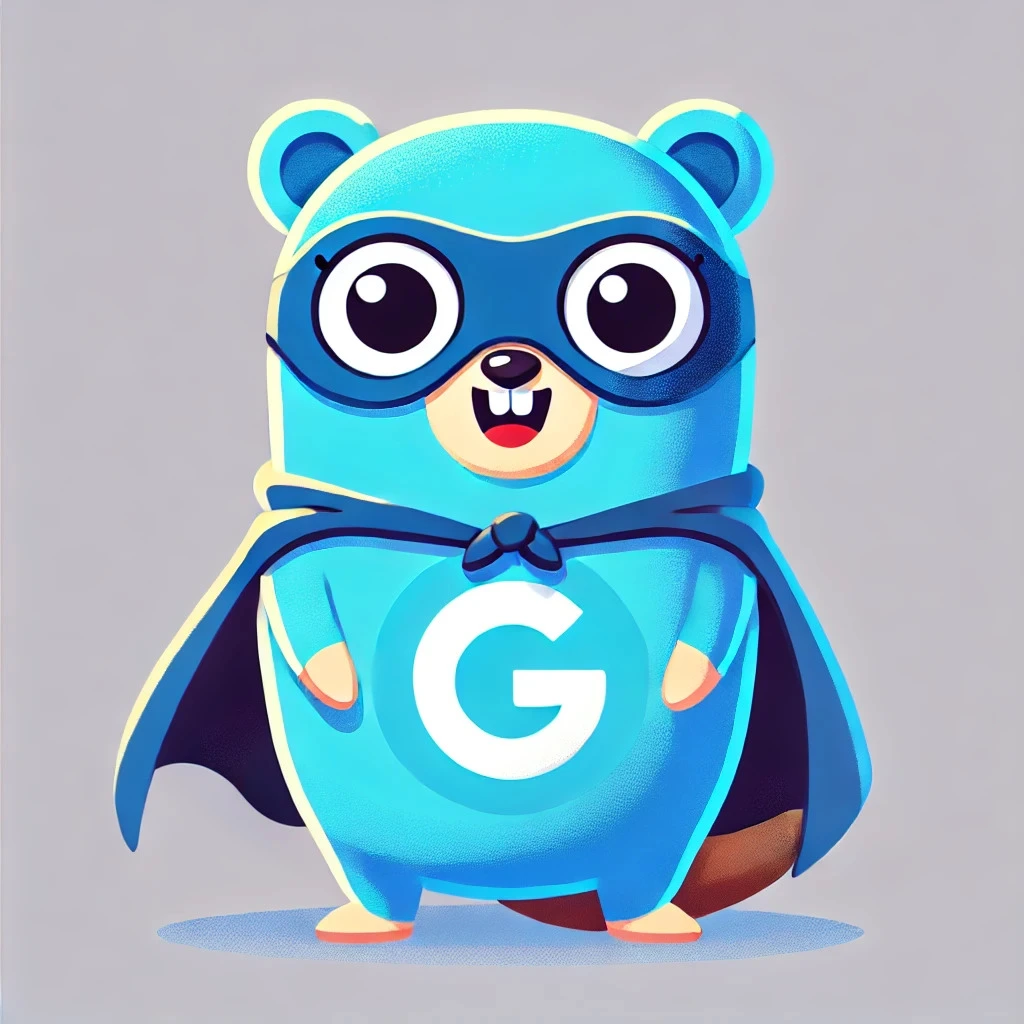
Golang Type Alias: Simplifying Code and Enhancing Readability
If you’re a Go (Golang) developer, you may have encountered the term “type alias.” While it’s not as frequently discussed as other Go features like interfaces, concurrency, or goroutines, type aliasing is a highly valuable tool in your Go programming skill set. It can significantly enhance your code’s readability and maintainability, which is especially important in larger, more complex projects. In this comprehensive guide, we’ll break down everything you need to know about Golang type aliases, including their definition, creation, and best use cases.
Introduction
This guide will also reference other essential Golang resources, such as gRPC with Go, the Art of CLI in Golang, and the Ultimate Guide to Debugging with Go, to help you expand your understanding of Golang and improve your skills.
What is a Type Alias in Golang?
In Go, a type alias is essentially an alternative name for an existing type. Unlike a custom type definition, a type alias doesn’t create a new type. Instead, it allows you to use an existing type under a different name. This can be incredibly useful when you need to refactor your code or when you want to improve the clarity of your program by giving descriptive names to certain types.
Here’s the key thing to understand: a type alias and the original type it represents are completely interchangeable. The Go compiler treats them as the same, meaning you can use them in any context where the original type would normally be used.
Basic Syntax of Type Alias in Go
To create a type alias in Go, you can use the following syntax:
type MyString = string
In this example, MyString
becomes an alias for the built-in string
type. This means that MyString
can be used anywhere a string
is expected. The two are treated identically by the Go compiler. No new type is created, but this alias can make your code much more readable.
Golang Type Alias vs. Custom Types
It’s crucial to differentiate between a type alias and a custom type in Go. While a type alias refers to an existing type, a custom type creates a completely new one. This distinction has important implications for how you use each in your programs.
Custom Types in Go
A custom type in Go defines a new, distinct type that is separate from the type it was derived from. This new type will not be compatible with the original unless explicit conversion is performed.
For example:
type MyString string
Here, MyString
is a custom type that is distinct from the built-in string
type. You cannot assign a value of type string
directly to a variable of type MyString
without performing explicit conversion.
Type Aliases in Go
In contrast, a type alias is simply another name for an existing type:
type MyAlias = string
With this alias, MyAlias
can be used interchangeably with string
. The Go compiler treats them as exactly the same type, so no conversion is necessary.
Key Differences
- Custom Types create a new type, which is distinct from the original and requires explicit conversion.
- Type Aliases are just alternative names for an existing type, with no distinction between them and the original type.
Benefits of Using Type Aliases in Go
So why should you use type aliases in Go? There are several practical advantages:
1. Improved Code Readability
One of the most significant benefits of type aliases is that they make your code more descriptive. For example, instead of using a generic type like string
, you can define an alias that gives more context to the purpose of that string:
type UserID = string
In this case, UserID
is an alias for string
, but it provides additional meaning to anyone reading the code, indicating that this particular string is being used to represent a user’s ID. This can greatly improve code clarity, especially in larger projects.
2. Easier Refactoring
In large codebases, refactoring can be a time-consuming and error-prone task. Type aliases can make this process much smoother. For example, if you decide to change the underlying type of a variable (e.g., from string
to int
), you can simply modify the type alias in one place, rather than updating every instance of the type throughout your codebase. This can help prevent bugs and save time.
3. Simplifying Complex Types
Go’s type system is powerful but can also become complex, especially when working with types like structs, interfaces, or third-party libraries. Type aliases can help simplify these types, making your code easier to read and maintain.
For example, working with complex types like *http.Response
can be tricky. A type alias can help clean up your code:
type HTTPResponse = *http.Response
This alias makes the code more readable without sacrificing functionality.
When to Use Type Aliases in Golang
Type aliases are a useful tool, but they should be used thoughtfully. Here are some common scenarios where type aliases can be particularly beneficial:
1. Interfacing with Third-Party Libraries
Third-party libraries often use complex or non-intuitive type names. In these cases, you can create a type alias to make the types easier to work with:
type JSONResponse = map[string]interface{}
By defining an alias like JSONResponse
, you can make your code more descriptive and easier to understand.
2. Enhancing Domain-Specific Code Readability
If your project includes domain-specific models or business logic, type aliases can enhance readability. For example:
type OrderID = string
type PaymentStatus = string
const (
Pending PaymentStatus = "pending"
Completed PaymentStatus = "completed"
Failed PaymentStatus = "failed"
)
By using type aliases like OrderID
and PaymentStatus
, you make it clear that these strings represent specific domain concepts rather than generic values.
3. Simplifying Generic Types (Go 1.18+)
Go 1.18 introduced generics, which allow functions to work with any data type. Type aliases can simplify the usage of generics in function signatures:
type List[T any] = []T
func printList[T any](list List[T]) {
for _, item := range list {
fmt.Println(item)
}
}
Here, List[T]
is a generic type alias for a slice of any type T
. This makes working with generics more intuitive and readable.
Best Practices for Using Golang Type Aliases
To make the most of type aliases in Go, follow these best practices:
- Use Descriptive Names: Your type aliases should be clear and meaningful. For example,
UserID
,OrderID
, orPaymentStatus
clearly indicate the purpose of the alias, improving the readability of your code. - Avoid Overuse: Don’t create type aliases for every type in your project. Reserve them for cases where they simplify complex types or provide a clear readability benefit.
- Maintain Consistency: Once you introduce a type alias like
UserID
, use it consistently across your codebase to avoid confusion. - Document When Necessary: If a type alias is not self-explanatory, consider adding a comment or documentation to explain its purpose. This is particularly helpful in large teams or open-source projects.
Example Use Cases for Golang Type Aliases
Let’s explore a few real-world use cases where type aliases can make your Go code more efficient and readable:
1. Simplifying HTTP Handlers
In web applications, you often work extensively with HTTP responses. A type alias can help make your code cleaner:
type JSONResponse = map[string]interface{}
func sendJSONResponse(w http.ResponseWriter, response JSONResponse) {
json.NewEncoder(w).Encode(response)
}
This alias makes it clear that you’re dealing with a JSON response, improving the readability of the code.
2. Working with Complex Structs
When working with complex structs in large projects, type aliases can help make function signatures and data handling cleaner:
type User struct {
ID UserID
Name string
}
Using UserID
as an alias for string
makes it clear that the ID
field is specific to a user, enhancing code clarity.
Conclusion
Golang type aliases are a powerful and often underutilized feature that can help you write cleaner, more maintainable code. By simplifying complex types, improving readability, and making refactoring easier, type aliases can provide significant benefits when used thoughtfully. However, it’s essential to use them appropriately and consistently to ensure your code remains easy to understand and maintain.
If you found this post helpful, be sure to explore other key Golang resources like gRPC with Go, the Art of CLI in Golang, and the Ultimate Guide to Debugging with Go. These guides will help you further your understanding of Golang and elevate your programming skills.