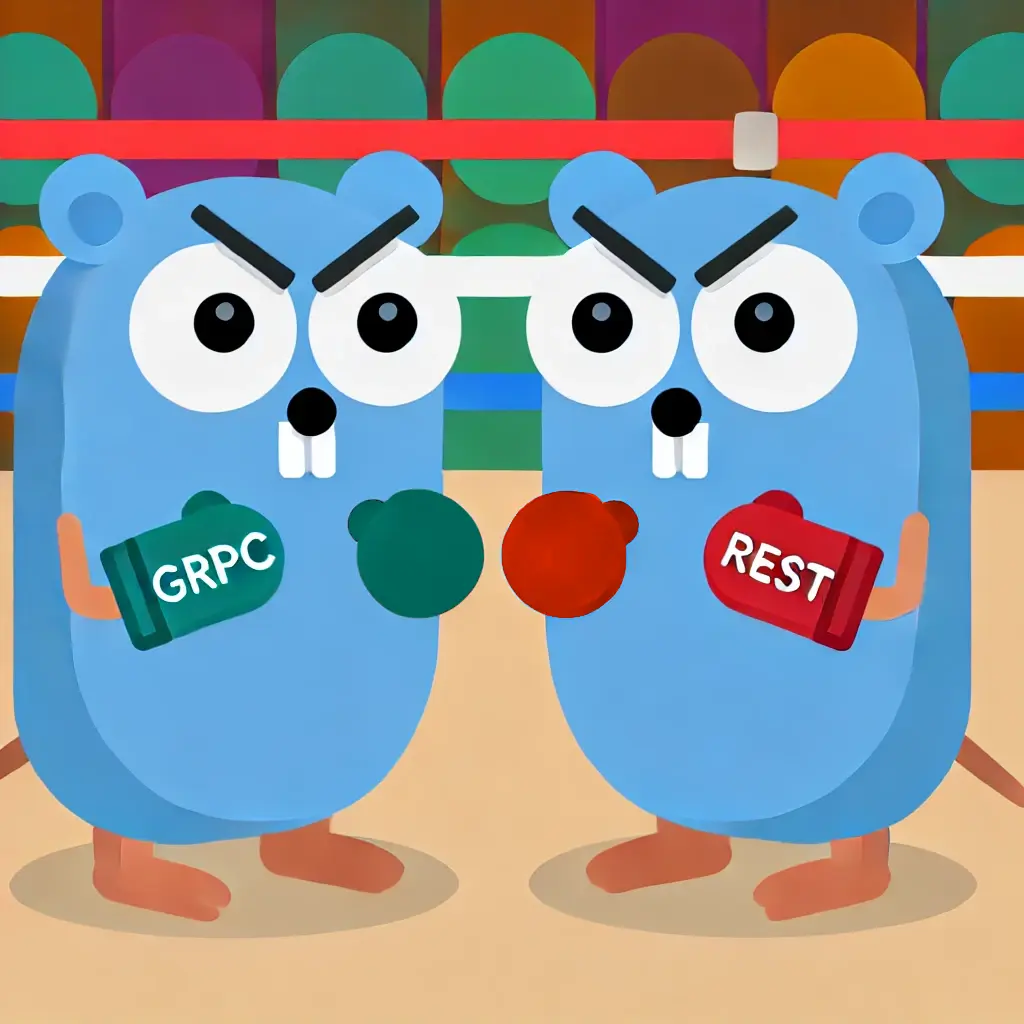
gRPC vs REST
At some point in a growing company, the debate between gRPC and REST becomes a focal point. Both technologies allow communication between distributed systems, yet they differ significantly in their design, functionality, and use cases. This blog post aims to explain these differences, presenting a detailed comparison between gRPC and REST, and exploring scenarios where one might be more suitable than the other.
What is gRPC?
gRPC is an open-source Remote Procedure Call (RPC) framework developed by Google. It operates over HTTP/2 and uses Protocol Buffers (protobuf) for data serialization. Unlike REST, which is heavily reliant on HTTP/1.1 and JSON, gRPC is designed to be language-agnostic and is made for distributed systems environments. It offers several built-in features such as load balancing, tracing, health checking, and authentication, making it modular and highly configurable.
How do I build a gRPC service?
The workflow for creating gRPC services involves defining a protobuf contract. This contract specifies the RPCs, requests, and response structures. Once defined, these protobuf files are compiled using the Protocol Buffers compiler, protoc
, which generates the necessary client and server interfaces for various programming languages. This generated code can then be utilized by microservices in a whole host of languages to communicate. You can see the currently supported languages here.
For example, we start by defining the service and message structures in a .proto
file. This would look something like the following:
message Person {
string name = 1;
int32 id = 2;
string email = 3;
message PhoneNumber {
string number = 1;
PhoneType type = 2;
}
repeated PhoneNumber phones = 4;
google.protobuf.Timestamp last_updated = 5;
}
enum PhoneType {
PHONE_TYPE_UNSPECIFIED = 0;
PHONE_TYPE_MOBILE = 1;
PHONE_TYPE_HOME = 2;
PHONE_TYPE_WORK = 3;
}
message AddressBook {
repeated Person people = 1;
}
The protoc
compiler then generates code, including domain objects, server interfaces, and client stubs. These generated components allow the implementation of the server and the instantiation of clients that can make RPC calls. If you want to learn more about gRPC, you can learn everything you’ll ever need to know and more here.
What are the Advantages of gRPC?
One of the primary benefits of gRPC is its strong message structure. Because the communication is entirely contract-based, it minimises the risk of breaking changes and ensures type safety - we can even build breaking change detection into CI using something like Buf. This makes gRPC a great choice for microservice architectures where services need to communicate frequently and reliably.
Another significant advantage is its use of HTTP/2, which offers much better performance and efficiency compared to HTTP/1.1. HTTP/2 supports multiplexing (a fancy way of saying multiple requests over one connection). This feature reduces network latency and means we can do near real-time communication through long-lived connections.
Additionally, protobuf messages are marshaled before being sent over the network, so protobuf message sizes are much smaller than text-based formats like JSON. gRPC’s built-in message compression and smaller message sizes compared to JSON make it great for bandwidth-constrained environments like IoT.
What are the Use Cases for gRPC?
gRPC is well-suited for:
- Microservices architectures that require efficient and reliable communication.
- Real-time applications like gaming and financial trading platforms.
- IoT environments where devices have limited processing power and bandwidth.
- Systems requiring strong typing and strict interface definitions to avoid runtime errors.
What is REST?
REST (Representational State Transfer) is a widely adopted architectural style for designing networked applications, particularly for web services. First introduced in 2000, REST is centered on stateless communication, using standard HTTP methods such as GET, POST, PUT, DELETE, etc.
RESTful APIs follow a resource-based approach where everything is considered a resource, identifiable through a unique URI (Uniform Resource Identifier). These resources can be accessed and manipulated using a defined set of operations, which makes REST easy to understand for developers who are familiar with HTTP protocols. Here’s the above protobuf definition defined in Open-API format, a popular way to describe schemas and generate code.
openapi: 3.0.0
info:
title: Address Book API
version: 1.0.0
paths:
/persons:
get:
summary: Get all persons
operationId: getPersons
responses:
'200':
description: A list of persons
content:
application/json:
schema:
$ref: '#/components/schemas/AddressBook'
post:
summary: Create a new person
operationId: createPerson
requestBody:
description: Person object that needs to be created
required: true
content:
application/json:
schema:
$ref: '#/components/schemas/Person'
responses:
'201':
description: Person created successfully
What are the Advantages of REST?
One of the key reasons REST has become so popular is its simplicity and it aligns well with the underlying structure of HTTP. As mentioned, REST’s resource-centric design fits developers mental models for how the web works, and it has been around for so long now that it’s very prevalent.
Another significant advantage of REST is its wide compatibility. REST APIs can be consumed by virtually any client, from web browsers and mobile applications to IoT devices. This broad compatibility allows REST APIs to integrate with a variety of systems, platforms and even operating systems, making it a go-to choice for developers building applications that need to be accessible from different environments.
REST’s use of human-readable formats, such as JSON or XML is another reason its popular.. These text-based formats make the data exchanged between clients and servers easier to read and [debug](https://www.bytesizego.com/blog/rubber-duck-debugging.
Additionally, REST benefits from HTTP’s built-in support for caching. By utilizing headers like Cache-Control and ETag. This feature allows for previously fetched resources to be reused without requiring additional server requests.
What are Some use Cases for REST?
REST is ideal for the following plus many more:
- Web applications providing a standard way for front-end applications to communicate with the server and retrieve or change data.
- Mobile applications that require dynamic content without the need for client-side updates.
- Public APIs to allow third-party developers to interact with their platforms. REST’s wide adoption and simplicity ensures that these APIs can be used with minimal friction.
- REST is particularly suitable for situations where simplicity, flexibility, and broad compatibility are the primary concerns.
Comparing gRPC and REST
Feature | gRPC | REST |
---|---|---|
Protocol | HTTP/2 | HTTP/1.1 |
Data Format | Protocol Buffers (binary) | JSON or XML (text) |
Performance | Higher performance | Lower performance |
Streaming | Bi-directional | N/A |
API contract | Strongly typed | Flexible |
Browser Support | Limited, using gRPC-Web | Broadly supported |
Error Handling | Explicit status codes | HTTP status codes |
Protocol
- gRPC: Utilizes HTTP/2, which supports multiplexing and provides enhanced performance and efficiency.
- REST: Typically uses HTTP/1.1, which is more widely compatible but less efficient.
Streaming
- gRPC: Offers bidirectional streaming, enabling continuous data exchange between client and server.
- REST: Operates on a request-response model without native support for streaming.
Data Format
- gRPC: Uses Protocol Buffers, resulting in compact and fast message serialization.
- REST: Employs text-based formats like JSON or XML, which are more readable but larger in size.
Typing
- gRPC: Enforces strong typing with strict interface definitions, leading to clearer contracts and fewer runtime errors.
- REST: More flexible but potentially less precise due to its loosely defined contracts.
Browser Support
- gRPC: Limited native support, requiring gRPC-web for browser usage.
- REST: Universally supported across web browsers.
Use Cases
- gRPC: Ideal for microservices and performance-critical applications.
- REST: Suitable for simpler applications requiring broad compatibility with various clients.
Wrapping Up
Both gRPC and REST have their own strengths and ideal use cases. gRPC’s strong typing, efficient serialization, and support for real-time communication make it a great tool for modern microservices architectures and performance-critical applications. On the other hand, REST’s simplicity, flexibility, and universal browser support make it a reliable choice for a wide range of applications.
Understanding the specific requirements of your project will enable you to choose the right technology. Whether you prioritize performance, efficiency, or simplicity, both gRPC and REST offer great solutions for building and maintaining distributed systems.
How can I learn more about gRPC?
You can read more of our blogs about gRPC here, here and here.
We also have a course which assumes no gRPC knowledge and will teach you everything you need to know here.