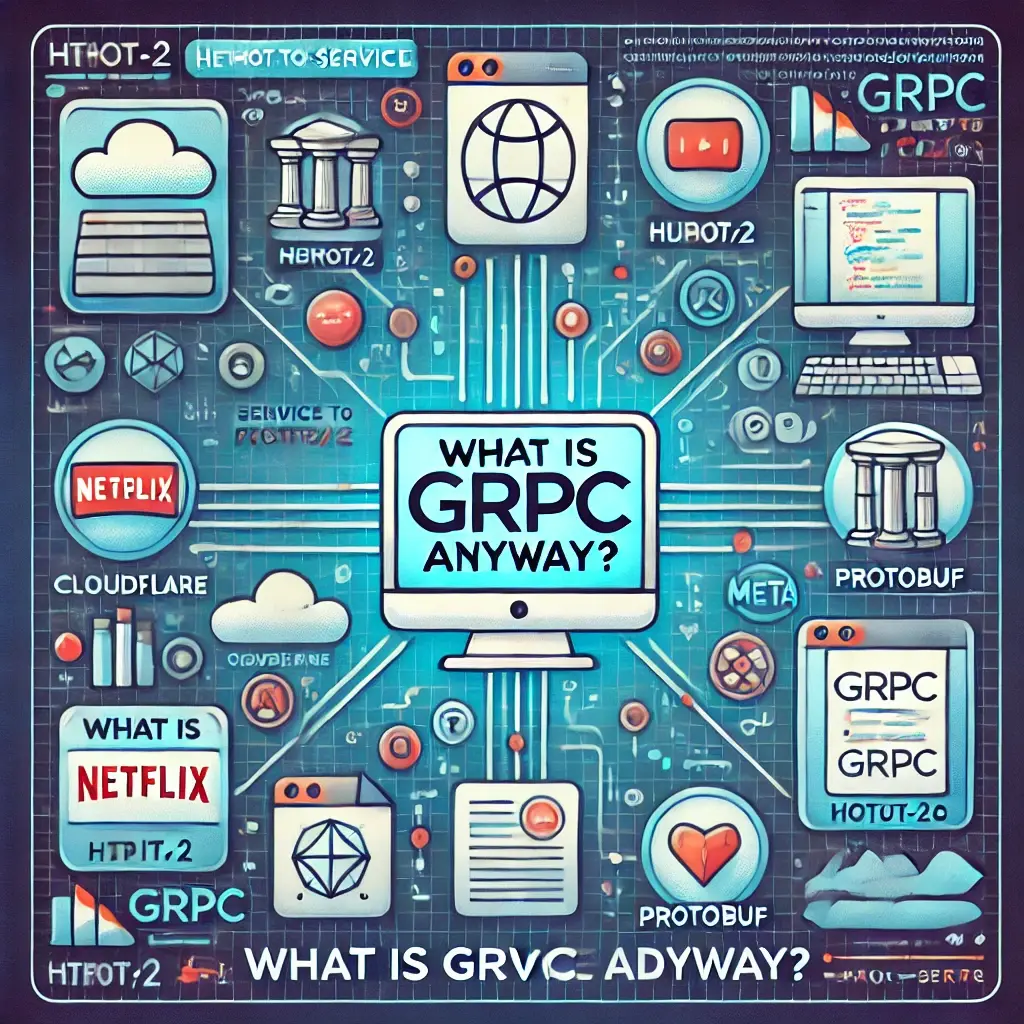
What is gRPC Anyway?
Chances are you have heard people mention gRPC, but what really is it and what’s it useful for? gRPC particularly excels at service-to-service communication and does so very efficiently with a strong schema. By using schema-first design, gRPC can be language agnostic and support many programming languages (including Go) with very low effort from the maintainer.
It does this by generating code based on a Protobuf.
Where did gRPC Come From?
Here’s a brief history of gRPC.
gRPC was developed by Google and released in 2015. It was created to improve communication between different services in distributed systems, especially for Google’s own needs as they had requirements around speed.
gRPC stands for “gRPC Remote Procedure Calls,” and it builds on the principles of an earlier technology called “Stubby” that Google used internally since 2001.
By making gRPC open source, Google made it available to everyone, and it has been widely adopted since. Big companies such as Netflix, Cloudflare, and Meta all use gRPC in some capacity.
How does gRPC work under the hood?
gRPC works by using HTTP/2 and Protocol Buffers (Protobuf) to make communication between services fast and efficient.
Under the hood, gRPC allows a client application to directly call a method on a server application as if it were a local function, even though they may be on different machines. It uses HTTP/2 for transport, which enables features like multiplexing and full-duplex communication, and Protobuf to serialize structured data into a binary format. This makes gRPC suitable for high-performance and low-latency scenarios in distributed systems.
Here’s a minimal Go Client example. The interesting line is client.SayHello
which was generated for us and looks like a local call.
package main
import (
"context"
"log"
"time"
"google.golang.org/grpc"
)
// Import the generated protobuf code
// import pb "path/to/your/proto/package"
func main() {
// Connect to the server at localhost:50051
conn, err := grpc.Dial("localhost:50051", grpc.WithInsecure())
if err != nil {
log.Fatalf("did not connect: %v", err)
}
defer conn.Close()
// Create a new client from the generated protobuf code
client := NewGreeterClient(conn)
// Call the SayHello method
ctx, cancel := context.WithTimeout(context.Background(), time.Second)
defer cancel()
// Send a request to the server
response, err := client.SayHello(ctx, &HelloRequest{Name: "World"})
if err != nil {
log.Fatalf("could not greet: %v", err)
}
// Print the response from the server
log.Printf("Greeting: %s", response.Message)
}
What is a Protobuf?
Protocol Buffers (Protobuf) is a method developed by Google for serializing structured data.
Protobufs are like blueprint designs for your data that tell gRPC how to encode (or serialize) and decode (or deserialize) messages so they can be sent over the network efficiently. This makes sending data faster and more compact compared to text-based formats like JSON or (god forbid) XML.
Do I have to use Protobuf or can I use Something else?
While gRPC primarily uses Protocol Buffers (Protobuf) for data serialization, it is not strictly limited to Protobuf. gRPC can theoretically work with other serialization formats. However, Protobuf is the default and most commonly used because it is efficient and optimized for gRPC’s use case. Using another format might require more custom configuration and could reduce some of the performance benefits of gRPC. All of the gRPC tooling assumes Protobuf, so we recommend sticking with it.
What are the Different Methods I can use in gRPC?
In gRPC, you can use four different types of methods to communicate between a client and a server:
- Unary RPC: The client sends a single request, and the server returns a single response.
- Server Streaming RPC: The client sends a single request, and the server returns a stream of responses.
- Client Streaming RPC: The client sends a stream of requests, and the server returns a single response.
- Bidirectional Streaming RPC: Both the client and server send streams of requests and responses simultaneously.
What are the Benefits of gRPC?
The benefits of gRPC include:
- High Performance: gRPC uses HTTP/2 and Protobuf, which makes communication fast and efficient.
- Strongly Typed Contracts: Ensures reliable communication by defining strict interfaces for services.
- Streaming Support: Allows both clients and servers to send multiple messages in a single connection, which is useful for real-time data.
- Language Agnostic: Supports many programming languages, making it versatile for different environments.
- Automatic Code Generation: Simplifies development by generating client and server code from service definitions.
What are the Downsides of gRPC?
The downsides of gRPC include:
- Limited Browser Support: gRPC is not natively supported in web browsers, making it less ideal for web-based applications.
- Complex Setup: Setting up gRPC can be more complex compared to simpler protocols like REST.
- Learning Curve: Understanding gRPC and Protocol Buffers may require additional learning, especially for developers unfamiliar with these technologies.
- Lack of Human-Readable Messages: Protobuf messages are not human-readable, making debugging more difficult without proper tools.
When Should I use gRPC?
You should use gRPC when you need high-performance communication between services, especially in distributed systems. It’s ideal for situations requiring low latency and high throughput, such as real-time services, microservices architectures, and mobile or IoT devices. gRPC is also a good choice when you need strong data typing and automatic code generation for multiple programming languages.
Does Go Work well with gRPC?
Yes, Go works very well with gRPC. The Go language has strong support for gRPC, with libraries and tools that make it easy to implement gRPC services and clients. The performance benefits of gRPC, such as low latency and efficient data serialization with Protocol Buffers, align well with Go’s philosophy, and therefore is a match made in heaven.
How can I learn more about gRPC?
We have a course which assumes no gRPC knowledge and will teach you everything you need to know here.