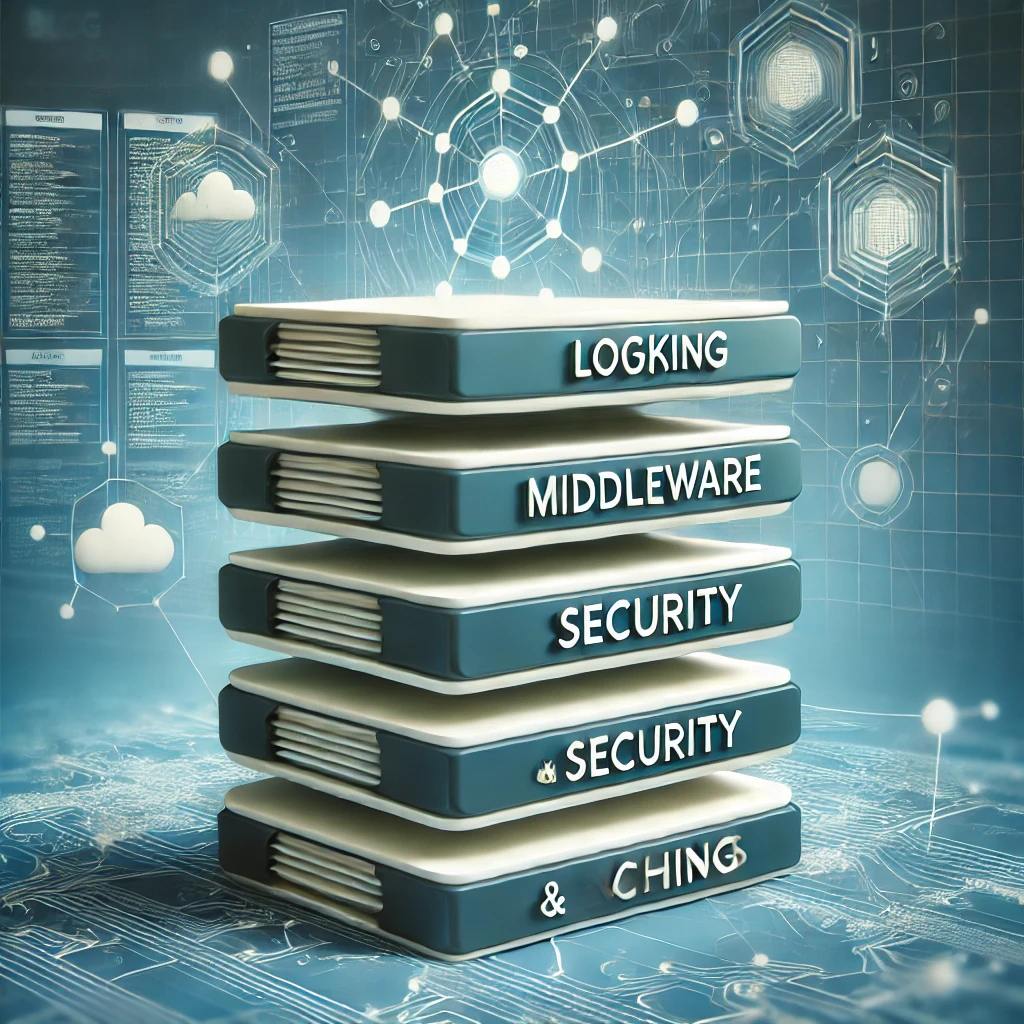
Understanding gRPC Middleware: Enhance Your gRPC Applications
Creating efficient and scalable applications is more important than ever. gRPC is a robust framework that helps developers build high-performance APIs, especially in microservices. One of the standout features that can elevate your gRPC applications is middleware. So, what’s the deal with middleware? Let’s unpack it!
Before we jump in, you might find these free courses super helpful:
What is gRPC Middleware?
Definition and Purpose
Think of gRPC middleware as a helpful middleman between your application logic and the network transport layer. It intercepts requests and responses, letting you add features like logging, authentication, and error handling without cluttering your main code. It’s all about keeping your code clean and your functionality intact.
How Middleware Works in gRPC
In the world of gRPC, middleware can come in two flavors: unary interceptors for single requests and stream interceptors for streaming calls. Middleware functions take in a handler and return a new one, allowing you to add extra logic before and after your core application logic.
The Flow of Middleware Execution
Here’s a quick rundown of how it works:
- Request Interception: Middleware catches incoming requests, allowing you to add logic for things like validation or logging.
- Next Handler Call: The middleware calls the next handler in the chain, which holds your business logic.
- Response Interception: Once the request is processed, middleware can log or modify the response before it heads back to the client.
Benefits of Using gRPC Middleware
Why should you consider using middleware in your gRPC applications? Here are a few compelling reasons:
- Code Reusability: By wrapping common functionalities into middleware, you can reuse that code across different services, keeping your code DRY (Don’t Repeat Yourself) and maintainable.
- Separation of Concerns: Middleware promotes a clean separation of concerns, allowing your business logic to focus on its core responsibilities.
- Improved Performance and Scalability: Middleware can enhance performance through caching or rate limiting, improving response times for frequently accessed data.
- Enhanced Security: Middleware can handle authentication and authorization consistently across your application.
- Centralized Error Handling: Middleware allows for uniform error responses, simplifying client-side error handling and improving user experience.
Implementing gRPC Middleware
Step-by-Step Example
Let’s walk through creating a simple logging middleware in a gRPC application using Go.
-
Set Up Your gRPC Service
First, ensure you have a basic gRPC service up and running. Here’s a detailed setup guide. -
Define the Middleware
Here’s a simple logging middleware that logs incoming requests:package main import ( "context" "log" "google.golang.org/grpc" ) func loggingMiddleware(next grpc.Handler) grpc.Handler { return func(ctx context.Context, req interface{}) (interface{}, error) { log.Println("Received request:", req) resp, err := next(ctx, req) log.Println("Response:", resp) return resp, err } }
-
Register the Middleware
func main() {
server := grpc.NewServer(
grpc.UnaryInterceptor(loggingMiddleware),
)
// Register your gRPC service here
pb.RegisterYourServiceServer(server, &yourService{})
log.Println("Starting server...")
if err := server.Serve(lis); err != nil {
log.Fatalf("Failed to serve: %v", err)
}
}
- Testing the Middleware Run your gRPC server and send some requests using a gRPC client. You should see logs for each incoming request and response.
Additional Middleware Examples
While logging is a great start, there are plenty of other middleware types you can explore:
1. Authentication Middleware
This one ensures that only authorized users can access your services. You might validate JWT tokens or API keys. Here’s a quick example:
func authMiddleware(next grpc.Handler) grpc.Handler {
return func(ctx context.Context, req interface{}) (interface{}, error) {
token, err := extractToken(ctx)
if err != nil || !isValidToken(token) {
return nil, grpc.Errorf(codes.Unauthenticated, "Invalid token")
}
return next(ctx, req)
}
}
2. Rate Limiting Middleware
Prevent abuse of your services by implementing rate limiting middleware. It can track requests per client and enforce limits, keeping your service responsive.
3. Monitoring Middleware
Keeping an eye on performance is essential. Middleware can gather metrics on request times, error rates, and other important data, which you can then integrate with tools like Prometheus or Grafana.
func monitoringMiddleware(next grpc.Handler) grpc.Handler {
return func(ctx context.Context, req interface{}) (interface{}, error) {
start := time.Now()
resp, err := next(ctx, req)
duration := time.Since(start)
log.Printf("Request processed in %s", duration)
return resp, err
}
}
4. Caching Middleware
Want to speed things up? Implement caching middleware to store results from expensive operations or frequently accessed data, which can help reduce server load and response times.
Best Practices for Using gRPC Middleware
To get the most out of your gRPC middleware, keep these best practices in mind:
1. Keep Middleware Lightweight
Make sure your middleware functions are lightweight. Avoid heavy computations or blocking calls to maintain application responsiveness.
2. Order Matters
The order in which you register middleware can make a difference. For instance, authentication middleware should come before logging middleware to ensure only authorized requests get logged.
3. Be Mindful of State
Try to keep your middleware stateless to avoid unexpected behavior in distributed environments. If you need to maintain state, consider using an external store.
4. Document Your Middleware
Clear documentation of your middleware functions is key. Outline their purpose, inputs, and outputs. This will help future developers (and your future self!) understand what’s going on.
Conclusion
gRPC middleware is an incredibly powerful tool that can enhance your applications by adding reusable, maintainable, and secure functionality. By leveraging middleware, you can refine your application’s architecture and tackle common challenges in modern software development.
As you work on your gRPC applications, think about how middleware can help you keep your code clean, boost performance, and improve security. For more insights, don’t miss the Ultimate Guide to Debugging with Go for effective debugging strategies, and check out our guide to building production-ready gRPC services from scratch with Go