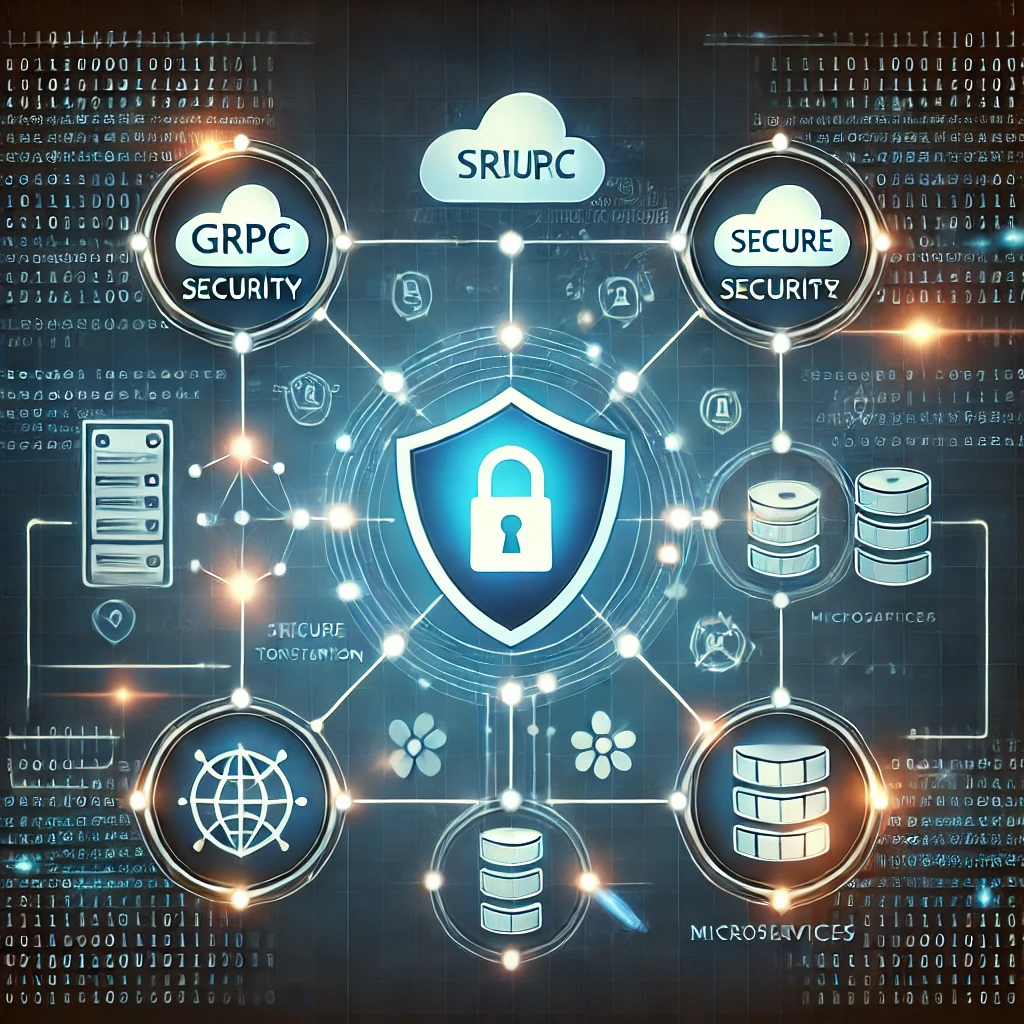
Enhancing gRPC Security | Best Practices for Secure Communication in Microservices
When it comes to connecting microservices securely, ensuring reliable and protected communication channels is critical. Google’s Remote Procedure Call (gRPC) framework has become a popular choice for building high-performance microservices, thanks to its speed and efficiency. But here’s the catch: the default gRPC setup doesn’t provide security out of the box. You need to add some security layers to make sure it’s truly safe.
If you’re diving into gRPC with Go, understanding gRPC with Go is a smart place to start—it covers the essentials of getting gRPC running with Go-based services. For even more on Go, check out our resources on debugging with Go and the art of CLI in Golang to get the most out of your Go development setup.
In this guide, we’ll break down the must-have security steps for gRPC, from encryption and authentication to logging and monitoring. Let’s make sure your microservices are protected from the ground up.
What is gRPC and Why is Security Important?
gRPC is an open-source, high-speed protocol that facilitates efficient communication between microservices, using HTTP/2 for streaming and multiplexing. It relies on Protocol Buffers, a compact binary serialization format that’s language-agnostic. In short, it’s a fast, efficient way for services to “talk” to each other, especially in distributed systems. Go and gRPC work really well together and are a match made in heaven.
But here’s the flip side: without added security, gRPC is exposed to risks. Without configurations to ensure protection, attackers could intercept data, gain unauthorized access, or even tamper with services. So, if you’re using gRPC, making security a priority isn’t just a good idea—it’s essential.
We cover off all aspects of gRPC security in our course.
Key Security Measures in gRPC
1. TLS Encryption for Data Protection
TLS (Transport Layer Security) is your first line of defense when securing gRPC communications. By encrypting data in transit, TLS ensures that information remains private and protected from prying eyes.
How to Set Up TLS in gRPC
Setting up TLS requires configuring both the client and server to use SSL certificates. Here’s how to get started:
- Generate SSL Certificates: For production environments, use a trusted Certificate Authority (CA) to generate SSL certificates. For internal services, self-signed certificates are an option, but they’re not ideal for production.
- Configure the gRPC Server: Add the SSL certificate and key to the server’s settings. Each language has its own gRPC library for SSL configuration, so make sure to follow the specifics of your environment.
- Enable Client-Side Authentication: Configure the client to trust the server’s certificate. This prevents man-in-the-middle attacks and ensures your client is connecting to a trusted server.
Using TLS in your gRPC implementation protects data privacy and integrity, making it much harder for malicious actors to intercept sensitive information.
2. Authentication Using JWT or mTLS
Authentication is what ensures that only verified users or systems can access your gRPC services. There are a few ways to handle authentication in gRPC, but two popular options are JWT and mTLS.
JSON Web Tokens (JWT)
JWTs are a popular choice for stateless client authentication. Each client request includes a token, which the server verifies to check the requester’s identity. JWTs are typically signed with HMAC or RSA algorithms, making them tamper-proof.
Advantages of JWT for gRPC Authentication:
- Stateless: The server does not need to retain any session data.
- Portable: JWTs can be easily shared across services.
- Flexible: You can add custom claims, like roles or permissions, to fine-tune access control.
Mutual TLS (mTLS)
mTLS takes security up a notch by verifying both client and server identities. This two-way authentication process is ideal for sensitive applications, as it ensures only authenticated services connect.
How to Set Up mTLS in gRPC:
- Issue Client Certificates: Each client needs a unique certificate from a CA.
- Configure Client Authentication: Set the server to require and validate client certificates.
- Verify Client Certificates: This confirms that the client’s identity is trusted before establishing a connection.
mTLS, combined with TLS encryption, offers a strong authentication mechanism for high-security needs.
3. Authorization and Access Control
Authentication answers who you are; authorization controls what you’re allowed to do. To manage this in gRPC, consider using role-based access control (RBAC) or attribute-based access control (ABAC), both of which restrict access based on roles or attributes.
Implementing Role-Based Access Control (RBAC)
RBAC grants permissions to roles instead of directly assigning them to individual users. For example, an admin role might have permission to access all endpoints, while a user role could have limited access to specific endpoints.
In gRPC, RBAC can be set up with middleware that intercepts requests to verify permissions before reaching the core service logic. This centralizes authorization and makes it easy to adjust access policies as needed.
Best Practices for Authorization in gRPC
- Limit Sensitive Endpoint Access: Only trusted roles should have access to high-risk endpoints.
- Use Middleware for Authorization: Middleware adds a consistent layer of protection without disrupting core service logic.
- Define Clear Permission Policies: Set clear policies for what each role can access to prevent accidental over-permissioning.
Access control ensures that even authenticated users only see what they’re authorized to, reducing the risk of privilege escalation.
4. Audit Logging and Monitoring
Audit logging provides a record of who accessed which resources and when. It’s essential for detecting unauthorized access and provides valuable forensic data in the event of a security incident.
Steps to Implement Effective Logging in gRPC
- Enable Request and Response Logging: Track each gRPC call with metadata like user identity, timestamps, and response status.
- Secure Log Storage: Use centralized, secure logging solutions to protect logs from tampering.
- Monitor and Analyze Logs: Review logs regularly and set up alerts for suspicious activities.
Pair logging with real-time monitoring tools like Prometheus or Grafana for insights into system health and early threat detection.
Challenges and How to Overcome Them
Implementing gRPC security isn’t without challenges, so here’s how to tackle common ones:
- Certificate Management: Regularly rotate SSL certificates. Automate renewal with tools like Certbot to streamline the process.
- Performance Overheads: TLS and mTLS can slow things down a bit. Minimize this by optimizing cryptographic settings and using lightweight algorithms.
- Compatibility: Ensure your gRPC security settings integrate smoothly with existing infrastructure, especially when adding layers like mTLS.
Planning and automation can help you manage these challenges, keeping your services secure and efficient.
Conclusion
Securing gRPC-based microservices requires more than a simple protocol setup—it needs a layered approach. By implementing TLS encryption, JWT or mTLS authentication, RBAC authorization, and diligent logging, you’ll have a robust framework for protecting sensitive data and ensuring service integrity.
Following these best practices allows you to build resilient microservices that uphold data security and trust, even in complex environments.
Frequently Asked Questions
1. What encryption does gRPC support?
gRPC supports TLS for encrypting data in transit, which ensures both privacy and data integrity.
2. How does mTLS enhance gRPC security?
mTLS adds an extra layer of protection by verifying both client and server identities, helping to ensure that only trusted entities communicate.
3. Can gRPC use API keys for authentication?
Yes, gRPC can use API keys, but JWT and mTLS are generally more secure and better suited for microservice environments.
4. What are best practices for managing certificates in gRPC?
Automate certificate rotation and renewal with secure, centralized management tools to minimize the risk of expired certificates.
5. Is gRPC secure by default?
No, gRPC isn’t inherently secure. To ensure protection, you’ll need to configure TLS, enforce authentication, and set up access controls.