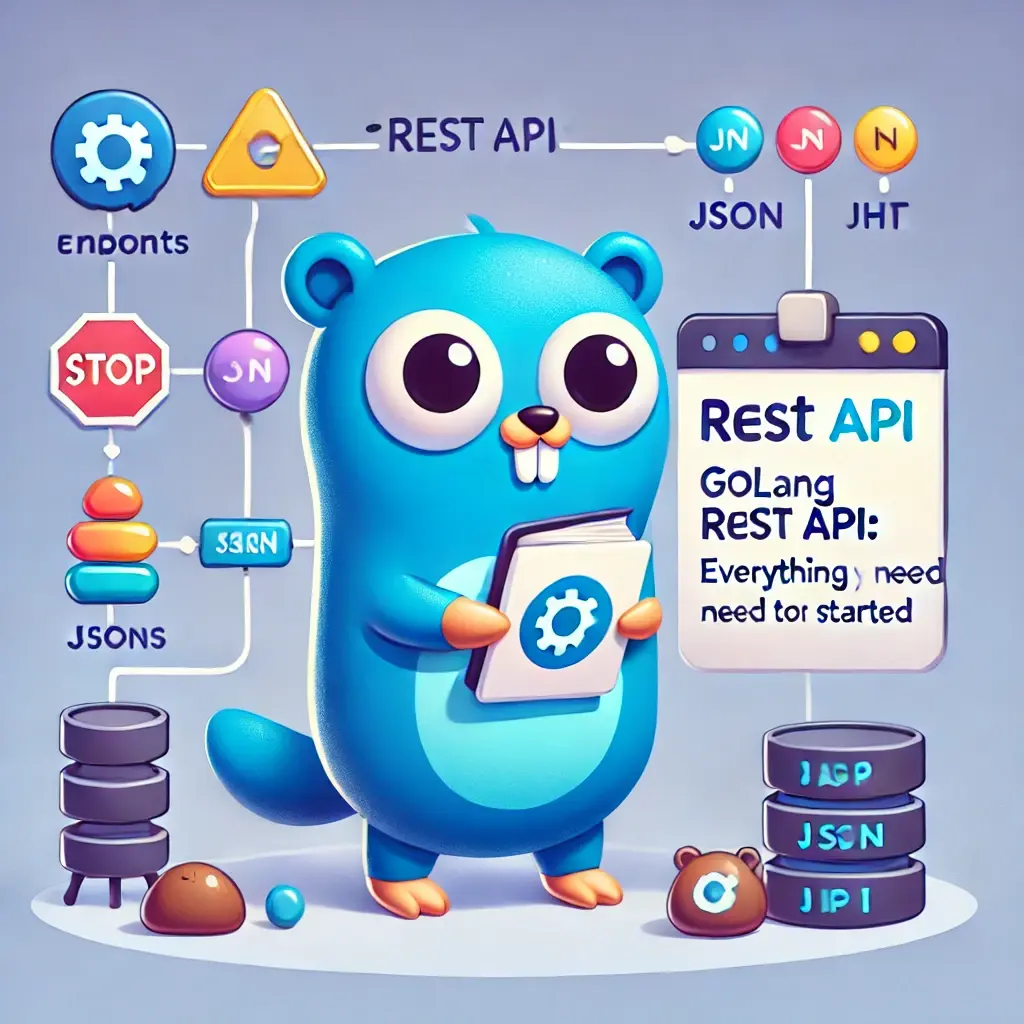
Golang REST API: Everything You Need to Get Started
Creating a REST API in Golang is an enriching experience that combines Go’s exceptional performance and simplicity with the principles of RESTful architecture. In this comprehensive guide, we’ll explore the essential aspects of building a REST API using Golang, including setting up your development environment, constructing your API, and ensuring its well-documented and thoroughly tested.
Understanding REST and Its Principles
What is REST?
Representational State Transfer (REST) is an architectural approach for creating networked applications. It relies on stateless communication and employs standard HTTP methods such as GET, POST, PUT, and DELETE to interact with resources. In REST, resources are identified using URIs (Uniform Resource Identifiers) and can be represented in various formats, most commonly JSON or XML.
Key Principles of RESTful APIs
RESTful APIs are built on several core principles:
-
Statelessness: Every API request from a client includes all the necessary information to handle the request. The server does not retain client context across requests.
-
Client-Server Architecture: The client and server operate independently, promoting separation of concerns and a modular approach to application design.
-
Resource Identification: Resources are identified through URIs, allowing clients to access and manipulate them using standard HTTP methods.
-
Uniform Interface: A uniform interface simplifies and decouples the architecture, facilitating interaction between clients and servers.
-
Representation of Resources: Resources can be represented in various formats, with JSON being the most widely used in modern APIs.
Advantages of Using REST APIs
REST APIs provide numerous benefits:
-
Scalability: The stateless nature allows for scalability, as each request is independent and can be distributed across multiple servers.
-
Flexibility: Clients can interact with resources in various ways, accommodating diverse applications.
-
Ease of Use: REST APIs are typically easier to understand and use, making them popular among developers.
Setting Up Your Golang Environment
Installing Go and Setting Up Your Workspace
To get started with Golang, you need to install the Go programming language. Here’s how to do it:
-
Download and Install Go: Visit the Go official website to download the installer for your operating system. Follow the installation instructions carefully.
-
Set Up Your Workspace: Create a directory for your Go projects. It’s common to set your workspace under
~/go
(Linux/Mac) orC:Go
(Windows). Ensure your GOPATH is set correctly by adding the following to your shell profile:
export GOPATH=$HOME/go
export PATH=$PATH:$GOPATH/bin
- Verify the Installation: Open your terminal and run
go version
to confirm that Go is installed successfully.
Understanding Go Modules
Go modules are essential for dependency management, allowing you to manage the versions of your dependencies easily. To add a new module, go to your project directory and execute:
go mod init your-module-name
This command will create a go.mod
file that tracks your module’s dependencies.
Recommended Tools and IDEs for Golang Development
While any text editor can be used to write Go code, these IDEs provide excellent support for Golang development:
- Visual Studio Code: With the Go extension, VS Code offers features like IntelliSense and debugging.
- GoLand: A commercial IDE specifically designed for Go development, featuring robust functionalities.
- Vim/Neovim: For those who prefer a lightweight option, Vim with the Go plugin is highly effective.
Building Your First REST API in Golang
Creating a Simple API with net/http
To build a basic REST API in Golang, we’ll use the built-in net/http
package. Here’s how to create a simple API:
- Create a New File: Start by creating a file named
main.go
and add the following code:
package main
import (
"encoding/json"
"net/http"
)
type Item struct {
ID string `json:"id"`
Name string `json:"name"`
}
var items []Item
func getItems(w http.ResponseWriter, r *http.Request) {
w.Header().Set("Content-Type", "application/json")
json.NewEncoder(w).Encode(items)
}
func main() {
http.HandleFunc("/items", getItems)
http.ListenAndServe(":8080", nil)
}
Open your terminal, navigate to your project directory, and execute:
go run main.go
Your API will be accessible at http://localhost:8080/items.
Structuring Your Project
As your project expands, it’s crucial to organize your code effectively. A recommended structure for a simple project might look like this:
/myapi
├── main.go
├── routes
│ └── routes.go
├── models
│ └── item.go
├── handlers
│ └── itemHandler.go
Handling JSON Requests and Responses
To handle incoming JSON requests, you can create a POST endpoint. Modify your main.go
as follows:
package main
import (
"encoding/json"
"net/http"
)
func createItem(w http.ResponseWriter, r *http.Request) {
var newItem Item
json.NewDecoder(r.Body).Decode(&newItem)
items = append(items, newItem)
w.WriteHeader(http.StatusCreated)
json.NewEncoder(w).Encode(newItem)
}
func main() {
http.HandleFunc("/items", getItems)
http.HandleFunc("/items", createItem)
http.ListenAndServe(":8080", nil)
}
Now you can send a POST request to /items
with a JSON body to add new items.
Testing and Documentation for Your API
Writing Unit Tests for Your API
Testing is crucial for ensuring your API functions as intended. You can use Go’s built-in testing package. Create a new file named main_test.go
:
package main
import (
"net/http"
"net/http/httptest"
"testing"
)
func TestGetItems(t *testing.T) {
req, err := http.NewRequest("GET", "/items", nil)
if err != nil {
t.Fatal(err)
}
rr := httptest.NewRecorder()
handler := http.HandlerFunc(getItems)
handler.ServeHTTP(rr, req)
if status := rr.Code; status != http.StatusOK {
t.Errorf("handler returned wrong status code: got %v want %v",
status, http.StatusOK)
}
}
Run your tests with:
go test
Using Swagger for API Documentation
API documentation is essential for user adoption. Swagger is an essential resource for simplifying the registration process of RESTful APIs. You can use the swaggo/swag package for Go:
- Install swag:
go get -u github.com/swaggo/swag/cmd/swag
- Initialize Swagger: Run swag init in your project directory to generate a docs folder containing the documentation.
- Add Swagger Comments: Annotate your handlers with comments that Swagger understands. For example:
// @Summary Get all items
// @Description get items
// @Produce json
// @Success 200 {array} Item
// @Router /items [get]
- Serve Swagger UI: Integrate Swagger UI in your application to visualize the API documentation.
Best Practices for API Testing
- Use Mock Servers: For integration testing, consider using mock servers to simulate API responses.
- Automate Testing: Integrate your tests with CI/CD pipelines to automate testing processes.
- Check for Edge Cases: Ensure your tests cover edge cases and error handling.
FAQs
What is the best way to handle errors in a Golang REST API?
Handling errors effectively is crucial for a positive user experience. Create a custom error response structure and use it consistently throughout your API. Ensure that each handler checks for errors and returns meaningful messages.
How can I secure my Golang REST API?
Securing your API can involve several strategies:
- Authentication: Implement JWT (JSON Web Tokens) or OAuth for user authentication.
- HTTPS: Use HTTPS to encrypt data in transit.
- Rate Limiting: Safeguard against misuse by restricting the number of requests from an individual client.
What are the performance considerations for Golang REST APIs?
Go is renowned for its performance, but consider these aspects:
- Connection Management: Use connection pooling for database connections.
- Concurrency: Leverage Go’s goroutines for handling multiple requests efficiently.
- Caching: Implement caching mechanisms for frequently accessed data.
How do I deploy a Golang REST API?
You can deploy your Golang API in various ways:
- Docker: Containerize your application using Docker for easy deployment.
- Cloud Services: Use platforms like AWS, Google Cloud, or Heroku.
- Traditional Hosting: Deploy on a VPS or dedicated server.
What libraries are recommended for building REST APIs in Golang?
Several libraries can enhance your REST API development in Golang:
- Gorilla Mux: A powerful URL router and dispatcher.
- Gin: A lightweight and fast HTTP web framework.
- Echo: Another high-performance framework with a rich set of features
Conclusion
Building a REST API in Golang is not only straightforward but also incredibly rewarding. By grasping the principles of REST, setting up your environment, and adhering to best practices for development, testing, and documentation, you can create a robust and scalable API. With the right tools and libraries, you’ll be equipped to handle various use cases and deliver outstanding performance. Whether you’re new to Go or looking to sharpen your skills, this guide provides a solid foundation for your API development journey.
If you enjoyed this blog, be sure to subscribe to our newsletter below, and check out our other content!