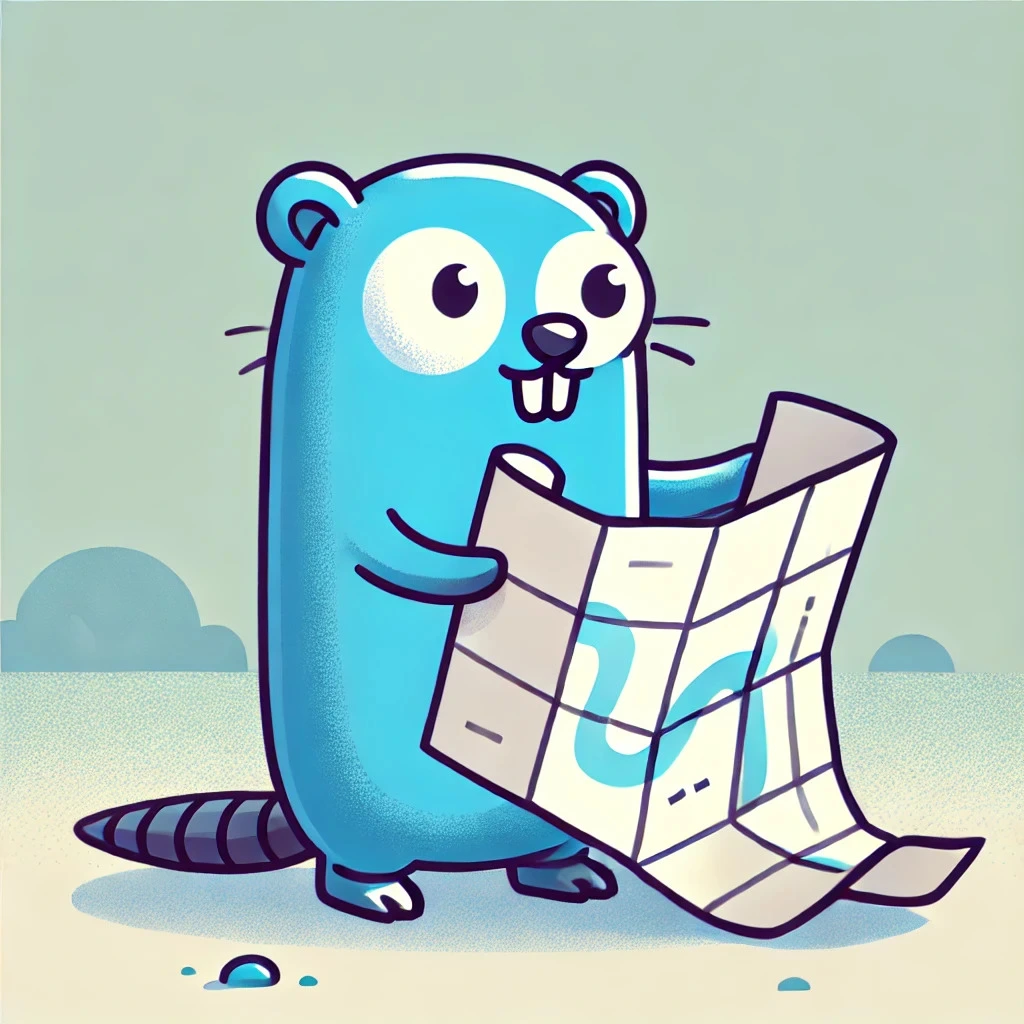
Let's Map This Out
one of the most powerful and flexible data structures is the map. Whether you’re a experienced developer or new to Go, understanding how to effectively use maps can enhance the efficiency and clarity of your code.
In this post, we’ll dive deep into Golang maps, exploring what they are, how they work, and how you can use them to solve real-world programming challenges.
What’s a Map?
In Go, a map is an unordered collection of key-value pairs. You can think of it as a dictionary or hash table if you’re familiar with those from other languages, where each key is unique and maps to a specific value. Maps allow for efficient data retrieval based on a key, making them highly useful for various applications such as caching, counting occurrences, and more.
Here’s the basic syntax of a map in Go:
map[KeyType]ValueType
- KeyType: The type of the key. It must be comparable (e.g., integers, strings, structs).
- ValueType: The type of the value stored in the map. This can be pretty much anything.
Creating and Initializing a Golang Map
To create a map, you can either use the built-in make
function or declare and initialize it in one step, like this:
m := make(map[string]int)
m["age"] = 25
m["score"] = 100
fmt.Println(m) // Output: map[age:25 score:100]
In this example, we:
- Created a map
m
where the keys are of typestring
, and the values are of typeint
. - Added two key-value pairs to the map.
And here’s an example of the other way.
m := map[string]int{
"peach": 10,
"banana": 30,
}
fmt.Println(m) // Output: map[peach:10 banana:30]
Common Map Operations
Adding Elements
Adding elements to a map is straightforward—simply assign a value to a key:
m["name"] = "Matt"
Accessing Elements
You can access elements by specifying the key. If the key exists, Go will return the corresponding value; otherwise, it returns the zero value for the map’s value type:
name := m["name"]
fmt.Println(name) // Output: Matt
Checking if a Key Exists
You can check if a key exists as follows:
value, exists := m["key"]
if exists {
fmt.Println("Key exists:", value)
} else {
fmt.Println("Key does not exist")
}
Deleting an Element
To delete a key-value pair from a map, use the delete
function:
delete(m, "age")
Looping through a map
You can loop through a map using the range
keyword:
for key, value := range m {
fmt.Printf("Key: %s, Value: %dn", key, value)
}
Practical Use Cases of Golang Maps
Let’s look at some real-world examples of where maps can be useful.
Counting occurrences of things
words := []string{"apple", "banana", "apple", "orange"}
countMap := make(map[string]int)
for _, word := range words {
countMap[word]++
}
fmt.Println(countMap) // Output: map[apple:2 banana:1 orange:1]
Caching
Maps are often used for caching expensive calculations or API results.
cache := make(map[string]int)
cache["x*x+3"] = 12
cache["x*x^9"] = 47
Grouping
If you want to group data by a certain property, maps allow for quick organization.
people := []struct {
Name string
City string
}{
{"John", "New York"},
{"Alice", "Los Angeles"},
{"Bob", "New York"},
}
cityGroup := make(map[string][]string)
for _, person := range people {
cityGroup[person.City] = append(cityGroup[person.City], person.Name)
}
fmt.Println(cityGroup)
// Output: map[Los Angeles:[Alice] New York:[John Bob]]
Some Important Things to Remember about Golang Maps
While maps are incredibly useful, there are a few things to keep in mind:
-
Maps Are Not Thread-Safe: If you’re accessing a map from multiple goroutines, use sync mechanisms like
sync.Mutex
orsync.RWMutex
to prevent race conditions. -
Nil Maps: A nil map is uninitialized and will cause a panic if you try to add elements to it. Always initialize a map before use.
-
Unordered Nature: Maps in Go are unordered, meaning that iterating over a map does not guarantee the order of keys. For example, the example we used before:
m := map[string]int{
"peach": 10,
"banana": 30,
}
fmt.Println(m)
could also output:
// Output: map[banana:30 peach:10]
so don’t depend on ordering!
Wrapping up
Knowing about maps and knowing when to use them will make you a better Go developer. The caching use case is a popular one if you are looking for opportunities to speed up your code.
If you found this blog useful, here are some others from the byteSizeGo collection you might enjoy: