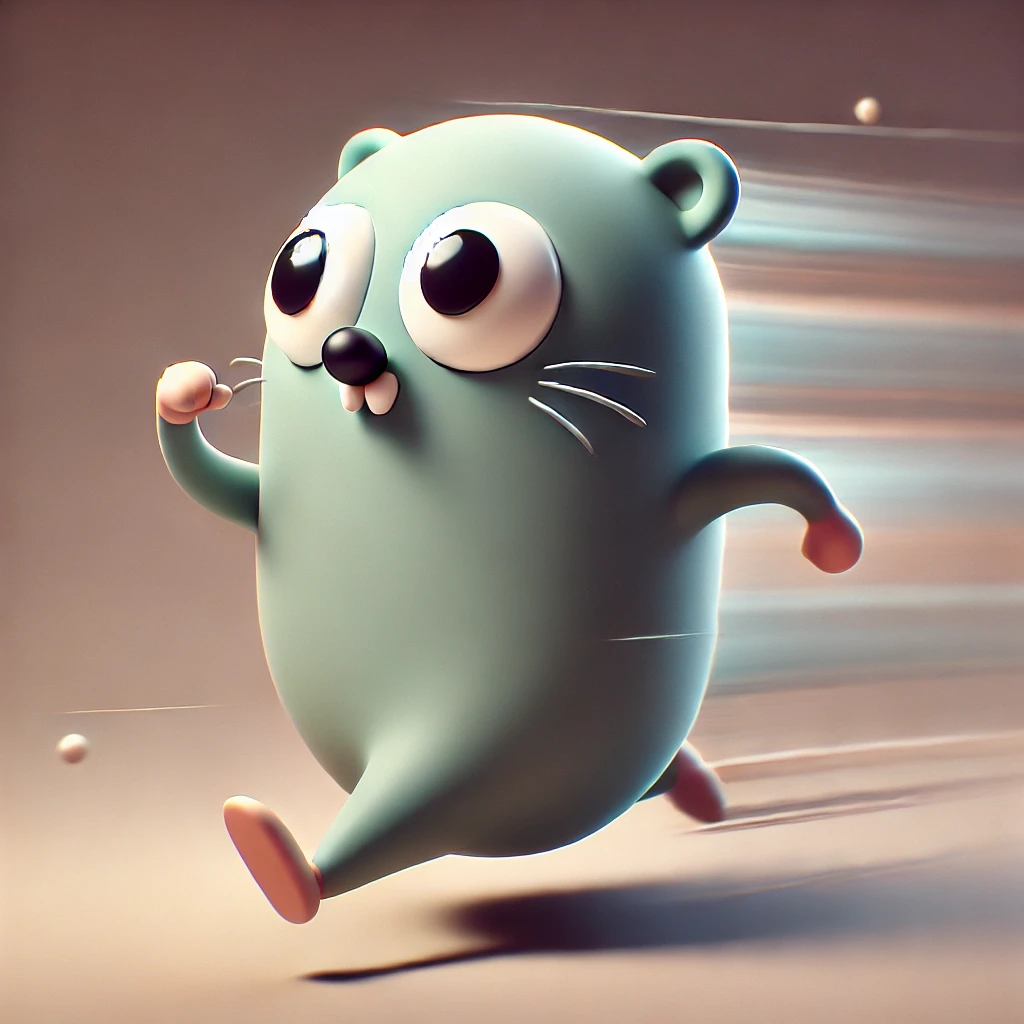
Boosting gRPC Performance | A Practical Guide for High-Throughput Applications
In the world of microservices, distributed systems, and real-time applications, performance can be the deciding factor between a successful system and one that struggles under load. gRPC (Google Remote Procedure Call) is a popular choice for building high-performance APIs, especially in systems where low latency and high throughput are key. Thanks to unique features like HTTP/2, protocol buffers, and efficient communication patterns, gRPC has become a preferred solution for cloud-native environments. But to get the most out of gRPC, you’ll need to make sure it’s optimized to handle the demands of your application.
In this guide, I’ll break down some proven techniques to boost gRPC performance, from network configurations to protobuf tuning and caching. If you’re just starting with gRPC in Go, this gRPC with Go guide provides foundational insights. And if you want to level up your Go setup, be sure to check out the Art of CLI in Golang and The Ultimate Guide to Debugging with Go for some great tips.
Understanding gRPC Performance Essentials
What is gRPC?
gRPC is an open-source RPC (remote procedure call) framework developed by Google that’s known for its efficiency in distributed systems. By using HTTP/2 for communication and Protocol Buffers (protobuf) for data serialization, gRPC reduces latency and maximizes throughput. That’s why it’s widely adopted in microservices, IoT applications, and cloud-native environments where speed matters.
Why Performance Matters in gRPC
When optimized properly, gRPC can deliver fast and efficient communication, enhancing user experience and keeping infrastructure costs low. But if it’s not tuned correctly, gRPC can turn into a bottleneck, causing delays, resource inefficiencies, and higher expenses. Optimizing gRPC isn’t just about squeezing out speed; it’s about building a system that’s stable and ready to scale.
Key Factors in gRPC Performance
Several components make gRPC a high-performance tool:
- HTTP/2: This enables multiplexing, which reduces the overhead of opening and closing connections.
- Protocol Buffers (Protobuf): This serialization format is fast and compact, which speeds up communication by reducing message size.
- Concurrency: gRPC can handle multiple calls over a single connection, which boosts throughput and reduces network usage.
Optimizing gRPC Network Configurations
Reducing Latency with HTTP/2
gRPC relies on HTTP/2 by default, which enables it to make multiple concurrent requests over one TCP connection. This minimizes the need to repeatedly open and close connections, which can save precious milliseconds. To fully leverage HTTP/2:
- Enable Persistent Connections: These connections are reused for multiple calls, reducing setup time.
- Utilize Multiplexing: HTTP/2 multiplexing allows multiple streams to run concurrently, which makes communication faster and more efficient.
Connection Pooling and Management
Using connection pooling can reduce latency and free up resources by reusing established connections. Here are a couple of key practices:
- Limit Idle Connections: Set a cap on idle connections to avoid using unnecessary resources.
- Fine-Tune Keep-Alive Settings: Adjust keep-alive settings so that frequently used connections stay active, which prevents the overhead of reconnecting.
Optimizing Load Balancing
Load balancing is essential for spreading traffic across servers and preventing any single server from getting overloaded. Effective load balancing can keep your gRPC calls running smoothly and reliably. Some approaches include:
- Round-Robin Load Balancing: This evenly distributes traffic across all servers, which works well if you have multiple service instances.
- Client-Side Load Balancing: Client-side balancing lets the client decide which server to contact based on load, improving scalability in larger systems.
- Use Proxies: Proxies like Envoy can manage gRPC load balancing, making it easier to distribute and scale your traffic.
Tuning gRPC with Protobuf Adjustments
Selecting Efficient Protobuf Types
Choosing the right protobuf data types can reduce the encoding and decoding time, which is key in performance-sensitive applications. Here are a few examples:
- Use int32 or int64 for numbers instead of strings; numeric types are more efficient for processing.
- Avoid nesting data structures if you can, as deeply nested structures can increase complexity and slow down serialization.
Reducing Message Size
Minimizing message size can reduce network load and speed up gRPC communication. Here’s how:
- Use Compression: Compressing larger messages can reduce latency, but it’s best to use fast compression algorithms to keep CPU usage low.
- Eliminate Redundant Data: Only include the data you actually need in protobuf messages.
- Use Packed Encoding: For repeated fields (like integers), packed encoding can reduce the size and improve serialization speed.
Schema Design for Speed
How you design your protobuf schemas can also affect performance. Here are a couple of best practices:
- Prioritize Lower-Numbered Fields: Since lower field numbers require fewer bytes, put your most-used fields in lower positions to improve efficiency.
- Use Oneof for Mutual Exclusivity: If only one of a few fields is used at a time, consider using
oneof
to manage exclusive fields, which reduces payload size and speeds up deserialization.
Leveraging Caching and Compression for Faster gRPC
Implementing Caching Strategies
Caching can cut down on repetitive calls to gRPC servers, reducing load and latency. Here’s how:
- Client-Side Caching: For data that doesn’t change frequently, client-side caching can reduce the number of server calls.
- Caching Middleware: Middleware solutions can intercept and cache responses, so the server only has to respond to new or changed requests.
- Leverage HTTP Caching Headers: HTTP headers like Cache-Control and ETag can help manage caching policies for gRPC responses.
Using Compression for Large Messages
For large messages, compression can significantly improve performance by reducing transfer times. Here are a few tips:
- Set Compression Parameters: Use compression algorithms like gzip or snappy that suit your system needs. Different compression settings can affect speed, so test to find a good balance.
- Selective Compression: Compress only large messages to save on CPU resources; small messages don’t always benefit from compression.
Choosing Compression Algorithms
Picking the right compression algorithm matters. Some, like gzip, have good compression rates but require more CPU. For a faster, lightweight option, consider Brotli if your environment can support it.
Monitoring and Benchmarking gRPC Performance
Monitoring is key to knowing how gRPC is performing and where you can improve. Here are some popular tools and practices:
- Prometheus and Grafana: These are widely used for collecting and visualizing gRPC performance metrics, letting you track request rate, error rate, and response time.
- Jaeger and OpenTelemetry: These tools help trace and analyze latency in distributed systems.
- Built-In gRPC Tracing: gRPC includes tracing tools for monitoring request handling and execution time, giving you granular performance insights.
By consistently monitoring and benchmarking your gRPC setup, you can find bottlenecks and adjust settings based on real-world traffic and load patterns, ensuring that gRPC meets your performance goals.
Conclusion
Tuning gRPC performance takes some effort, but it’s well worth it if you’re aiming for a responsive, high-throughput system. By focusing on network configurations, protobuf schema optimization, caching, and compression, you can build a highly efficient gRPC environment that scales smoothly. As your system grows and traffic patterns change, regular monitoring and benchmarking will help keep your gRPC performance on track. With these practices in place, gRPC can handle even the most demanding application requirements, providing smooth, reliable communication between services. If you want to learn more about this, check out our course which will teach you everything you need to know about gRPC and Go.
Frequently Asked Questions (FAQ)
Q1: What tools can I use to monitor and benchmark gRPC performance?
A1: Prometheus and Grafana are great for tracking gRPC metrics, while Jaeger and OpenTelemetry are useful for latency analysis. Built-in gRPC tracing also provides request-level insights.
Q2: Does using HTTP/2 improve gRPC speed?
A2: Absolutely. HTTP/2 allows for persistent connections and multiplexing, which means multiple streams over a single connection—making it faster and more efficient.
Q3: How can I reduce latency in gRPC communication?
A3: You can reduce latency by using connection pooling, enabling multiplexing, optimizing keep-alive settings, and using client-side load balancing to distribute traffic efficiently.
Q4: Are there any gRPC-specific load balancers?
A4: Yes, load balancers like Envoy and Linkerd work well with gRPC, providing client-side load balancing and managing distributed traffic effectively.
Q5: What’s the impact of message size on gRPC performance?
A5: Large messages increase latency and CPU usage, so it’s best to keep them small through efficient protobuf schemas, compression, and packed encoding to improve performance.