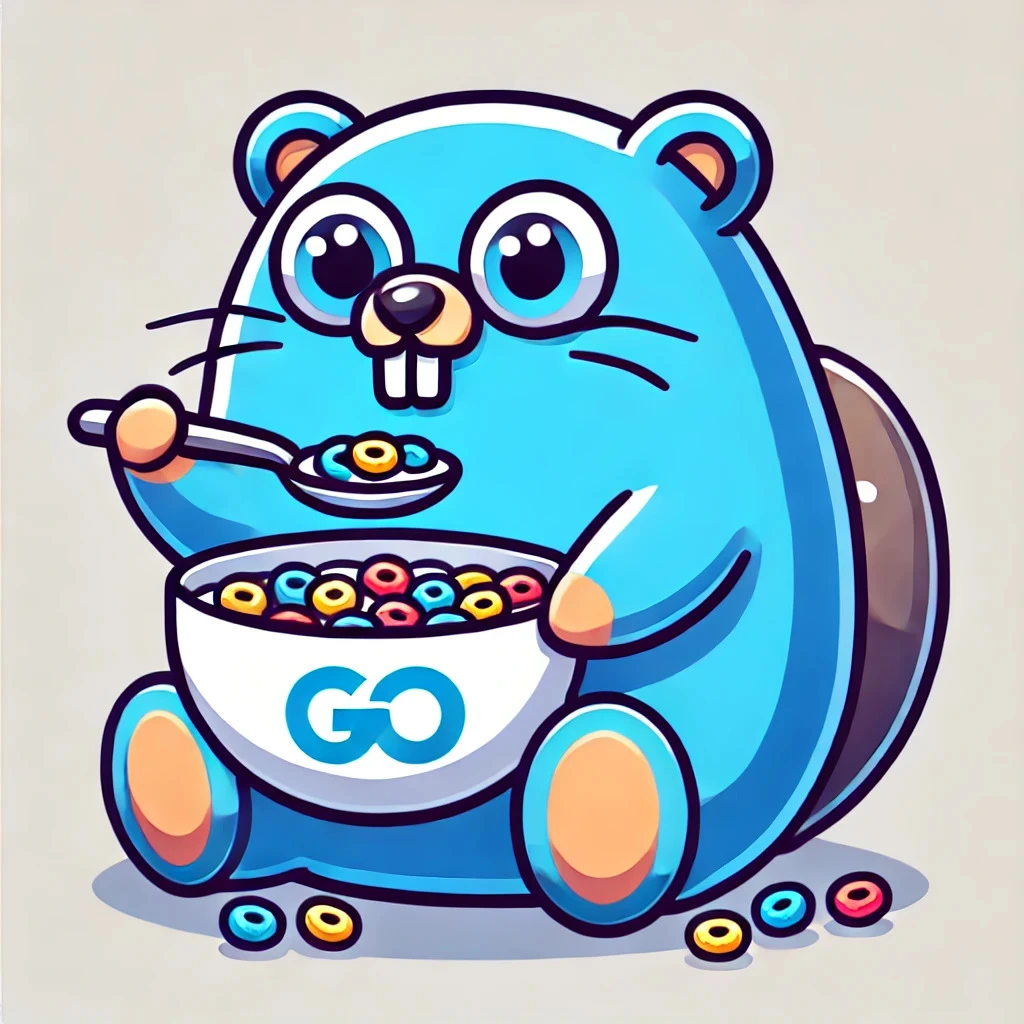
Mastering gRPC Error Handling: Best Practices and Key Strategies
As microservices architecture becomes the backbone of modern development, gRPC has emerged as a powerful tool for building high-performance, scalable services. But like any complex system, it’s essential to know how to manage errors efficiently to keep your microservices running smoothly. In this post, we’ll dive into gRPC error handling and explore key strategies for making your services more resilient.
Before we dive into gRPC error handling, it’s important to have a solid foundation in Go and debugging, which is crucial for understanding and resolving gRPC-related issues. Check out our resources like The Ultimate Guide to Debugging with Go to sharpen your debugging skills. If you’re just getting started with gRPC, you might also find our post on gRPC with Go a helpful introduction, as well as our in-depth course on Building Production-Ready Services with gRPC and Go.
What Is gRPC and Why Is Error Handling Important?
gRPC (Google Remote Procedure Call) is an open-source framework developed by Google that allows for efficient communication between distributed systems using HTTP/2 and Protocol Buffers for serialization. It also supports features like bi-directional streaming and multiplexing, which make it an ideal choice for building microservices.
However, despite its performance advantages, gRPC error handling is still essential for maintaining service reliability. Without a proper error-handling strategy, even minor issues can cascade, causing widespread service outages. That’s why knowing how to handle errors in gRPC calls is essential for creating robust, scalable applications.
If you’re just getting started with gRPC and want a comprehensive introduction to its implementation in Go, be sure to check out our detailed guide on gRPC with Go.
Common gRPC Error Codes
gRPC defines a series of status codes to help developers diagnose and resolve errors that occur during communication between services. These codes are crucial for effective gRPC error handling, and understanding them will help you build more reliable systems.
Here are some of the most common gRPC error codes:
- OK (0) – The operation completed successfully.
- INVALID_ARGUMENT (3) – The client sent an invalid argument in the request.
- DEADLINE_EXCEEDED (4) – The operation took too long and exceeded the set deadline.
- NOT_FOUND (5) – The requested resource was not found.
- UNAUTHENTICATED (16) – The request does not include the required authentication credentials.
Each of these codes provides specific insights into the nature of the error, making it easier to identify the root cause and resolve issues efficiently.
Best Practices for gRPC Error Handling
1. Use the Correct Status Codes
The first step in effective gRPC error handling is ensuring that your service returns the correct status codes. Returning INVALID_ARGUMENT for invalid client requests or NOT_FOUND for missing resources helps the client understand the exact nature of the problem and allows for quicker resolution. Proper status codes are key to building systems that are easy to debug and troubleshoot.
Ready to enhance your debugging expertise? Be sure to read The Ultimate Guide to Debugging with Go, where we discuss the best tools and techniques to identify and fix errors efficiently.
2. Provide Detailed Error Messages
While status codes offer useful information, adding detailed error messages can provide even more context. For example, using gRPC Status and ErrorDetails, you can give clients deeper insight into what went wrong. Here’s a Go example:
import (
"google.golang.org/grpc/codes"
"google.golang.org/grpc/status"
)
func example() error {
return status.Errorf(codes.InvalidArgument, "Invalid request: Incorrect input data.")
}
Adding detailed messages helps make your gRPC error handling more transparent and easier to diagnose.
3. Implement Retry Logic for Transient Errors
Not all errors are fatal. Transient errors, such as UNAVAILABLE or DEADLINE_EXCEEDED, often resolve themselves if retried. Implementing retry logic with exponential backoff is a best practice.
for retries < maxRetries {
response, err := client.SomeCall()
if err != nil && isTransient(err) {
time.Sleep(backoff(retries))
retries++
} else {
return err
}
}
Retry logic helps ensure that services remain available even when some calls fail intermittently.
4. Set Deadlines and Timeouts
One of the most effective ways to avoid unnecessary delays and improve your service’s responsiveness is by setting deadlines and timeouts. In gRPC, both clients and servers can use deadlines to control how long they wait for operations to complete. Here’s how you can set a deadline in Go:
ctx, cancel := context.WithTimeout(context.Background(), 5*time.Second)
defer cancel()
response, err := client.SomeCall(ctx, request)
if err != nil {
// Handle deadline exceeded error
}
Deadlines ensure that services don’t get stuck waiting for unresponsive or slow operations, a key component of effective gRPC error handling.
5. Monitor and Log gRPC Errors
Monitoring and logging errors is essential for maintaining the health of your microservices. Use tools like Prometheus and Grafana to track error rates, latencies, and other important metrics. These tools allow you to stay proactive, catch issues early, and fine-tune your gRPC error handling strategy.
Handling Streaming Errors in gRPC
In addition to unary RPC calls, gRPC also supports streaming, which can complicate error handling. Streaming introduces scenarios where errors may occur at any point in the stream. To effectively handle streaming errors, it’s important to:
-
Gracefully Handle Stream Closures – Always close the stream with a proper status code if an error occurs.
-
Handle Partial Failures – In some cases, streams can continue to function even after a partial failure. Log these failures and make sure they are communicated to the client to avoid confusion.
Conclusion
Mastering gRPC error handling is crucial for building robust, scalable microservices. By following best practices such as using appropriate status codes, providing detailed error messages, implementing retry logic, and monitoring for errors, you can ensure that your services are resilient and reliable.
If you’re looking to dive deeper into building efficient Go applications, check out The Ultimate Guide to Debugging with Go and Building Production-Ready Services with gRPC and Go. These resources will help you master not just gRPC, but also the broader skills needed to succeed in Go development.
Finally, stay up to date with the latest best practices for Go and gRPC development by subscribing to ByteSizeGo’s newsletter for concise, valuable technical insights delivered straight to your inbox.