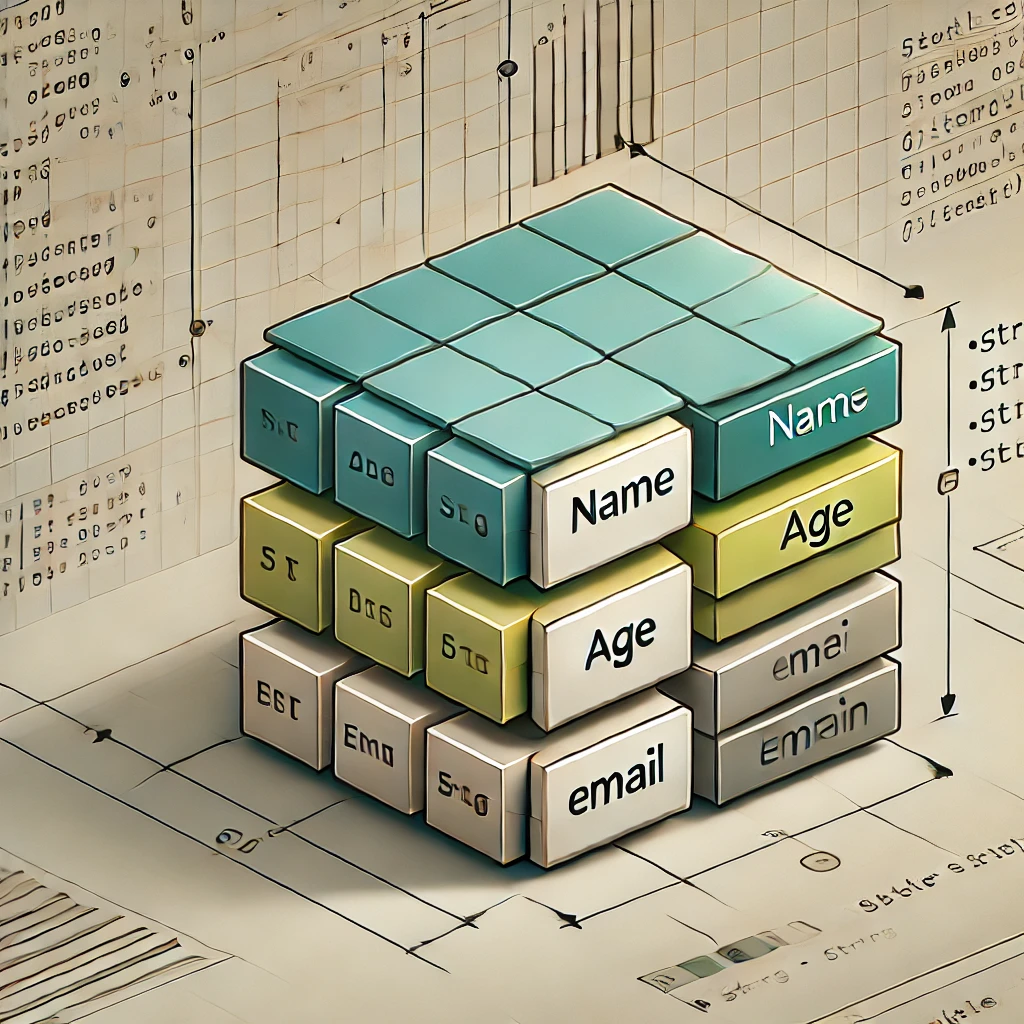
Golang JSON to Struct: A Complete Guide
Working with JSON in Go is a common task, whether you’re building APIs, consuming third-party data, or handling configurations. The challenge often lies in converting JSON data into Go structs efficiently. In this guide, we’ll explore how to perform this conversion quickly and effectively.
Why Convert JSON to Structs in Go?
Go’s strong typing ensures your data is structured and predictable. By converting JSON into Go structs, you gain:
- Type safety: Prevent runtime errors by ensuring the data adheres to the struct’s schema.
- Better readability: Your code becomes easier to understand with clear field definitions.
- Improved tooling: Tools like json-to-go and IDEs like GoLand streamline the process.
Steps to Convert JSON to Struct in Golang
1. Write or Obtain the JSON Data
Here’s an example of a simple JSON object:
{
"name": "John Doe",
"age": 30,
"email": "johndoe@example.com"
}
2. Use the json-to-go
Tool
Visit the json-to-go website and paste your JSON into the input box. The tool generates the equivalent Go struct instantly:
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
Email string `json:"email"`
}
3. Paste the Struct into GoLand
If you’re using Goland, simply copy the JSON data to your clipboard, right-click in the editor, and select Paste JSON as Struct. GoLand automatically generates the Go struct for you, including the appropriate json
tags.
This feature saves time and ensures your struct matches the JSON schema perfectly. Check out our free course on mastering Go with Golang for more tips and tricks like this.
4. Parse JSON into the Struct
With your struct ready, you can parse JSON data into it using Go’s encoding/json
package:
package main
import (
"encoding/json"
"fmt"
)
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
Email string `json:"email"`
}
func main() {
jsonData := `{"name":"John Doe","age":30,"email":"johndoe@example.com"}`
var person Person
err := json.Unmarshal([]byte(jsonData), &person)
if err != nil {
fmt.Println("Error decoding JSON:", err)
return
}
fmt.Printf("Decoded Struct: %+v\n", person)
}
5. Verify Your Struct
Once you’ve unmarshalled the JSON, always verify the struct’s values. This helps catch discrepancies between the JSON schema and your struct.
Conclusion
Converting JSON to Go structs doesn’t have to be a manual, error-prone process. With tools like json-to-go and IDE features like GoLand’s Paste JSON as Struct, you can save time and reduce mistakes.
Try out these techniques and streamline your workflow!
Looking for more tips on Go development? Explore our blog for practical guides and tutorials.