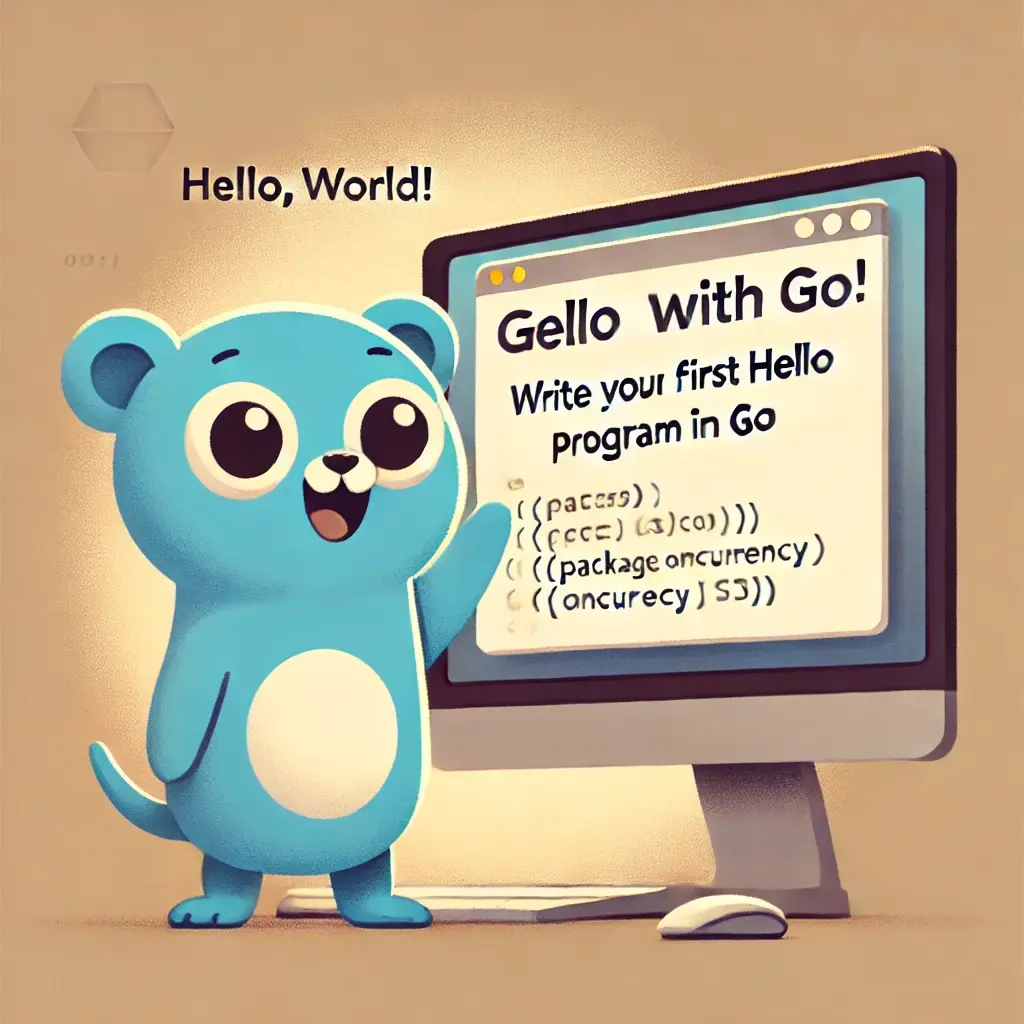
Getting Started with Go: Write Your First Hello World Program in Go
Hey there! If you want to dive into the programming world, you’ve come to the right place. Today, we’ll get started with Go (or Golang, as some folks call it) by writing your very first "Hello World"
program. But that’s not all—I’ll also point you to some fantastic resources like the Ultimate Guide to Debugging with Go and gRPC with Go to help you on your journey. Plus, if you’re interested in building command-line applications, check out the Art of CLI with Go.
Why Choose Go for Your Programming Journey?
Overview of Go and Its Key Features
So, why Go? Well, it’s an open-source programming language created by Google, and it’s designed to be simple and efficient. Whether you’re a newbie or an experienced coder, Go has something for you. One of its coolest features is its concurrency model, which lets you run multiple tasks at the same time. This is super handy and we explore it in other byteSizeGo blogs.
Benefits of Learning Go for Beginners and Experienced Programmers
For those just starting, Go’s clean syntax makes it easier to learn than languages like C++ or Java. And for seasoned developers? They appreciate Go for its performance and memory management. It’s perfect for creating scalable applications across various fields like web development and cloud services.
Real-World Applications and Industries Where Go Is Popular
- Cloud Services: Many cloud-based applications are built with Go.
- Web Development: Frameworks like Gin and Echo make web app development a breeze.
- DevOps Tools: Tools such as Docker and Kubernetes are written in Go, showcasing its importance in the DevOps realm.
- Networking: Thanks to its concurrency model, Go is a go-to for developing network servers and applications.
Go really can be used for almost everything, which explains why its getting so popular.
Setting Up Your Go Environment
Step-by-Step Guide to Installing Go on Windows, macOS, and Linux
Ready to install Go? Let’s get you set up. Here’s how to do it based on your operating system:
Windows
- Get the Go installer from the pofficial Go website](https://golang.org/dl/).
- Run the installer and follow the prompts. Easy peasy!
- Set the
GOPATH
environment variable to your workspace directory. - To check if it’s all good, open Command Prompt and type
go version
.
macOS
- If you use Homebrew, just open Terminal and run
brew install go
. - Alternatively, grab the binary from the Go website.
- Don’t forget to set the
GOPATH
in your.bash_profile
or.zshrc
. - Verify your installation with
go version
.
Linux
- Download the tarball from the Go website.
- Extract it to
/usr/local
with the command:tar -C /usr/local -xzf go*.tar.gz
. - Add
/usr/local/go/bin
to yourPATH
by editing.bashrc
or.profile
. - Confirm it’s working with
go version
.
How to Configure the Go Environment Variables
Setting up environment variables is key to working smoothly with Go. Here’s what you need:
GOPATH
: This is your workspace directory where all your Go code lives (by default, it’s~/go
).GOROOT
: This is where Go itself is installed, usually set automatically.
Want to see how it’s all set up? Just run go env
in your terminal.
Verifying Your Go Installation with Basic Commands
To ensure everything’s running smoothly, try these commands:
go version
: This tells you what version of Go you have installed.go env
: This shows your current Go environment settings.
If you see what you expect, congratulations—you’re all set to start coding!
Writing and Running Your First “Hello World” Program in Go
Explanation of Go’s Syntax with a Simple “Hello World” Example
Alright, let’s write that "Hello World"
program! Open your favorite text editor or IDE and create a new file named hello.go
. Here’s what the code looks like:
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
package main
: This tells Go that you’re creating an executable program.import "fmt"
: This imports thefmt
package, which lets you format your output.func main()
: This is where your program starts running.fmt.Println()
: This function prints your message to the console.
Guide to Creating, Saving, and Running Go Files
-
First, create a new directory for your project:
mkdir hello-go cd hello-go
-
Create the
hello.go
file:nano hello.go
-
Paste in the code and save it.
-
To run your program, launch the terminal and navigate to your project folder. Then type:
go run hello.go
You should see: Hello, World!
popping up on your screen!
Common Mistakes Beginners Make and How to Avoid Them
Here are some frequent mistakes to keep an eye on:
- Forgetting the
package main
: Don’t skip this—it’s crucial! - Mismatched brackets or parentheses: Double-check those!
- Not using the
.go
extension: Go needs this to recognize your file as Go code.
Understanding the Go Programming Workflow
How the Go Build System Works
Go has a built-in build system that makes it super easy to compile and run your code. Instead of dealing with complicated steps, you can just use the go
command for everything:
go run
: This command compiles and runs your program all in one go.go build
: This creates an executable file that you can run separately.go install
: This installs your binary into your$GOPATH/bin
directory for easy access.
With these commands, you can focus more on coding and less on the nitty-gritty of compilation.
Basics of Go Modules for Managing Dependencies
Starting with Go 1.11, managing dependencies became a lot easier thanks to modules. Here’s how to get started with them:
-
Initialize a module in your project directory:
go mod init hello-go
-
As you add external packages, Go automatically keeps track of them in a
go.mod
file. -
Want to add a package? Just use:
go get github.com/some/package
Tips for Efficient Coding and Debugging in Go
- Use
go fmt
: This formats your code according to Go standards—trust me, your future self will thank you! - Go Doc: Want to learn more about packages? Use
go doc
. - Testing: Go makes testing easy with its built-in framework. Create test files with the
_test.go
suffix and rungo test
to check your code.
Conclusion
Learning Go is a fantastic choice if you’re looking to boost your programming skills. Its simple syntax and powerful features make it a solid platform for building modern applications.
As you continue this journey, remember to practice and explore different projects to really get the hang of it. Whether it’s web development or cloud computing, the skills you pick up in Go will serve you well. And don’t forget to explore gRPC with Go for even more advanced concepts!
What challenges have you faced while learning Go? I’d be eager to hear about your experiences in the comments! If you found this guide helpful, share it with others who might be interested in learning Go.
Frequently Asked Questions
-
Is Go a good language for beginners?
Absolutely! Go is known for its straightforwardness, making it an excellent choice for newbies. Its clean syntax and robust standard library simplify the learning process. -
What are the main differences between Go and Python?
Go is geared more towards performance and concurrency, while Python shines in rapid prototyping and has a wealth of libraries for various tasks. -
Do I need any prior coding experience to learn Go?
Nope! Go’s simplicity makes it accessible even for those new to programming. However, a basic understanding of programming concepts can help. -
How do I install Go on my computer?
You can download the Go installer from the official website and follow the instructions for your operating system. -
What’s the best way to practice Go as a beginner?
Start with simple projects, explore Go’s built-in packages, and gradually tackle more complex tasks to build your confidence.