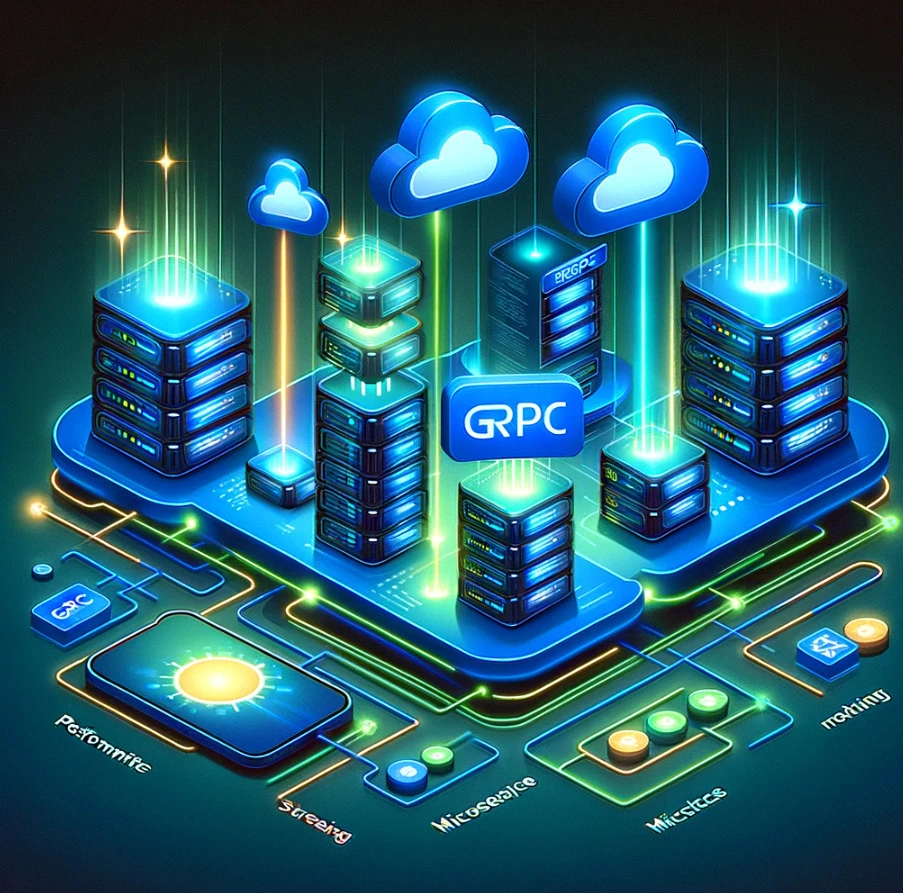
gRPC use cases
If you’ve been looking for a way to streamline communication in your systems, gRPC might just be your new best friend. In this post, we’re going to explore some real-world scenarios where gRPC excels, from microservices to mobile apps, and why it’s a favorite among developers.
For those of you working with Go, you’ll want to check out this detailed guide on using gRPC with Go to get a better understanding of its capabilities in a Go environment. If building command-line tools is part of your development journey, I also recommend exploring the “Art of CLI in Golang”. And if debugging is more your style, don’t miss out on The Ultimate Guide to Debugging with Go — all great content to support your gRPC endeavors.
What is gRPC?
So, what’s the big deal about gRPC? At its core, gRPC stands for Google Remote Procedure Call, and it’s changing the way we handle communication between systems. Unlike traditional REST APIs, which use HTTP/1.1, gRPC leverages the speed of HTTP/2. This means faster data transfer, lower latency, and advanced features like bi-directional streaming. Plus, it uses Protocol Buffers (Protobufs)—Google’s language-neutral and platform-neutral format for serializing data. This makes communication not only faster but also more compact, which is perfect for modern applications where every millisecond counts.
1. Microservices Communication with gRPC
Microservices are everywhere these days. They’re all about breaking down applications into smaller, manageable pieces, and gRPC fits into this puzzle perfectly. Here’s why developers love using gRPC for microservices:
High Performance: Thanks to HTTP/2, gRPC handles multiple connections simultaneously without getting bogged down. It’s a big step up from REST, especially when you’re dealing with lots of data. Type Safety: gRPC uses Protocol Buffers, which means every interaction is strongly typed. This makes it easier to catch errors early on and keeps communication clean and precise. Streaming Capabilities: If you’ve ever wished for real-time updates without hassle, gRPC has you covered. It supports client, server, and bi-directional streaming right out of the box—ideal for dashboards, monitoring tools, or anything that needs instant feedback. Companies like Netflix and Spotify are using gRPC for precisely these reasons. It keeps their complex microservices communicating smoothly, without any hiccups.
Practical Example: Setting Up a gRPC Service in Go To bring these concepts to life, here’s a simple “Hello World” example of a gRPC service implemented in Go:
// server.go
package main
import (
"context"
"log"
"net"
pb "example.com/microservices/proto" // Update with your module path
"google.golang.org/grpc"
)
// Server is used to implement the gRPC service
type Server struct {
pb.UnimplementedExampleServiceServer
}
// SayHello implements the ExampleService
func (s *Server) SayHello(ctx context.Context, in *pb.HelloRequest) (*pb.HelloReply, error) {
log.Printf("Received: %v", in.Name)
return &pb.HelloReply{Message: "Hello " + in.Name}, nil
}
func main() {
lis, err := net.Listen("tcp", ":50051")
if err != nil {
log.Fatalf("failed to listen: %v", err)
}
grpcServer := grpc.NewServer()
pb.RegisterExampleServiceServer(grpcServer, &Server{})
log.Println("gRPC server running on port 50051")
if err := grpcServer.Serve(lis); err != nil {
log.Fatalf("failed to serve: %v", err)
}
}
// client.go
package main
import (
"context"
"log"
"time"
pb "example.com/microservices/proto" // Update with your module path
"google.golang.org/grpc"
)
func main() {
conn, err := grpc.Dial("localhost:50051", grpc.WithInsecure())
if err != nil {
log.Fatalf("did not connect: %v", err)
}
defer conn.Close()
client := pb.NewExampleServiceClient(conn)
ctx, cancel := context.WithTimeout(context.Background(), time.Second)
defer cancel()
response, err := client.SayHello(ctx, &pb.HelloRequest{Name: "gRPC Developer"})
if err != nil {
log.Fatalf("could not greet: %v", err)
}
log.Printf("Greeting: %s", response.Message)
}
To test this implementation:
- Define a proto file for your service:
syntax = "proto3";
package example;
service ExampleService {
rpc SayHello(HelloRequest) returns (HelloReply);
}
message HelloRequest {
string name = 1;
}
message HelloReply {
string message = 1;
}
- Compile the .proto file using protoc:
protoc --go_out=. --go-grpc_out=. example.proto
- Run the server:
go run server.go
- Run the client:
go run client.go
This simple example demonstrates how gRPC facilitates high-performance communication in a microservices environment.
2. Real-Time Data Streaming with gRPC
In some industries, like finance or gaming, speed isn’t just important—it’s everything. That’s why gRPC has become a go-to for real-time data streaming:
Financial Services:
Imagine you’re trading stocks, and the data updates a fraction too late. With gRPC, banks and financial platforms get real-time market data updates without a hitch, allowing traders to make quick, informed decisions.
Gaming:
Multiplayer games thrive on split-second interactions. gRPC’s bi-directional streaming keeps game data in sync between server and client, ensuring a smooth and immersive player experience.
IoT (Internet of Things):
Think of all those connected devices sending data non-stop—smart homes, wearable tech, industrial sensors. gRPC’s efficiency makes it a top choice for handling continuous data streams with almost zero delay. For companies dealing with high-speed environments, gRPC isn’t just a nice-to-have; it’s a necessity.
3. gRPC for Mobile and Web Applications
If you’re developing mobile or web applications, you know how crucial it is to be efficient and fast. Here’s how gRPC stands out in this space:
Low Bandwidth Consumption:
Data needs to be compact when working with mobile networks, and gRPC’s Protocol Buffers are a lot more efficient than JSON. Less data sent means faster loading times.
Improved Performance:
Because gRPC uses HTTP/2, it can handle multiple requests at once over a single connection, reducing the time it takes for pages to load and apps to respond.
API Management:
Ever had to update an API and worried about breaking existing clients? gRPC makes versioning simple, so you can iterate without headaches. A lot of well-known mobile apps, from e-commerce platforms to social networks, are opting for gRPC to make sure data loads quickly and stays fresh, giving users a smooth, seamless experience.
4. Cross-Platform Interoperability with gRPC
One of the best things about gRPC is how well it plays with others. If you’ve got a project with components written in different languages or running on different platforms, gRPC is a lifesaver.
Polyglot Support:
Whether you’re coding in Java, Python, Go, C++, or Ruby, gRPC has you covered. It’s designed to support a wide range of programming languages, making it perfect for teams with diverse skills.
Legacy System Integration:**
Got some old systems that need to talk to modern microservices? gRPC can bridge that gap without a major overhaul, making integration simpler.
Cross-Platform Compatibility:
From Windows to Linux, from on-premises to the cloud, gRPC works smoothly across platforms. This makes it ideal for companies with complex tech stacks or hybrid environments. If your organization uses a mix of technologies, gRPC’s interoperability can save you tons of time and effort, streamlining communication across the board.
Conclusion
gRPC is changing the game for developers who need fast, reliable communication across a variety of applications. Whether you’re working on microservices, real-time data streaming, mobile apps, or multi-platform systems, gRPC offers a solution that’s efficient, flexible, and scalable. Its combination of HTTP/2, protocol buffers, and built-in streaming capabilities make it a standout choice for modern software development. As the tech world continues to evolve, one thing is clear: gRPC is here to stay. If you want to keep your systems ahead of the curve, it’s time to dive deeper into what gRPC can do for you.
Frequently Asked Questions (FAQs)
1. What is the difference between gRPC and REST?
While REST relies on HTTP/1.1, gRPC uses HTTP/2, allowing for faster communication with features like multiplexing and header compression. Plus, gRPC supports streaming—something REST doesn’t offer.
2. Is gRPC suitable for mobile applications?
Absolutely. gRPC’s low-latency communication and compact data format are perfect for mobile networks, helping apps load faster and use less data.
3. Can gRPC handle streaming data?
Yes, gRPC is built for streaming. Whether you need client-side, server-side, or bi-directional streaming, gRPC handles it all, making it ideal for real-time applications.
4. Does gRPC support multiple programming languages?
Yes, gRPC is a polyglot framework. It supports a wide range of languages—Java, Python, Go, C++, and more—making it a versatile choice for diverse tech stacks.
5. How does gRPC ensure secure communication?
gRPC uses HTTP/2’s built-in encryption features and supports Transport Layer Security (TLS), so communication between client and server is always secure.