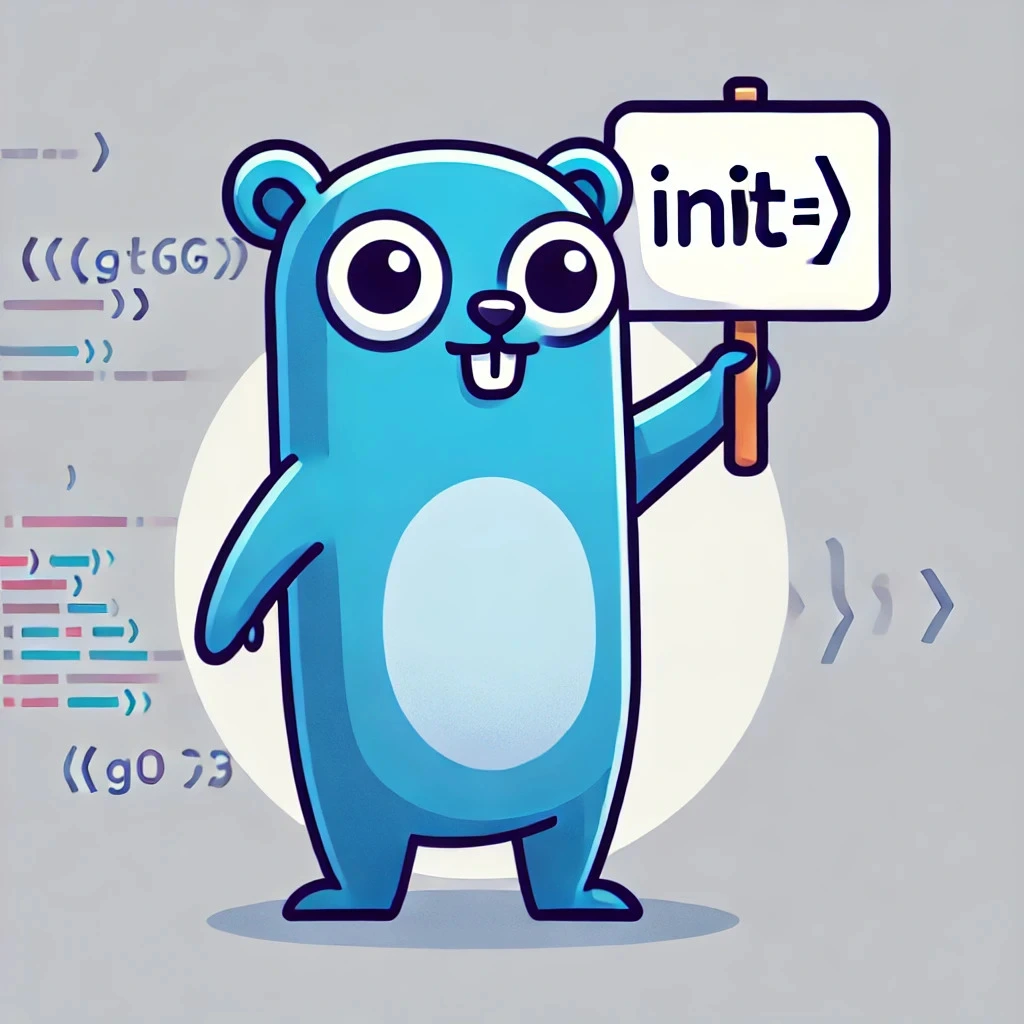
Why You Should Think Twice Before Using the init Function in Go
The init function in Go is a powerful feature that allows developers to perform initialization tasks before the program starts executing. While it might seem convenient, relying heavily on the init function can lead to code that’s difficult to understand and maintain (and may not work how you want).
In this article, we’ll explore why you should reconsider using the init function in your Go (Golang) projects, balancing the pros and cons to help you make informed decisions.
Understanding the init Function in Go
Before diving into the reasons to avoid it, let’s briefly recap what the init function does:
- Automatic Execution: The init function is called automatically when a package is initialized, right before the main function executes.
- Multiple init Functions: You can have multiple init functions within a single package or even a single file.
- No Parameters or Returns: The init function cannot accept parameters or return values.
Reasons to Avoid Using the init Function in Go
1. Hidden Side Effects
The init function can introduce side effects that are not immediately apparent when reading the code. Since it runs automatically, any setup or changes it makes happen behind the scenes, which can surprise developers who are not expecting it.
- Complex Debugging: Hidden initializations make debugging more complicated because the program state changes without explicit calls.
- Unpredictable Behavior: Unexpected side effects can lead to unpredictable behavior, especially in larger codebases.
Here’s an example. Let’s imagine this config.go
:
// config/config.go
package config
import (
"fmt"
)
var ConfigValue string
func init() {
fmt.Println("Running config.init()")
ConfigValue = "Initialized in init()"
}
and this main.go
:
// main.go
package main
import (
"fmt"
"myapp/config"
)
func main() {
fmt.Println("Starting main()")
config.ConfigValue = "Set in main()"
fmt.Println("ConfigValue:", config.ConfigValue)
}
You might expect this output:
Starting main()
ConfigValue: Set in main()
but you actually get:
Running config.init()
Starting main()
ConfigValue: Set in main()
This could be problematic if a service or other package expected that value to be set already.
2. Testing Difficulties
Testing code that relies on the init function can be challenging.
- Order of Execution: The execution order of init functions across packages can affect tests, making them flaky or unreliable.
- Isolation Issues: Since init runs automatically, it can be hard to isolate and test individual components without triggering unwanted initializations.
Here’s an example of a flaky test because of the init function. Imagine we have a shared variable:
// counter/counter.go
package counter
var Value int
and the following:
// packageA/packageA.go
package packageA
import "myapp/counter"
func init() {
counter.Value += 1
}
// packageB/packageB.go
package packageB
import "myapp/counter"
func init() {
counter.Value *= 2
}
and the following test case:
// main_test.go
package main
import (
"myapp/counter"
_ "myapp/packageA"
_ "myapp/packageB"
"testing"
)
func TestCounterValue(t *testing.T) {
expected := 2 // Expecting Value to be (0 + 1) * 2 = 2
if counter.Value != expected {
t.Errorf("Expected %d, got %d", expected, counter.Value)
}
}
3. Decreased Readability and Maintainability
Using init functions can make your code less transparent.
- Implicit Actions: Developers new to the codebase might not realize that certain initializations occur in the init function.
- Maintenance Overhead: Over time, as the code evolves, the init function can become a dumping ground for various initializations, increasing complexity.
4. Package Import Issues
The init function can cause problems with package imports.
- Unintended Imports: Importing a package solely for its side effects (i.e., its init function) can lead to messy dependencies.
- Cyclic Dependencies: Overuse of init functions can contribute to cyclic dependencies between packages, complicating the build process.
When init Can Be Useful
While there are valid concerns about using the init function, it’s not entirely without merit.
Initialization of Complex Variables
For initializing complex variables that require more than a simple assignment, init can be handy. For example, think of a cache or some precomputed values:
// palindrome/palindrome.go
package palindrome
var palindromeTable map[string]bool
func init() {
palindromeTable = make(map[string]bool)
words := []string{"level", "radar", "world", "deified", "hello"}
for _, word := range words {
palindromeTable[word] = isPalindrome(word)
}
}
func isPalindrome(s string) bool {
n := len(s)
for i := 0; i < n/2; i++ {
if s[i] != s[n-1-i] {
return false
}
}
return true
}
func IsPalindrome(word string) bool {
if val, exists := palindromeTable[word]; exists {
return val
}
// If word not in table, compute and store it
result := isPalindrome(word)
palindromeTable[word] = result
return result
}
Setting Up Test Data
In testing scenarios, init can prepare the necessary environment before tests run.
For example, suppose you have a package that interacts with a database. During tests, you might want to initialize mock data.
// data/data.go
package data
var Users []string
func init() {
// In tests, we might want to initialize this differently
if len(Users) == 0 {
Users = []string{"Alice", "Bob", "Charlie"}
}
}
func GetUsers() []string {
return Users
}
Best Practices If You Choose to Use init
If you decide that using the init function is necessary, consider these best practices:
- Keep It Simple: Limit the init function to simple, unavoidable initializations.
- Avoid Side Effects: Do not perform actions that alter the state in unexpected ways.
- Document Thoroughly: Clearly document what the init function does to aid other developers.
Alternatives to Using init
- Explicit Initialization Functions: Create functions that initialize your package and require explicit calls.
- Singletons or Lazy Initialization: Use patterns that initialize resources when they are first needed.
Wrapping up
I hope you found this useful! If you did, here are some more byteSize blogs and free courses you might enjoy: