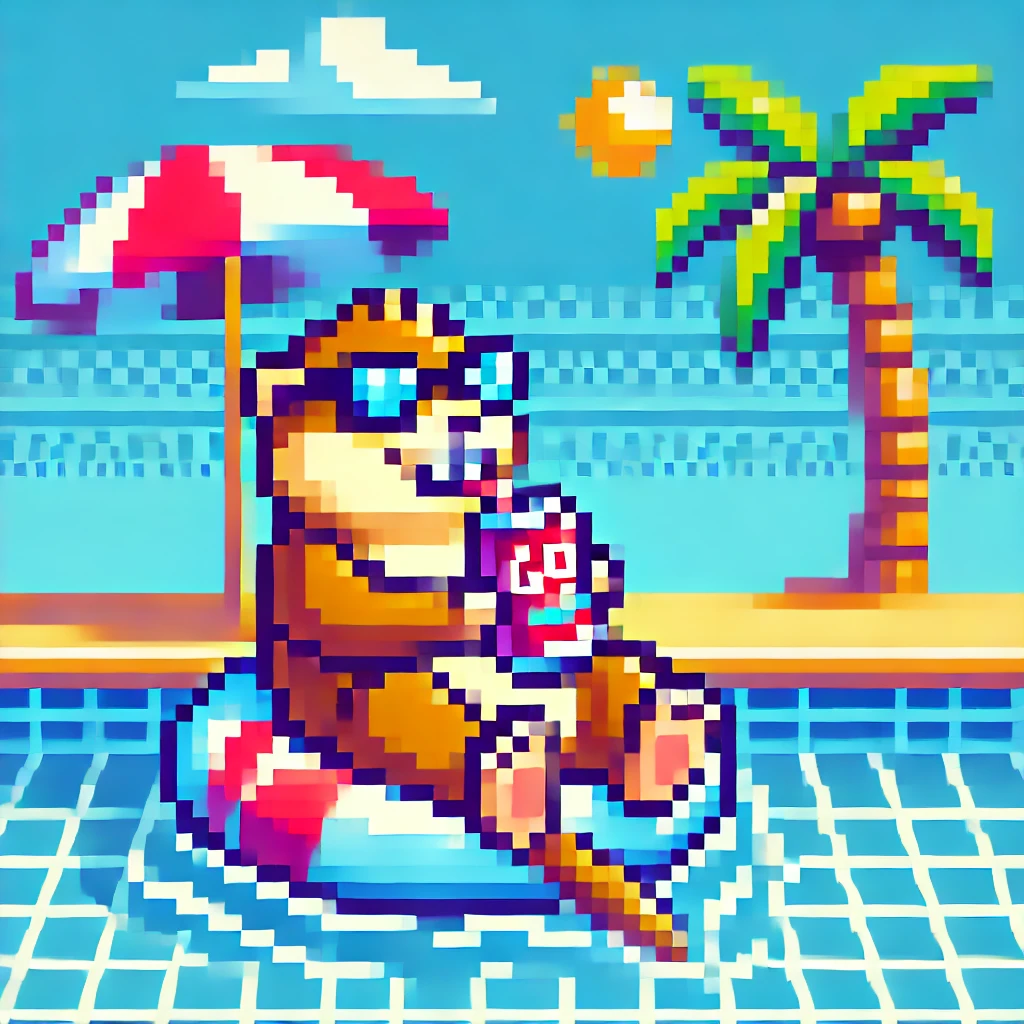
omitzero in Go1.24: tips and gotchas!
Go 1.24 has a cool new feature called omitzero
. It helps you clean up your JSON output by skipping fields that have
default values. This is in addition to the emitempty
tag that you can still use.
What Does omitzero
Do?
In Go, you use struct tags to decide what data gets included in your JSON. Before, we used omitempty
to skip fields
that were empty, like:
- Numbers that are
0
. - Strings that are
""
(empty). - Pointers or slices that are
nil
(nothing).
Now, with omitzero
, you can specifically skip fields that have a zero value (like 0
for numbers or an empty
time).
You can also add custom definitions of empty. We’ll see that shortly.
Example of omitzero
Here’s what it looks like:
type Example struct {
Name string `json:"name,omitzero"`
Age int `json:"age,omitzero"`
Balance float64 `json:"balance,omitzero"`
}
If Name
is ""
(empty), Age
is 0
, and Balance
is 0.0
, these fields won’t appear in your JSON output.
How is omitzero
Different From omitempty
?
Here’s a comparison:
Tag | What It Does |
---|---|
omitempty | Skips fields that are empty. |
omitzero | Skips fields with zero values. |
Why Use omitzero
?
-
Cleaner JSON: Your JSON outputs are smaller and easier to read.
-
Precise Control: You can focus on skipping zero values only.
-
Better for APIs: Helps you build clearer APIs that don’t include unnecessary fields.
Special Cases
Go 1.24 fixed an old problem with omitempty
. It didn’t handle time.Time
well when it was zero. But with omitzero
,
zero-value times are properly skipped!
Also, you can use both omitzero
and omitempty
if you want to skip fields for either reason.
type Event struct {
Name string `json:"name,omitempty,omitzero"`
Timestamp time.Time `json:"timestamp,omitzero"`
}
Here:
Name
gets skipped if it’s empty or zero.Timestamp
gets skipped if it’s zero.
The isZero
Method
The omitzero
feature relies on a special method in Go called isZero
. This method is used by the encoding system to
determine whether a value is the zero value for its type. If a struct field implements the isZero
method, it overrides
Go’s default behavior and allows developers to define what counts as a “zero value.”
Here’s an example of how to use isZero
:
package main
import (
"encoding/json"
"fmt"
)
type CustomField struct {
Value int
}
func (cf CustomField) isZero() bool {
return cf.Value <= 0 // Define zero as any non-positive value
}
type Example struct {
Custom CustomField `json:"custom,omitzero"`
}
func main() {
data := Example{
Custom: CustomField{Value: 0},
}
result, _ := json.Marshal(data)
fmt.Println(string(result)) // Output: {}
}
In this example, the CustomField
type defines its own logic for determining zero values. This gives developers even
more control when using omitzero
.
When Should You Use omitzero
?
Use omitzero
when you want to skip only zero values. If you need to skip empty values as well, stick with omitempty
or combine both.
Conclusion
omitzero
is an awesome feature in Go 1.24 that helps make your JSON cleaner and more meaningful. Give it a try if
you’re working with APIs or want to simplify your data.
Read the full release notes here. For more tips, check out ByteSizeGo blogs.
Happy coding!