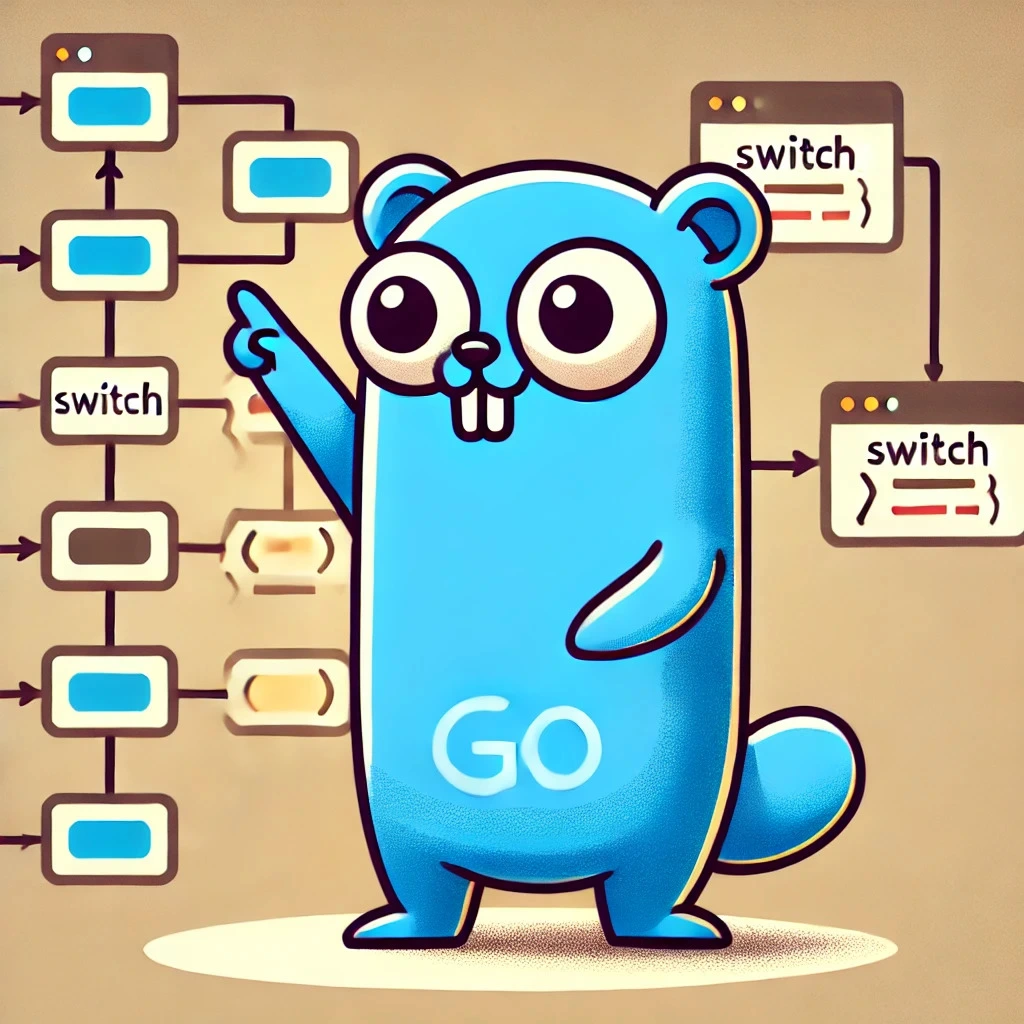
What is a switch Statement in Golang?
When programming in Go (or any language for that matter), decision-making is a core part of building applications. One of the most important tools for this is the switch statement. While most programmers are familiar with if statements, switch statements can provide a cleaner, more readable way to handle multiple conditions, especially when you have multiple clauses you want to consider. In this blog, we’ll explore how to effectively use the switch statement in Go, examining its syntax, use cases, and some advanced patterns.
What is a switch Statement in Golang?
If you have written any other modern programming language, you’ll find getting to grips with the switch statement in Golang very easy.
A switch statement in Golang evaluates an expression and executes the case that matches the expression’s result. Unlike in some other languages, where switch cases are strictly limited to integers, Golang allows cases to match on almost any type, including strings, booleans, or custom types. This makes it pretty versatile. Here’s an example:
switch expression {
case value1:
// Code to execute when expression == value1
case value2:
// Code to execute when expression == value2
default:
// Code to execute if no cases match
}
And here’s one that demonstrates how you might use it:
package main
import "fmt"
func main() {
day := 2
switch day {
case 1:
fmt.Println("Monday")
case 2:
fmt.Println("Tuesday")
case 3:
fmt.Println("Wednesday")
default:
fmt.Println("Invalid day")
}
}
In this example, when day equals 2, the program outputs “Tuesday”. The default case is executed when none of the specified cases match.
Expression-Less switch in Golang
This is where the switch statement in Go gets a little more interesting. Golang allows you to omit the expression altogether, as follows:
package main
import "fmt"
func main() {
score := 85
switch {
case score >= 90:
fmt.Println("A")
case score >= 80:
fmt.Println("B")
case score >= 70:
fmt.Println("C")
default:
fmt.Println("F")
}
}
In this case, instead of evaluating a single expression, the program checks conditions for each case block. You’ll see this pattern used quite a bit in Go; I use it fairly often for checking the type of errors with errors.Is.
Multiple Cases in a single Line!
You can also do this:
package main
import "fmt"
func main() {
day := "Saturday"
switch day {
case "Saturday", "Sunday":
fmt.Println("It's the weekend!")
default:
fmt.Println("It's a weekday.")
}
}
Here, both “Saturday” and “Sunday” cases trigger the same print statement. It’s the same as having separate cases, it’s just more concise.
Using the Fallthrough Keyword in a Golang switch
In some languages, each case in a switch statement automatically “falls through” to the next one unless explicitly terminated by a break. However, Go does not have implicit fallthrough. You must explicitly declare a fallthrough if you want one case to trigger the next. Make sure you read that a few times, it can be a common issue when just starting out with Go. Here’s an example:
package main
import "fmt"
func main() {
i := 2
switch i {
case 1:
fmt.Println("One")
case 2:
fmt.Println("Two")
fallthrough
case 3:
fmt.Println("Three")
default:
fmt.Println("Unknown")
}
}
In this example, when i is 2, both “Two” and “Three” will be printed due to the fallthrough statement. Use fallthrough sparingly, as it can reduce readability. You can run this example yourself here.
Type Switching in Go
You can also switch on the type of a thing, like this:
package main
import "fmt"
func typeCheck(i interface{}) {
switch v := i.(type) {
case int:
fmt.Println("Integer:", v)
case string:
fmt.Println("String:", v)
case bool:
fmt.Println("Boolean:", v)
default:
fmt.Println("Unknown type")
}
}
func main() {
typeCheck(42)
typeCheck("Golang")
typeCheck(true)
}
This is pretty cool and quite a popular thing to do - especially in code pre-generics.
Best Practices for Using switch in Golang
-
Keep Cases Focused: When using a switch, ensure that each case is specific and straightforward. Long, complex logic inside each case can lead to readability issues.
-
Use Expression-less Switches When Appropriate: If you are checking multiple conditions, consider using an expression-less switch to simplify your code.
-
Avoid Overusing fallthrough: The fallthrough keyword can make your code harder to follow. Use it only when there’s a clear and justifiable reason.
-
Leverage Type Switches for Dynamic Typing: If you work with interfaces, type switches are essential for handling different data types cleanly and efficiently.
Wrapping up on Switch Statements in Go
You now know everything there is to know about switch statements in Go! If you found this useful, consider signing up to our newsletter below and checking out some of our other great Go content. Here’s some we recommend: