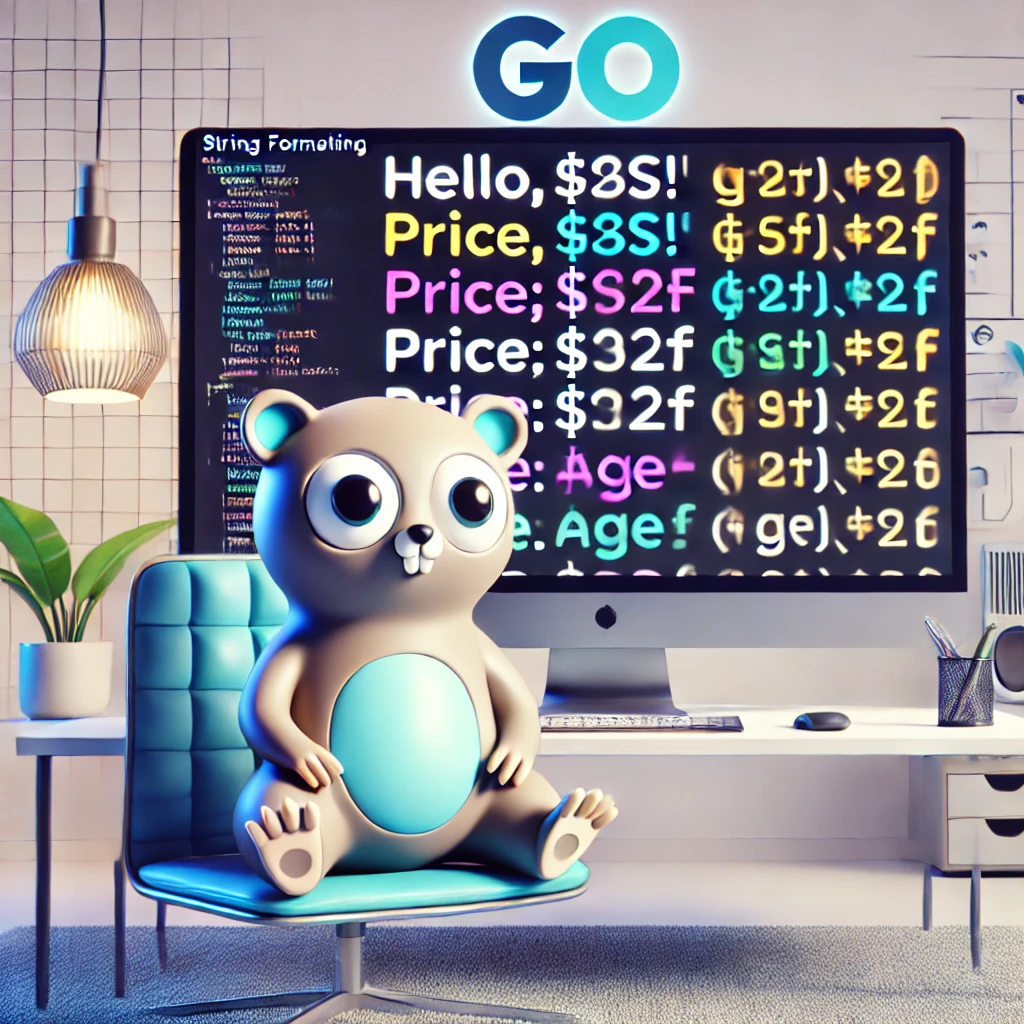
Mastering String formatting in Go
Formatting strings is a fundamental aspect of programming, and Go provides an easy way to do this via the fmt
package. Whether you’re constructing user-facing messages, logging debug information, or building complex output for
APIs, understanding string formatting in Go is crucial.
In this blog post, we’ll explore the essentials of string formatting in Go, covering practical use cases, syntax, and best practices.
Why Use String Formatting?
String formatting allows you to:
- Concatenate strings and variables cleanly without manual concatenation.
- Control the layout and precision of numbers and strings.
- Format output for human readability or machine parsing.
For an in-depth overview of the fmt
package, visit the official fmt package documentation.
Basics of fmt.Sprintf
The fmt.Sprintf
function is the workhorse for string formatting in Go. It takes a format string and a list of
arguments, returning a formatted string.
Example:
package main
import "fmt"
func main() {
name := "Alice"
age := 30
formatted := fmt.Sprintf("My name is %s, and I am %d years old.", name, age)
fmt.Println(formatted)
}
Output:
My name is Alice, and I am 30 years old.
In the format string:
%s
is a placeholder for strings.%d
is a placeholder for integers.
Common Format Specifiers
Specifier | Type | Example |
---|---|---|
%s | String | "Alice" |
%d | Integer (base 10) | 30 |
%f | Float (default precision) | 3.141593 |
%.2f | Float (2 decimal places) | 3.14 |
%t | Boolean | true |
%v | Any value (default) | Alice , 30 , true |
%#v | Go-syntax representation | "Alice" , 30 |
%T | Type of the value | string , int |
%% | Literal percent sign | % |
Example:
package main
import "fmt"
func main() {
price := 123.456
fmt.Printf("Price: $%.2f", price) // Formats with 2 decimal places
}
Output:
Price: $123.46
You can find more details about these specifiers in the fmt package documentation.
Formatting Complex Data Structures
You can use %v
to format structs, slices, or maps in a default manner:
package main
import "fmt"
type Person struct {
Name string
Age int
}
func main() {
person := Person{Name: "Alice", Age: 30}
fmt.Printf("Person: %v\n", person)
fmt.Printf("Detailed: %+v\n", person)
}
Output:
Person: {Alice 30}
Detailed: {Name:Alice Age:30}
%+v
includes field names in the output.%#v
outputs a Go-syntax representation.
Right Align, Width, and Padding
You can control the alignment and width of your formatted strings:
Specifier | Description |
---|---|
%10s | Right-align, 10 spaces |
%-10s | Left-align, 10 spaces |
%010d | Pad with zeros |
Example:
package main
import "fmt"
func main() {
fmt.Printf("%10s\n", "Go")
fmt.Printf("%-10s!\n", "Go")
fmt.Printf("%010d\n", 42)
}
Output:
Go
Go !
0000000042
Best Practices
-
Use
fmt.Sprintf
for Reusability: Avoid mixing logic and formatting infmt.Printf
. Usefmt.Sprintf
for reusable strings. -
Minimize Hardcoding: Use format specifiers to dynamically include variables in your strings.
-
Leverage
%v
for Debugging:%v
is a quick way to inspect values during development. -
Precision Matters: Use specific specifiers like
%.2f
for floats to avoid unintentional inaccuracies.
Advanced: Custom Formatting with Stringer
Go allows custom types to define their string representation by implementing the fmt.Stringer
interface. Learn more
about this interface in the fmt.Stringer documentation.
Example:
package main
import "fmt"
type Currency struct {
Code string
Value float64
}
func (c Currency) String() string {
return fmt.Sprintf("%s %.2f", c.Code, c.Value)
}
func main() {
usd := Currency{Code: "USD", Value: 123.456}
fmt.Println(usd)
}
Output:
USD 123.46
Conclusion
Formatting strings in Go is both powerful and flexible, enabling you to handle everything from basic text substitutions
to advanced custom formatting. The fmt
package is an indispensable tool for creating clean, readable, and maintainable
code.
To dive deeper, explore the official fmt documentation, and practice these techniques to master string formatting in Go.
For more insights on Go programming, you might find this article on Working With Time in Golang helpful.
Happy coding!