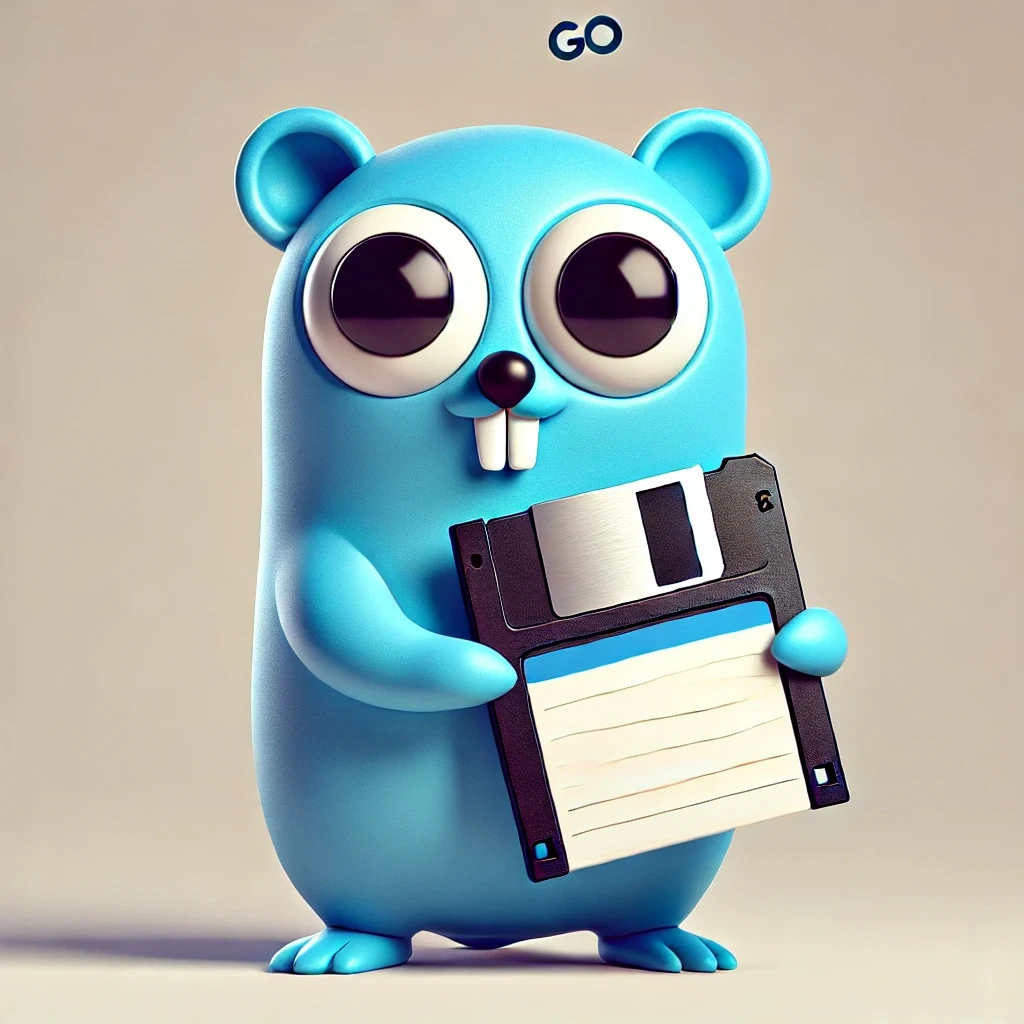
Reading Files Efficiently in Go
When working with file operations in Go, you should consider reading files line by line. This is especially useful when dealing with large files where loading the entire file into memory would be a bad idea. In this post, we will explore different ways to read a file (including line by line) in Golang, showcasing code examples and practical use cases.
Why Read a File Line by Line?
- Memory efficiency: Instead of loading the entire file into memory, we process each line individually.
- Stream processing: Great for real-time processing, such as log files.
- Simplicity: Sometimes, line-by-line processing is the simplest solution for parsing.
Here’s a few different ways to do it:
Method 1: Using bufio.Scanner
The bufio
package provides a convenient way to read files line by line. It’s one of the most commonly used methods because it’s simple and efficient.
package main
import (
"bufio"
"fmt"
"log"
"os"
)
func main() {
// Open the file
file, err := os.Open("example.txt")
if err != nil {
log.Fatalf("failed to open file: %s", err)
}
defer file.Close()
// Create a new scanner to read the file line by line
scanner := bufio.NewScanner(file)
// Loop through the file and read each line
for scanner.Scan() {
line := scanner.Text() // Get the line as a string
fmt.Println(line)
}
// Check for errors during the scan
if err := scanner.Err(); err != nil {
log.Fatalf("error reading file: %s", err)
}
}
Method 2: Using bufio.Reader with ReadString
Another way to read files line by line is to use bufio.Reader. This method gives you more control over how lines are read and allows you to specify the delimiter.
package main
import (
"bufio"
"fmt"
"log"
"os"
)
func main() {
// Open the file
file, err := os.Open("example.txt")
if err != nil {
log.Fatalf("failed to open file: %s", err)
}
defer file.Close()
// Create a new reader
reader := bufio.NewReader(file)
for {
// Read until we encounter a newline character
line, err := reader.ReadString('n')
if err != nil {
// If we encounter EOF, break out of the loop
if err.Error() == "EOF" {
break
}
log.Fatalf("error reading file: %s", err)
}
// Print the line
fmt.Print(line)
}
}
What if I want to read the entire file?
ioutil.ReadFile
can be used to read an entire file into memory and then split it into lines. This method is useful if you need to read small files and don’t mind holding the entire file in memory but is not recommended for big files.
package main
import (
"fmt"
"io/ioutil"
"log"
"strings"
)
func main() {
// Read the entire file into memory
data, err := ioutil.ReadFile("example.txt")
if err != nil {
log.Fatalf("failed to read file: %s", err)
}
// Split the file content into lines
lines := strings.Split(string(data), "n")
// Print each line
for _, line := range lines {
fmt.Println(line)
}
}